Mastering JavaScript Operators: From Basics to Bitwise
In this blog, we'll dive into the world of JavaScript operators, covering everything from basic arithmetic to bitwise operations. We'll explore terms like "unary," "binary," and "operand," and provide practical examples to help you understand each concept. Let's get started!
Basic Operators
Unary, Binary, and Operand
- Unary operators act on a single operand (e.g., x).
- Binary operators act on two operands (e.g., x + y).
- Operands are the values that operators act upon.
Example:
let x = 5; let y = 3; // Unary operator let negX = -x; // -5 // Binary operator let sum = x + y; // 8
Maths
JavaScript provides a variety of mathematical operators for performing arithmetic operations.
Example:
let a = 10; let b = 3; let addition = a + b; // 13 let subtraction = a - b; // 7 let multiplication = a * b; // 30 let division = a / b; // 3.333... let modulus = a % b; // 1 let exponentiation = a ** b; // 1000
String Concatenation with Binary +
The + operator can also be used to concatenate strings.
Example:
let firstName = "John"; let lastName = "Doe"; let fullName = firstName + " " + lastName; // "John Doe"
Numeric Conversion, Unary +
The unary + operator can convert a value to a number.
Example:
let str = "123"; let num = +str; // 123 console.log(typeof num); // "number"
Operator Precedence
Operator precedence determines the order in which operations are performed.
Example:
let result = 2 + 3 * 4; // 14 (multiplication has higher precedence than addition)
Assignment
The assignment operator = is used to assign values to variables.
Example:
let x = 10; let y = 5; x = y; // x is now 5
Modify-in-Place
Modify-in-place operators combine assignment with another operation.
Example:
let x = 10; x += 5; // x is now 15 x -= 3; // x is now 12 x *= 2; // x is now 24 x /= 4; // x is now 6 x %= 5; // x is now 1
Increment/Decrement
Increment (++) and decrement (--) operators increase or decrease a value by 1.
Example:
let x = 5; x++; // x is now 6 x--; // x is now 5
Bitwise Operators
Bitwise operators perform operations on binary representations of numbers.
Example:
let a = 5; // 0101 in binary let b = 3; // 0011 in binary let andResult = a & b; // 0001 in binary, which is 1 let orResult = a | b; // 0111 in binary, which is 7 let xorResult = a ^ b; // 0110 in binary, which is 6 let notResult = ~a; // -6 (two's complement of 0101) let leftShift = a << 1; // 1010 in binary, which is 10 let rightShift = a >> 1; // 0010 in binary, which is 2
Comma
The comma operator , evaluates both of its operands and returns the value of the second operand.
Example:
let x = (5, 10); // x is 10
Conclusion
Understanding JavaScript operators is crucial for writing efficient and effective code. From basic arithmetic to bitwise operations, each operator has its unique use case. By mastering these operators, you'll be well on your way to becoming a proficient JavaScript developer.
Stay tuned for more in-depth blogs on JavaScript! Happy coding!
以上是Mastering JavaScript Operators: From Basics to Bitwise的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
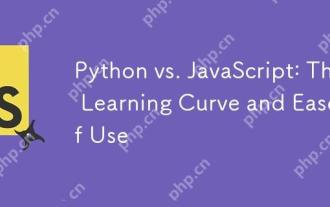
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
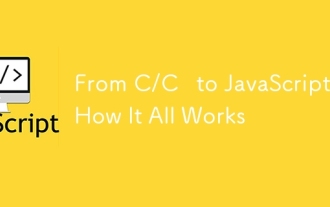
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
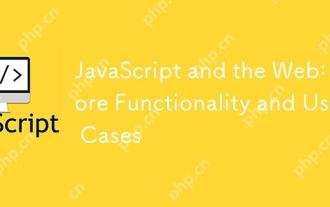
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
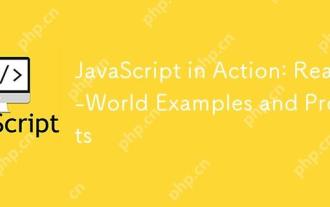
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
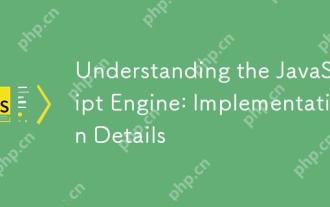
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
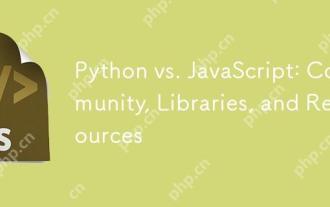
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
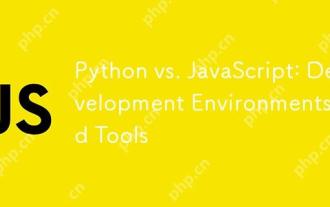
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
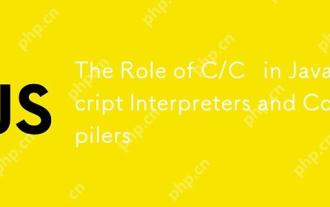
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
