使用 Linq、Criteria API 和 Query Over 扩展 NHibernate 的 Ardalis.Specification
Ardalis.Specification 是一个功能强大的库,支持查询数据库的规范模式,主要为 Entity Framework Core 设计,但在这里我将演示如何扩展 Ardalis.Specification 来使用NHibernate 也是一种 ORM。
这篇博文假设您对 Ardalis.Specification 有一定的经验,并且希望在使用 NHibernate 的项目中使用它。如果您还不熟悉 Ardalis.Specification,请参阅文档以了解更多信息。
首先,NHibernate 中有三种不同的内置方法来执行查询
- Linq 查询(使用 IQueryable)
- 标准 API
- 查询结束
我将介绍如何扩展 Ardalis.Specification 来处理所有 3 种方式,但由于 Linq to Query 也可以像 Entity Framework Core 一样与 IQueryable 配合使用,因此我将首先介绍该选项。
Linq 查询
在创建连接关系时,Entity Framework Core 和 NHIbernate 之间存在细微差别。在 Entity Framework Core 中,我们在 IQueryable 上有扩展方法: Include 和 ThenInclude (这些也是 Ardalis.Specification 中使用的方法名称)。
Fetch、FetchMany、ThenFetch 和 ThenFetchMany 是 IQueryable 上执行连接的 NHibernate 特定方法。 IEvaluator 为我们提供了使用 NHibernate 时调用正确扩展方法所需的可扩展性。
添加 IEvaluator 的实现,如下所示:
public class FetchEvaluator : IEvaluator { private static readonly MethodInfo FetchMethodInfo = typeof(EagerFetchingExtensionMethods) .GetTypeInfo().GetDeclaredMethods(nameof(EagerFetchingExtensionMethods.Fetch)) .Single(); private static readonly MethodInfo FetchManyMethodInfo = typeof(EagerFetchingExtensionMethods) .GetTypeInfo().GetDeclaredMethods(nameof(EagerFetchingExtensionMethods.FetchMany)) .Single(); private static readonly MethodInfo ThenFetchMethodInfo = typeof(EagerFetchingExtensionMethods) .GetTypeInfo().GetDeclaredMethods(nameof(EagerFetchingExtensionMethods.ThenFetch)) .Single(); private static readonly MethodInfo ThenFetchManyMethodInfo = typeof(EagerFetchingExtensionMethods) .GetTypeInfo().GetDeclaredMethods(nameof(EagerFetchingExtensionMethods.ThenFetchMany)) .Single(); public static FetchEvaluator Instance { get; } = new FetchEvaluator(); public IQueryable<T> GetQuery<T>(IQueryable<T> query, ISpecification<T> specification) where T : class { foreach (var includeInfo in specification.IncludeExpressions) { query = includeInfo.Type switch { IncludeTypeEnum.Include => BuildInclude<T>(query, includeInfo), IncludeTypeEnum.ThenInclude => BuildThenInclude<T>(query, includeInfo), _ => query }; } return query; } public bool IsCriteriaEvaluator { get; } = false; private IQueryable<T> BuildInclude<T>(IQueryable query, IncludeExpressionInfo includeInfo) { _ = includeInfo ?? throw new ArgumentNullException(nameof(includeInfo)); var methodInfo = (IsGenericEnumerable(includeInfo.PropertyType, out var propertyType) ? FetchManyMethodInfo : FetchMethodInfo); var method = methodInfo.MakeGenericMethod(includeInfo.EntityType, propertyType); var result = method.Invoke(null, new object[] { query, includeInfo.LambdaExpression }); _ = result ?? throw new TargetException(); return (IQueryable<T>)result; } private IQueryable<T> BuildThenInclude<T>(IQueryable query, IncludeExpressionInfo includeInfo) { _ = includeInfo ?? throw new ArgumentNullException(nameof(includeInfo)); _ = includeInfo.PreviousPropertyType ?? throw new ArgumentNullException(nameof(includeInfo.PreviousPropertyType)); var