使用 cProfile 和 PyPy 模块优化 Python 代码:完整指南
介绍
作为 Python 开发人员,我们通常先关注如何让代码正常运行,然后再担心优化它。然而,在处理大规模应用程序或性能关键型代码时,优化变得至关重要。在这篇文章中,我们将介绍两个可用于优化 Python 代码的强大工具:cProfile 模块和 PyPy 解释器。
在这篇文章结束时,您将学到:
- 如何使用 cProfile 模块识别性能瓶颈。
- 如何优化代码以提高速度。
- 如何使用 PyPy 通过即时 (JIT) 编译进一步加速您的 Python 程序。
为什么性能优化很重要
Python 以其易用性、可读性和庞大的库生态系统而闻名。但由于其解释性质,它也比 C 或 Java 等其他语言慢。因此,了解如何优化 Python 代码对于性能敏感的应用程序(例如机器学习模型、实时系统或高频交易系统)至关重要。
优化通常遵循以下步骤:
- 分析您的代码以了解瓶颈所在。
- 优化代码效率低下的区域。
- 在更快的解释器(如 PyPy)中运行优化的代码,以实现最大性能。
现在,让我们开始分析您的代码。
步骤 1:使用 cProfile 分析您的代码
什么是cProfile?
cProfile 是一个用于性能分析的内置 Python 模块。它跟踪代码中每个函数执行所需的时间,这可以帮助您识别导致速度变慢的函数或代码部分。
从命令行使用 cProfile
分析脚本的最简单方法是从命令行运行 cProfile。例如,假设您有一个名为 my_script.py 的脚本:
python -m cProfile -s cumulative my_script.py
说明:
- -m cProfile:将 cProfile 模块作为 Python 标准库的一部分运行。
- -scumulative:按每个函数花费的累积时间对分析结果进行排序。
- my_script.py:您的 Python 脚本。
这将生成您的代码花费时间的详细分类。
示例:分析 Python 脚本
让我们看一个递归计算斐波那契数的基本 Python 脚本:
def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2) if __name__ == "__main__": print(fibonacci(30))
使用 cProfile 运行此脚本:
python -m cProfile -s cumulative fibonacci_script.py
了解 cProfile 输出
运行 cProfile 后,您将看到如下内容:
ncalls tottime percall cumtime percall filename:lineno(function) 8320 0.050 0.000 0.124 0.000 fibonacci_script.py:3(fibonacci)
每列提供关键性能数据:
- ncalls:调用函数的次数。
- tottime:函数花费的总时间(不包括子函数)。
- cumtime:函数(包括子函数)所花费的累计时间。
- 每次调用:每次调用的时间。
如果您的斐波那契函数花费太多时间,此输出将告诉您优化工作的重点。
分析代码的特定部分
如果您只想分析特定部分,也可以在代码中以编程方式使用 cProfile。
import cProfile def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2) if __name__ == "__main__": cProfile.run('fibonacci(30)')
第 2 步:优化您的 Python 代码
使用 cProfile 确定代码中的瓶颈后,就可以进行优化了。
常见的Python优化技术
- 使用内置函数:sum()、min() 和 max() 等内置函数在 Python 中经过高度优化,通常比手动实现的循环更快。
示例:
# Before: Custom sum loop total = 0 for i in range(1000000): total += i # After: Using built-in sum total = sum(range(1000000))
- 避免不必要的函数调用:函数调用会产生开销,尤其是在循环内。尽量减少多余的调用。
示例:
# Before: Unnecessary repeated calculations for i in range(1000): print(len(my_list)) # len() is called 1000 times # After: Compute once and reuse list_len = len(my_list) for i in range(1000): print(list_len)
- Memoization:对于递归函数,您可以使用memoization来存储昂贵的计算结果,以避免重复工作。
示例:
from functools import lru_cache @lru_cache(maxsize=None) def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2)
通过存储每个递归调用的结果,大大加快了斐波那契计算的速度。
第 3 步:使用 PyPy 进行即时编译
什么是 PyPy?
