使用 Python 构建强大的数据流平台:实时数据处理综合指南
简介:
数据流平台对于金融、物联网、医疗保健和社交媒体等各个行业高效处理实时数据至关重要。然而,实现一个强大的数据流平台来处理实时摄取、处理、容错和可扩展性需要仔细考虑几个关键因素。
在本文中,我们将使用 Kafka 进行消息代理构建一个基于 Python 的数据流平台,探索实时系统中的各种挑战,并讨论扩展、监控、数据一致性和容错的策略。我们将超越基本示例,涵盖不同领域的用例,例如欺诈检测、预测分析和物联网监控。
1.深入探讨流架构
除了基本组件之外,我们还可以扩展针对不同用例设计的特定架构:
Lambda 架构:
- 批处理层:处理大量历史数据(例如,使用 Apache Spark 或 Hadoop)。
- 速度层:处理实时流数据(使用Kafka Streams)。
- 服务层:组合两个层的结果以提供低延迟查询。
Kappa 建筑:
一个简化版本,仅专注于实时数据处理,没有批处理层。非常适合需要连续处理数据流的环境。
包括这些架构如何在各种场景下处理数据的图表和解释。
2.高级 Kafka 设置
在 Docker 中运行 Kafka(用于云部署)
不用在本地运行 Kafka,而是在 Docker 中运行 Kafka,可以轻松部署在云或生产环境中:
version: '3' services: zookeeper: image: wurstmeister/zookeeper ports: - "2181:2181" kafka: image: wurstmeister/kafka ports: - "9092:9092" environment: KAFKA_ADVERTISED_LISTENERS: INSIDE://kafka:9092,OUTSIDE://localhost:9092 KAFKA_LISTENER_SECURITY_PROTOCOL_MAP: INSIDE:PLAINTEXT,OUTSIDE:PLAINTEXT KAFKA_INTER_BROKER_LISTENER_NAME: INSIDE depends_on: - zookeeper
使用此 Docker 设置可以在生产和云环境中实现更好的可扩展性。
3.使用 Apache Avro 进行架构管理
由于流系统中的数据通常是异构的,因此管理模式对于生产者和消费者之间的一致性至关重要。 Apache Avro 提供紧凑、快速的二进制格式,用于高效序列化大型数据流。
具有 Avro 架构的生产者代码:
from confluent_kafka import avro from confluent_kafka.avro import AvroProducer value_schema_str = """ { "namespace": "example.avro", "type": "record", "name": "User", "fields": [ {"name": "name", "type": "string"}, {"name": "age", "type": "int"} ] } """ value_schema = avro.loads(value_schema_str) def avro_produce(): avroProducer = AvroProducer({ 'bootstrap.servers': 'localhost:9092', 'schema.registry.url': 'http://localhost:8081' }, default_value_schema=value_schema) avroProducer.produce(topic='users', value={"name": "John", "age": 30}) avroProducer.flush() if __name__ == "__main__": avro_produce()
说明:
- 模式注册表: 确保生产者和消费者就模式达成一致。
- AvroProducer: 使用 Avro 处理消息序列化。
4.使用 Apache Kafka Streams 进行流处理
除了使用streamz之外,还引入Kafka Streams作为更高级的流处理库。 Kafka Streams 提供内置的容错、状态处理和一次性语义。
Kafka 流处理器示例:
from confluent_kafka import Consumer, Producer from confluent_kafka.avro import AvroConsumer import json def process_stream(): c = Consumer({ 'bootstrap.servers': 'localhost:9092', 'group.id': 'stream_group', 'auto.offset.reset': 'earliest' }) c.subscribe(['sensor_data']) while True: msg = c.poll(1.0) if msg is None: continue message_data = json.loads(msg.value().decode('utf-8')) # Process the sensor data and detect anomalies if message_data['temperature'] > 100: print(f"Warning! High temperature: {message_data['temperature']}") c.close() if __name__ == "__main__": process_stream()
流处理的关键用例:
- 实时异常检测 (IoT):检测传感器数据中的异常情况。
- 欺诈检测(金融):实时标记可疑交易。
- 预测分析:预测未来事件,例如股票价格变动。
5.处理复杂事件处理 (CEP)
复杂事件处理是数据流平台的一个关键方面,其中分析多个事件以检测随时间变化的模式或趋势。
用例示例:欺诈检测
我们可以实现事件模式,例如在短时间内检测多次失败的登录尝试。
from streamz import Stream # Assuming the event source is streaming failed login attempts def process_event(event): if event['login_attempts'] > 5: print(f"Fraud Alert: Multiple failed login attempts from {event['ip']}") def source(): # Simulate event stream yield {'ip': '192.168.1.1', 'login_attempts': 6} yield {'ip': '192.168.1.2', 'login_attempts': 2} # Apply pattern matching in the stream stream = Stream.from_iterable(source()) stream.map(process_event).sink(print) stream.start()
这展示了如何应用 CEP 进行实时欺诈检测。
6.数据流平台的安全性
在处理实时数据时,安全性常常被忽视,但却至关重要。在本节中,讨论 Kafka 和流平台的加密、身份验证和授权策略。
Kafka Security Configuration:
- TLS Encryption: Secure data in transit by enabling TLS on Kafka brokers.
- SASL Authentication: Implement Simple Authentication and Security Layer (SASL) with either Kerberos or SCRAM.
# server.properties (Kafka Broker) listeners=SASL_SSL://localhost:9093 ssl.keystore.location=/var/private/ssl/kafka.server.keystore.jks ssl.keystore.password=test1234 ssl.key.password=test1234
Access Control in Kafka:
Use ACLs (Access Control Lists) to define who can read, write, or manage Kafka topics.