method = (IsGenericEnumerable(includeInfo.PreviousPropertyType, out var previousPropertyType) ? ThenFetchManyMethodInfo : ThenFetchMethodInfo); IsGenericEnumerable(includeInfo.PropertyType, out var propertyType); var result = method.MakeGenericMethod(includeInfo.EntityType, previousPropertyType, propertyType) .Invoke(null, new object[] { query, includeInfo.LambdaExpression }); _ = result ?? throw new TargetException(); return (IQueryable<T>)result; } private static bool IsGenericEnumerable(Type type, out Type propertyType) { if (type.IsGenericType && (type.GetGenericTypeDefinition() == typeof(IEnumerable<>))) { propertyType = type.GenericTypeArguments[0]; return true; } propertyType = type; return false; } }
接下来我们需要配置 ISpecificationEvaluator 以使用我们的 FetchEvaluator(和其他评估器)。我们添加一个实现 ISpecificationEvaluator ,如下所示,并在构造函数中配置评估器。 WhereEvaluator、OrderEvaluator 和 PaginationEvaluator 都在 Ardalis.Specification 中,并且在 NHibernate 中也能很好地工作。
public class LinqToQuerySpecificationEvaluator : ISpecificationEvaluator { private List<IEvaluator> Evaluators { get; } = new List<IEvaluator>(); public LinqToQuerySpecificationEvaluator() { Evaluators.AddRange(new IEvaluator[] { WhereEvaluator.Instance, OrderEvaluator.Instance, PaginationEvaluator.Instance, FetchEvaluator.Instance }); } public IQueryable<TResult> GetQuery<T, TResult>(IQueryable<T> query, ISpecification<T, TResult> specification) where T : class { if (specification is null) throw new ArgumentNullException(nameof(specification)); if (specification.Selector is null && specification.SelectorMany is null) throw new SelectorNotFoundException(); if (specification.Selector is not null && specification.SelectorMany is not null) throw new ConcurrentSelectorsException(); query = GetQuery(query, (ISpecification<T>)specification); return specification.Selector is not null ? query.Select(specification.Selector) : query.SelectMany(specification.SelectorMany!); } public IQueryable<T> GetQuery<T>(IQueryable<T> query, ISpecification<T> specification, bool evaluateCriteriaOnly = false) where T : class { if (specification is null) throw new ArgumentNullException(nameof(specification)); var evaluators = evaluateCriteriaOnly ? Evaluators.Where(x => x.IsCriteriaEvaluator) : Evaluators; foreach (var evaluator in evaluators) query = evaluator.GetQuery(query, specification); return query; } }
现在我们可以在我们的存储库中创建对 LinqToQuerySpecificationEvaluator 的引用,可能如下所示:
public class Repository : IRepository { private readonly ISession _session; private readonly ISpecificationEvaluator _specificationEvaluator; public Repository(ISession session) { _session = session; _specificationEvaluator = new LinqToQuerySpecificationEvaluator(); } ... other repository methods public IEnumerable<T> List<T>(ISpecification<T> specification) where T : class { return _specificationEvaluator.GetQuery(_session.Query<T>().AsQueryable(), specification).ToList(); } public IEnumerable<TResult> List<T, TResult>(ISpecification<T, TResult> specification) where T : class { return _specificationEvaluator.GetQuery(_session.Query<T>().AsQueryable(), specification).ToList(); } public void Dispose() { _session.Dispose(); } }
