课程 使用 API 和 Web 抓取实现 HR 自动化
欢迎回到我们的Python从0到英雄系列!到目前为止,我们已经学习了如何操作数据并使用强大的外部库来执行与工资和人力资源系统相关的任务。但是,如果您需要获取实时数据或与外部服务交互怎么办?这就是 API 和 网页抓取 发挥作用的地方。
在本课中,我们将介绍:
- API是什么以及它们为何有用。
- 如何使用 Python 的 requests 库与 REST API 交互。
- 如何应用网络抓取技术从网站提取数据。
- 实际示例,例如获取工资的实时税率或从网站抓取员工福利数据。
在本课程结束时,您将能够自动化外部数据检索,使您的 HR 系统更加动态和数据驱动。
1.什么是API?
API(应用程序编程接口)是一组允许不同软件应用程序相互通信的规则。简而言之,它允许您直接从代码与另一个服务或数据库交互。
例如:
- 您可以使用 API 获取实时税率用于工资计算。
- 您可以与人力资源软件 API 集成,将员工数据直接提取到您的系统中。
- 或者您可以使用天气 API 来了解何时根据极端天气条件为员工提供特殊福利。
大多数 API 使用名为 REST(表述性状态传输)的标准,它允许您发送 HTTP 请求(如 GET 或 POST)来访问或更新数据。
2. 使用Requests库与API交互
Python 的 requests 库可以轻松使用 API。您可以通过运行来安装它:
pip install requests
发出基本 API 请求
让我们从一个简单的示例开始,了解如何使用 GET 请求从 API 获取数据。
import requests # Example API to get public data url = "https://jsonplaceholder.typicode.com/users" response = requests.get(url) # Check if the request was successful (status code 200) if response.status_code == 200: data = response.json() # Parse the response as JSON print(data) else: print(f"Failed to retrieve data. Status code: {response.status_code}")
在此示例中:
- 我们使用 requests.get() 函数从 API 获取数据。
- 如果请求成功,数据会被解析为JSON,我们就可以处理它了。
HR应用示例:获取实时税务数据
假设您想要获取实时税率以用于工资核算。许多国家提供了税率的公共 API。
在此示例中,我们将模拟从税务 API 获取数据。使用实际 API 时的逻辑是类似的。
import requests # Simulated API for tax rates api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] print(f"Federal Tax Rate: {federal_tax}%") print(f"State Tax Rate: {state_tax}%") # Use the tax rates to calculate total tax for an employee's salary salary = 5000 total_tax = salary * (federal_tax + state_tax) / 100 print(f"Total tax for a salary of ${salary}: ${total_tax:.2f}") else: print(f"Failed to retrieve tax rates. Status code: {response.status_code}")
此脚本可以修改为与实际税率 API 配合使用,帮助您使工资系统保持最新的税率。
3. 网页抓取来收集数据
虽然 API 是获取数据的首选方法,但并非所有网站都提供它们。在这些情况下,网络抓取可用于从网页中提取数据。
Python 的 BeautifulSoup 库以及请求使网络抓取变得简单。您可以通过运行来安装它:
pip install beautifulsoup4
示例:从网站上抓取员工福利数据
想象一下您想要从公司的人力资源网站上抓取有关员工福利的数据。这是一个基本示例:
import requests from bs4 import BeautifulSoup # URL of the webpage you want to scrape url = "https://example.com/employee-benefits" response = requests.get(url) # Parse the page content with BeautifulSoup soup = BeautifulSoup(response.content, 'html.parser') # Find and extract the data you need (e.g., benefits list) benefits = soup.find_all("div", class_="benefit-item") # Loop through and print out the benefits for benefit in benefits: title = benefit.find("h3").get_text() description = benefit.find("p").get_text() print(f"Benefit: {title}") print(f"Description: {description}\n")
在此示例中:
- 我们使用requests.get()请求网页的内容。
- BeautifulSoup 对象解析 HTML 内容。
- 然后,我们使用 find_all() 提取我们感兴趣的特定元素(例如,福利标题和描述)。
此技术对于从网络收集与人力资源相关的数据(例如福利、职位发布或薪资基准)非常有用。
4. 在 HR 应用程序中结合 API 和 Web 抓取
让我们将所有内容放在一起,创建一个结合 API 使用和 Web 抓取的迷你应用程序,用于真实的 HR 场景:计算员工的总成本。
我们会:
- Use an API to get real-time tax rates.
- Scrape a webpage for additional employee benefit costs.
