React Toastify 入门:增强您的通知
介绍
在现代 Web 应用程序中,向用户提供实时反馈对于保持流畅且引人入胜的体验至关重要。通知在传达重要事件(例如成功操作、错误或警告)而不中断用户工作流程方面发挥着关键作用。这就是 React Toastify 发挥作用的地方。它是一个流行的库,可以简化向 React 应用程序添加可自定义 toast 通知的过程。与会打断用户旅程的传统警报框不同,Toast 通知以微妙而优雅的方式出现,确保在不让用户脱离当前上下文的情况下传达重要信息。
借助 Toastify,开发人员可以轻松实现美观且高度灵活的通知,允许自定义位置、样式和时间,同时易于设置和使用。这使其成为希望通过有效的反馈机制增强用户体验的开发人员不可或缺的工具。
为什么使用 React Toastify?
Toast 通知在 Web 应用程序的许多常见场景中至关重要。例如,在用户提交表单后,您可能希望显示一条成功消息以确认操作已完成,或者在出现问题时显示一条错误消息。同样,在处理 API 调用时,Toast 通知可以通知用户结果,例如数据检索成功或错误。
React-Toastify 可以无缝且高效地处理这些通知。以下是它与默认浏览器警报和其他库的一些主要优点:
- 易于集成:设置简单,只需最少的配置即可开始显示通知。其直观的 API 甚至适合初学者,让开发人员无需复杂的设置即可快速添加 Toast 通知。
- 可定制的设计和定位: Toastify 的突出功能之一是它能够自定义通知的外观和行为。您可以轻松修改样式,将它们放置在屏幕上的任何位置(例如,右上角、左下角),甚至创建自定义过渡。这种灵活性有助于在您的应用程序中保持一致的 UI/UX。
支持自动和手动关闭:Toastify 让您可以控制通知保持可见的时间。您可以选择在指定时间后自动关闭或允许用户手动关闭通知,从而根据上下文提供更好的用户体验。
与默认浏览器警报的比较:默认浏览器警报具有侵入性,会阻止用户交互,直到被关闭。另一方面,Toastify 提供非侵入式、优雅的 toast,出现在屏幕一角,并允许用户继续与页面交互。它还支持更高级的功能,例如不同的 toast 类型(成功、错误、信息)和更丰富的样式,这是浏览器警报无法实现的。
通过将 React-Toastify 集成到您的 React 应用程序中,您可以获得一种强大且可自定义的方式来管理通知,从而更轻松地向用户提供反馈,同时保持流畅、现代的用户体验。
安装和设置
React-Toastify 入门非常简单,只需几个步骤。以下是您在 React 项目中安装和设置它的方法:
第 1 步:安装 React Toastify
首先,您需要将 React-Toastify 包添加到您的项目中。在终端中使用以下命令:
npm install react-toastify
第 2 步:在项目中导入并使用 React Toastify
安装包后,您需要将 React Toastify 及其核心组件导入到您的 React 项目中。至少,您应该导入 ToastContainer,它负责在屏幕上呈现 toast 通知。
设置方法如下:
- 将 ToastContainer 和 toast 导入到您的组件中。
- 确保 ToastContainer 包含在组件的 JSX 中。
- 使用 toast 函数触发 toast 通知。
示例:
import React from 'react'; import { ToastContainer, toast } from 'react-toastify'; import 'react-toastify/dist/ReactToastify.css'; const App = () => { const notify = () => toast("This is a toast notification!"); return ( <div> <h1>React Toastify Example</h1> <button onClick={notify}>Show Notification</button> <ToastContainer /> </div> ); }; export default App;
第三步:添加吐司样式
不要忘记导入 React Toastify CSS 文件以应用通知的默认样式:
import 'react-toastify/dist/ReactToastify.css';
Now, when you click the button, a toast notification will appear on the screen. The ToastContainer can be positioned anywhere in your app, and the toasts will automatically appear within it. You can further customize the appearance and behavior of the toast, which we will explore in the following sections.
Basic Usage of React Toastify
Once you have React Toastify set up, you can easily trigger various types of notifications based on user actions. Let's explore how to use it to display different toast notifications for success, error, info, and warning messages.
Example 1: Triggering a Success Notification
A common use case for a success notification is after a form submission. You can trigger it using the following code:
toast.success("Form submitted successfully!");
This will display a success message styled with a green icon, indicating a positive action.
Example 2: Error Notification
You can also display an error message when something goes wrong, such as a failed API call or form validation error:
toast.error("Something went wrong. Please try again!");
This shows a red-colored toast indicating an issue that requires the user's attention.
Example 3: Info Notification
Info notifications are useful when you want to inform the user about a status or update without implying success or failure. For example:
toast.info("New updates are available!");
Example 4: Warning Notification
If you need to alert the user to potential issues or important warnings, you can use the warning notification:
toast.warn("Your session is about to expire!");
This shows an orange toast, typically used for warnings or cautions.
import React from 'react'; import { ToastContainer, toast } from 'react-toastify'; import 'react-toastify/dist/ReactToastify.css'; const App = () => { const showToasts = () => { toast.success("Form submitted successfully!"); toast.error("Something went wrong. Please try again!"); toast.info("New updates are available!"); toast.warn("Your session is about to expire!"); }; return (); }; export default App;React Toastify Notifications
With this code, clicking the button will trigger all types of notifications, allowing you to see how each one looks and behaves in your application.
Customizing Toast Notifications
One of the great features of React Toastify is its flexibility in customizing toast notifications to fit the look and feel of your application. You can easily adjust the position, duration, and even styling to suit your needs. Let’s walk through some of these customizations.