就是这样。现在,我们可以在规范中使用 Linq to Query,就像我们通常使用 Ardalis 一样。规范:
public class TrackByName : Specification<Core.Entitites.Track> { public TrackByName(string trackName) { Query.Where(x => x.Name == trackName); } }
现在我们已经介绍了基于 Linq 的查询,让我们继续处理 Criteria API 和 Query Over,这需要不同的方法。
在 NHibernate 中混合 Linq、Criteria 和 Query Over
由于 Criteria API 和 Query Over 有自己的实现来生成 SQL,并且不使用 IQueryable,因此它们与 IEvaluator 接口不兼容。我的解决方案是在这种情况下避免对这些方法使用 IEvaluator 接口,而是关注规范模式的好处。但我也希望能够混搭
我的解决方案中包含 Linq to Query、Criteria 和 Query Over(如果您只需要其中一种实现,您可以根据您的最佳需求进行挑选)。
为了能够做到这一点,我添加了四个继承Specification或Specification的新类
注意: 定义这些类的程序集需要对 NHibernate 的引用,因为我们为 Criteria 和 QueryOver 定义操作,这可以在 NHibernate
中找到
public class CriteriaSpecification<T> : Specification<T> { private Action<ICriteria>? _action; public Action<ICriteria> GetCriteria() => _action ?? throw new NotSupportedException("The criteria has not been specified. Please use UseCriteria() to define the criteria."); protected void UseCriteria(Action<ICriteria> action) => _action = action; } public class CriteriaSpecification<T, TResult> : Specification<T, TResult> { private Action<ICriteria>? _action; public Action<ICriteria> GetCriteria() => _action ?? throw new NotSupportedException("The criteria has not been specified. Please use UseCriteria() to define the criteria."); protected void UseCriteria(Action<ICriteria> action) => _action = action; } public class QueryOverSpecification<T> : Specification<T> { private Action<IQueryOver<T, T>>? _action; public Action<IQueryOver<T, T>> GetQueryOver() => _action ?? throw new NotSupportedException("The Query over has not been specified. Please use the UseQueryOver() to define the query over."); protected void UseQueryOver(Action<IQueryOver<T, T>> action) => _action = action; } public class QueryOverSpecification<T, TResult> : Specification<T, TResult> { private Func<IQueryOver<T, T>, IQueryOver<T, T>>? _action; public Func<IQueryOver<T, T>, IQueryOver<T, T>> GetQueryOver() => _action ?? throw new NotSupportedException("The Query over has not been specified. Please use the UseQueryOver() to define the query over."); protected void UseQueryOver(Func<IQueryOver<T, T>, IQueryOver<T, T>> action) => _action = action; }
然后我们可以在存储库中使用模式匹配来更改使用 NHibernate 进行查询的方式
public IEnumerable<T> List<T>(ISpecification<T> specification) where T : class { return specification switch { CriteriaSpecification<T> criteriaSpecification => _session.CreateCriteria<T>() .Apply(query => criteriaSpecification.GetCriteria().Invoke(query)) .List<T>(), QueryOverSpecification<T> queryOverSpecification => _session.QueryOver<T>() .Apply(queryOver => queryOverSpecification.GetQueryOver().Invoke(queryOver)) .List<T>(), _ => _specificationEvaluator.GetQuery(_session.Query<T>().AsQueryable(), specification).ToList() }; } public IEnumerable<TResult> List<T, TResult>(ISpecification<T, TResult> specification) where T : class { return specification switch { CriteriaSpecification<T, TResult> criteriaSpecification => _session.CreateCriteria<T>() .Apply(query => criteriaSpecification.GetCriteria().Invoke(query)) .List<TResult>(), QueryOverSpecification<T, TResult> queryOverSpecification => _session.QueryOver<T>() .Apply(queryOver => queryOverSpecification.GetQueryOver().Invoke(queryOver)) .List<TResult>(), _ => _specificationEvaluator.GetQuery(_session.Query<T>().AsQueryable(), specification).ToList() }; }
上面的Apply()方法是一个扩展方法,它将查询简化为一行:
public static class QueryExtensions { public static T Apply<T>(this T obj, Action<T> action) { action(obj); return obj; } public static TResult Apply<T, TResult>(this T obj, Func<T, TResult> func) { return func(obj); } }
标准规范示例
注意: 定义这些类的程序集需要对 NHibernate 的引用,因为我们定义 Criteria 的操作,可以在 NHibernate
中找到
public class TrackByNameCriteria : CriteriaSpecification<Track> { public TrackByNameCriteria(string trackName) { this.UseCriteria(criteria => criteria.Add(Restrictions.Eq(nameof(Track.Name), trackName))); } }
查询超规格示例
注意: 定义这些类的程序集需要对 NHibernate 的引用,因为我们定义 QueryOver 的操作,可以在 NHibernate
中找到
public class TrackByNameQueryOver : QueryOverSpecification<Track> { public TrackByNameQueryOver(string trackName) { this.UseQueryOver(queryOver => queryOver.Where(x => x.Name == trackName)); } }
通过扩展 NHibernate 的 Ardalis.Specification,我们解锁了在单个存储库模式中使用 Linq to Query、Criteria API 和 Query Over 的能力。这种方法为 NHibernate 用户提供了高度适应性和强大的解决方案
以上是使用 Linq、Criteria API 和 Query Over 扩展 NHibernate 的 Ardalis.Specification的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
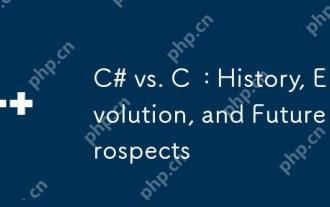
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
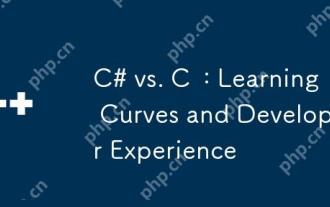
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
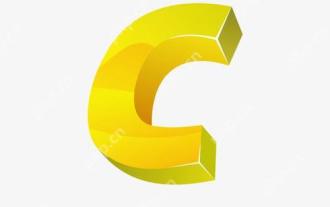
静态分析在C 中的应用主要包括发现内存管理问题、检查代码逻辑错误和提高代码安全性。1)静态分析可以识别内存泄漏、双重释放和未初始化指针等问题。2)它能检测未使用变量、死代码和逻辑矛盾。3)静态分析工具如Coverity能发现缓冲区溢出、整数溢出和不安全API调用,提升代码安全性。
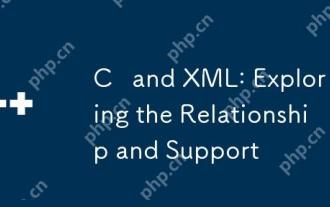
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
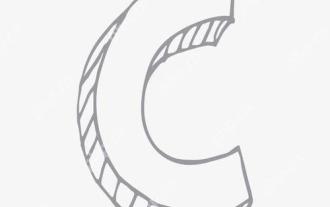
使用C 中的chrono库可以让你更加精确地控制时间和时间间隔,让我们来探讨一下这个库的魅力所在吧。C 的chrono库是标准库的一部分,它提供了一种现代化的方式来处理时间和时间间隔。对于那些曾经饱受time.h和ctime折磨的程序员来说,chrono无疑是一个福音。它不仅提高了代码的可读性和可维护性,还提供了更高的精度和灵活性。让我们从基础开始,chrono库主要包括以下几个关键组件:std::chrono::system_clock:表示系统时钟,用于获取当前时间。std::chron
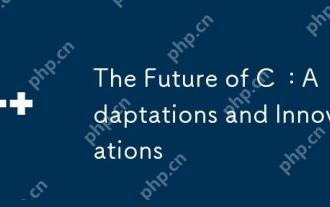
C 的未来将专注于并行计算、安全性、模块化和AI/机器学习领域:1)并行计算将通过协程等特性得到增强;2)安全性将通过更严格的类型检查和内存管理机制提升;3)模块化将简化代码组织和编译;4)AI和机器学习将促使C 适应新需求,如数值计算和GPU编程支持。
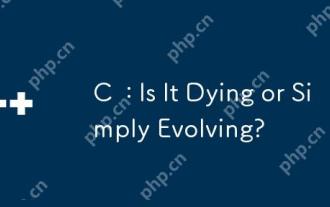
1)c relevantduetoItsAverity and效率和效果临界。2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
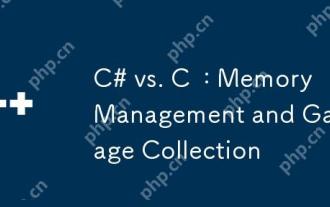
C#使用自动垃圾回收机制,而C 采用手动内存管理。1.C#的垃圾回收器自动管理内存,减少内存泄漏风险,但可能导致性能下降。2.C 提供灵活的内存控制,适合需要精细管理的应用,但需谨慎处理以避免内存泄漏。