7. Monitoring & Observability
Real-time monitoring is crucial to ensure smooth functioning. Discuss how to set up monitoring for Kafka and Python applications using tools like Prometheus, Grafana, and Kafka Manager.
Prometheus Metrics for Kafka:
scrape_configs: - job_name: 'kafka' static_configs: - targets: ['localhost:9092'] metrics_path: /metrics scrape_interval: 15s
Logging and Metrics with Python:
Integrate logging and monitoring libraries to track errors and performance:
import logging logging.basicConfig(level=logging.INFO) def process_message(msg): logging.info(f"Processing message: {msg}")
8. Data Sink Options: Batch and Real-time Storage
Discuss how processed data can be stored for further analysis and exploration.
Real-Time Databases:
- TimescaleDB: A PostgreSQL extension for time-series data.
- InfluxDB: Ideal for storing real-time sensor or event data.
Batch Databases:
- PostgreSQL/MySQL: Traditional relational databases for storing transactional data.
- HDFS/S3: For long-term storage of large volumes of data.
9. Handling Backpressure & Flow Control
In data streaming, producers can often overwhelm consumers, causing a bottleneck. We need mechanisms to handle backpressure.
Backpressure Handling with Kafka:
- Set consumer max.poll.records to control how many records the consumer retrieves in each poll.
max.poll.records=500
Implementing Flow Control in Python:
# Limit the rate of message production import time from confluent_kafka import Producer def produce_limited(): p = Producer({'bootstrap.servers': 'localhost:9092'}) for data in range(100): p.produce('stock_prices', key=str(data), value=f"Price-{data}") p.poll(0) time.sleep(0.1) # Slow down the production rate p.flush() if __name__ == "__main__": produce_limited()
10. Conclusion and Future Scope
In this expanded version, we’ve delved into a broad spectrum of challenges and solutions in data streaming platforms. From architecture to security, monitoring, stream processing, and fault tolerance, this guide helps you build a production-ready system for real-time data processing using Python.
Future Enhancements:
- Explore **state
full stream processing** in more detail.
- Add support for exactly-once semantics using Kafka transactions.
- Use serverless frameworks like AWS Lambda to auto-scale stream processing.
Join me to gain deeper insights into the following topics:
- Python
- Data Streaming
- Apache Kafka
- Big Data
- Real-Time Data Processing
- Stream Processing
- Data Engineering
- Machine Learning
- Artificial Intelligence
- Cloud Computing
- Internet of Things (IoT)
- Data Science
- Complex Event Processing
- Kafka Streams
- APIs
- Cybersecurity
- DevOps
- Docker
- Apache Avro
- Microservices
- Technical Tutorials
- Developer Community
- Data Visualization
- Programming
Stay tuned for more articles and updates as we explore these areas and beyond.
以上是使用 Python 构建强大的数据流平台:实时数据处理综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
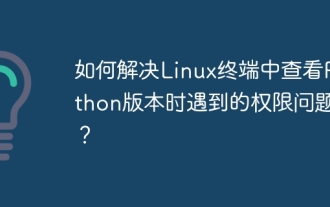
Linux终端中查看Python版本时遇到权限问题的解决方法当你在Linux终端中尝试查看Python的版本时,输入python...
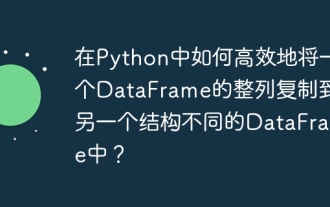
在使用Python的pandas库时,如何在两个结构不同的DataFrame之间进行整列复制是一个常见的问题。假设我们有两个Dat...
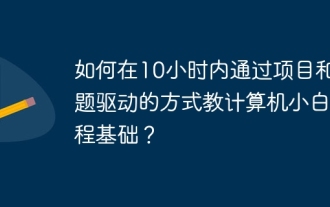
如何在10小时内教计算机小白编程基础?如果你只有10个小时来教计算机小白一些编程知识,你会选择教些什么�...
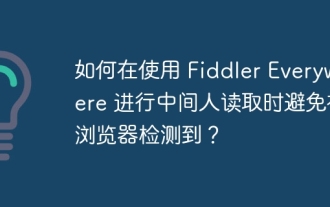
使用FiddlerEverywhere进行中间人读取时如何避免被检测到当你使用FiddlerEverywhere...
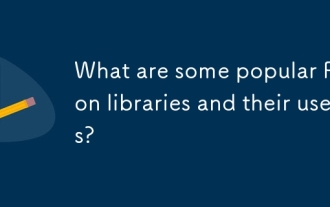
本文讨论了诸如Numpy,Pandas,Matplotlib,Scikit-Learn,Tensorflow,Tensorflow,Django,Blask和请求等流行的Python库,并详细介绍了它们在科学计算,数据分析,可视化,机器学习,网络开发和H中的用途
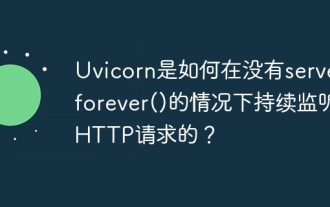
Uvicorn是如何持续监听HTTP请求的?Uvicorn是一个基于ASGI的轻量级Web服务器,其核心功能之一便是监听HTTP请求并进�...
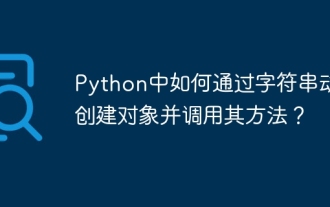
在Python中,如何通过字符串动态创建对象并调用其方法?这是一个常见的编程需求,尤其在需要根据配置或运行...