Example: Total Employee Cost Calculator
import requests from bs4 import BeautifulSoup # Step 1: Get tax rates from API def get_tax_rates(): api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] return federal_tax, state_tax else: print("Error fetching tax rates.") return None, None # Step 2: Scrape employee benefit costs from a website def get_benefit_costs(): url = "https://example.com/employee-benefits" response = requests.get(url) if response.status_code == 200: soup = BeautifulSoup(response.content, 'html.parser') # Let's assume the page lists the monthly benefit cost benefit_costs = soup.find("div", class_="benefit-total").get_text() return float(benefit_costs.strip("$")) else: print("Error fetching benefit costs.") return 0.0 # Step 3: Calculate total employee cost def calculate_total_employee_cost(salary): federal_tax, state_tax = get_tax_rates() benefits_cost = get_benefit_costs() if federal_tax is not None and state_tax is not None: # Total tax deduction total_tax = salary * (federal_tax + state_tax) / 100 # Total cost = salary + benefits + tax total_cost = salary + benefits_cost + total_tax return total_cost else: return None # Example usage employee_salary = 5000 total_cost = calculate_total_employee_cost(employee_salary) if total_cost: print(f"Total cost for the employee: ${total_cost:.2f}") else: print("Could not calculate employee cost.")
How It Works:
- The get_tax_rates() function retrieves tax rates from an API.
- The get_benefit_costs() function scrapes a webpage for the employee benefits cost.
- The calculate_total_employee_cost() function calculates the total cost by combining salary, taxes, and benefits.
This is a simplified example but demonstrates how you can combine data from different sources (APIs and web scraping) to create more dynamic and useful HR applications.
Best Practices for Web Scraping
While web scraping is powerful, there are some important best practices to follow:
- Respect the website’s robots.txt: Some websites don’t allow scraping, and you should check their robots.txt file before scraping.
- Use appropriate intervals between requests: Avoid overloading the server by adding delays between requests using the time.sleep() function.
- Avoid scraping sensitive or copyrighted data: Always make sure you’re not violating any legal or ethical rules when scraping data.
Conclusion
In this lesson, we explored how to interact with external services using APIs and how to extract data from websites through web scraping. These techniques open up endless possibilities for integrating external data into your Python applications, especially in an HR context.
以上是课程 使用 API 和 Web 抓取实现 HR 自动化的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
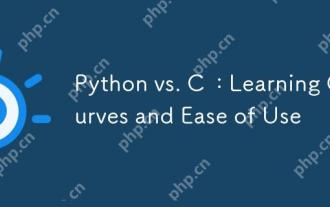
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
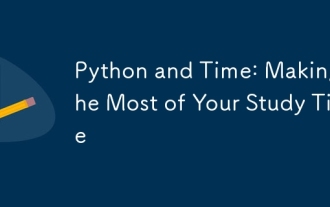
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
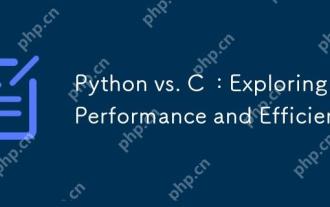
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
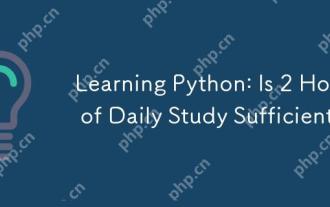
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
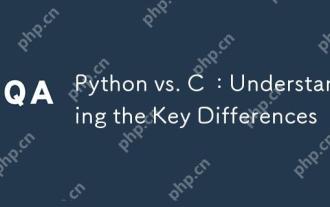
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
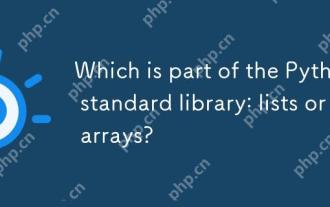
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
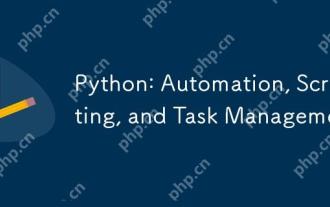
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
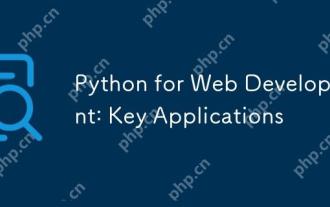
Python在Web开发中的关键应用包括使用Django和Flask框架、API开发、数据分析与可视化、机器学习与AI、以及性能优化。1.Django和Flask框架:Django适合快速开发复杂应用,Flask适用于小型或高度自定义项目。2.API开发:使用Flask或DjangoRESTFramework构建RESTfulAPI。3.数据分析与可视化:利用Python处理数据并通过Web界面展示。4.机器学习与AI:Python用于构建智能Web应用。5.性能优化:通过异步编程、缓存和代码优