PyPy 是另一种 Python 解释器,它使用即时 (JIT) 编译来加速 Python 代码。 PyPy 将频繁执行的代码路径编译为机器代码,使其比某些任务的标准 CPython 解释器快得多。
Installing PyPy
You can install PyPy using a package manager like apt on Linux or brew on macOS:
# On Ubuntu sudo apt-get install pypy3 # On macOS (using Homebrew) brew install pypy3
Running Python Code with PyPy
Once PyPy is installed, you can run your script with it instead of CPython:
pypy3 my_script.py
Why Use PyPy?
- PyPy is ideal for CPU-bound tasks where the program spends most of its time in computation (e.g., loops, recursive functions, number-crunching).
- PyPy’s JIT compiler optimizes the code paths that are executed most frequently, which can result in significant speedups without any code changes.
Step 4: Combining cProfile and PyPy for Maximum Optimization
Now, let’s combine these tools to fully optimize your Python code.
Example Workflow
- Profile your code using cProfile to identify bottlenecks.
- Optimize your code using the techniques we discussed (built-ins, memoization, avoiding unnecessary function calls).
- Run your optimized code with PyPy to achieve additional performance improvements.
Let’s revisit our Fibonacci example and put everything together.
from functools import lru_cache @lru_cache(maxsize=None) def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2) if __name__ == "__main__": import cProfile cProfile.run('print(fibonacci(30))')
After optimizing the code with memoization, run it using PyPy for further performance improvements:
pypy3 fibonacci_script.py
Conclusion
By leveraging cProfile and PyPy, you can greatly optimize your Python code. Use cProfile to identify and address performance bottlenecks in your code. Then, use PyPy to further boost your program’s execution speed through JIT compilation.
In summary:
- Profile your code with cProfile to understand performance bottlenecks.
- Apply Python optimization techniques, such as using built-ins and memoization.
- Run the optimized code on PyPy to achieve even better performance.
With this approach, you can make your Python programs run faster and more efficiently, especially for CPU-bound tasks.
Connect with me:
Github
Linkedin
以上是使用 cProfile 和 PyPy 模块优化 Python 代码:完整指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
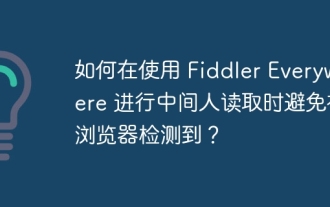
使用FiddlerEverywhere进行中间人读取时如何避免被检测到当你使用FiddlerEverywhere...
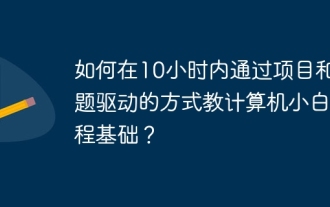
如何在10小时内教计算机小白编程基础?如果你只有10个小时来教计算机小白一些编程知识,你会选择教些什么�...
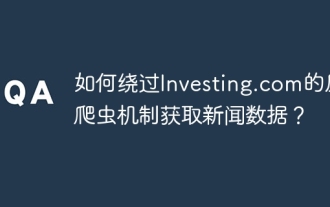
攻克Investing.com的反爬虫策略许多人尝试爬取Investing.com(https://cn.investing.com/news/latest-news)的新闻数据时,常常�...
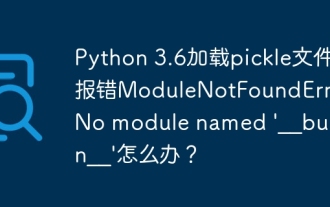
Python3.6环境下加载pickle文件报错:ModuleNotFoundError:Nomodulenamed...
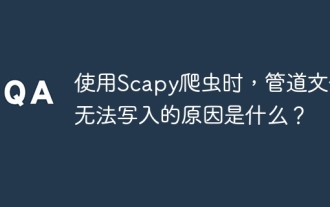
使用Scapy爬虫时管道文件无法写入的原因探讨在学习和使用Scapy爬虫进行数据持久化存储时,可能会遇到管道文�...