Customizing Position
React Toastify allows you to position toast notifications in various locations on the screen. By default, toasts are displayed in the top-right corner, but you can customize their position using the position property of the ToastContainer or while triggering individual toasts.
Available positions:
- top-right (default)
- top-center
- top-left
- bottom-right
- bottom-center
- bottom-left
Here’s an example of how to change the position of toasts globally via the ToastContainer:
<ToastContainer position="bottom-left" />
If you want to customize the position of individual toasts, you can pass the position option like this:
toast.success("Operation successful!", { position: "top-center" });
This will display the success notification at the top-center of the screen.
Adjusting the Auto-Dismiss Timer
toast.info("This will disappear in 3 seconds!", { autoClose: 3000 });
If you want the toast to stay on screen until the user manually dismisses it, set autoClose to false:
toast.warn("This requires manual dismissal.", { autoClose: false });
Customizing Toast Styling
React Toastify provides the flexibility to style your toasts either through CSS classes or inline styles. You can pass a custom CSS class to the className or bodyClassName options to style the overall toast or its content.
Here’s an example of using a custom CSS class to style a toast:
toast("Custom styled toast!", { className: "custom-toast", bodyClassName: "custom-toast-body", autoClose: 5000 });
In your CSS file, you can define the styling:
.custom-toast { background-color: #333; color: #fff; } .custom-toast-body { font-size: 18px; }
This gives you complete control over how your notifications appear, allowing you to match the toast design with your application’s overall theme.
Advanced Features of React Toastify
React Toastify offers useful features to enhance the functionality of your toast notifications. Here's how you can leverage progress bars, custom icons, transitions, and event listeners.
Progress Bars in Toast Notifications
By default, React Toastify includes a progress bar that indicates how long the toast will stay visible. To disable the progress bar:
toast.info("No progress bar", { hideProgressBar: true });
Custom Icons and Transitions
You can replace default icons with custom icons for a more personalized look:
toast("Custom Icon", { icon: "?" });
To apply custom transitions like Bounce:
toast("Bounce Animation", { transition: Bounce });
Adding Event Listeners to Toasts
React Toastify allows you to add event listeners to handle custom actions, such as on click:
toast.info("Click me!", { onClick: () => alert("Toast clicked!") });
You can also trigger actions when a toast is closed:
toast.success("Success!", { onClose: () => console.log("Toast closed") });
Best Practices for Using React Toastify
To ensure that toast notifications enhance rather than hinder the user experience, it's important to follow best practices. Here are some guidelines to consider:
-
Use Notifications Sparingly
While notifications can be helpful, overusing them can frustrate or distract users. Reserve toast notifications for important updates, such as confirming successful actions (e.g., form submissions) or displaying error messages that require attention.
-
Choose the Right Notification Type
Use appropriate toast types (success, error, info, warning) to convey the correct tone. For instance, success messages should be used for completed actions, while warnings should be reserved for potential issues.
-
Avoid Blocking User Actions
Since toasts are non-intrusive, they should not block important user interactions. Display notifications in a way that doesn’t prevent users from continuing their tasks.
-
Customize Timing Based on Context
Set reasonable auto-dismiss times for toasts. Error messages might need to stay longer, while success notifications can disappear quickly. For critical issues, consider letting users manually dismiss the notification.
Conclusion
React-Toastify makes implementing notifications in React applications seamless and efficient, offering a flexible solution for delivering real-time feedback to users. With its customizable design, positioning options, and support for advanced features like progress bars and event listeners, it simplifies the notification process while allowing for great control over the user experience.
By following best practices and using toast notifications wisely, you can enhance user interaction without overwhelming them. For more detailed information and advanced use cases, be sure to check out the official React Toastify documentation.
以上是React Toastify 入门:增强您的通知的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
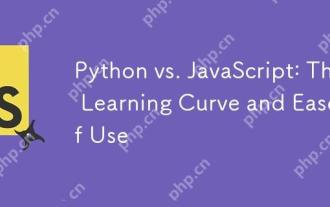
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
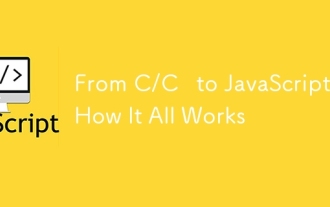
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
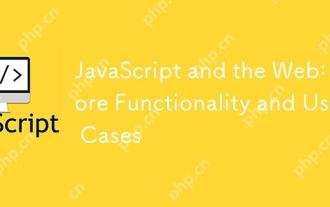
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
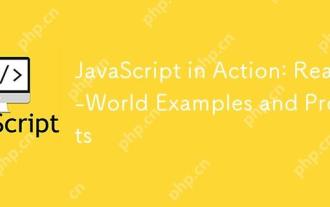
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
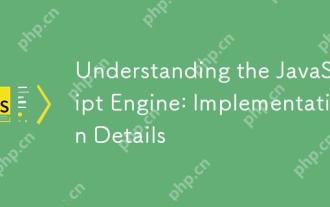
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
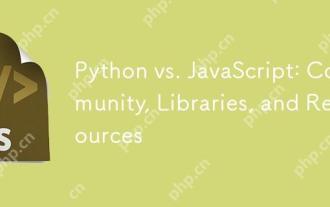
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
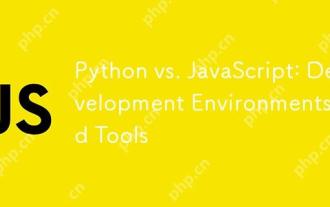
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
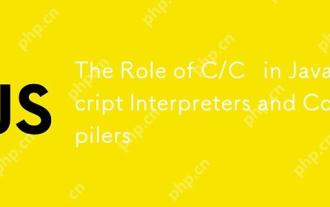
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
