C# 基础:从 javascript 开发人员的角度来看
作为一名初级开发人员,我一直害怕学习主要使用 OOP 范式的“旧”编程语言。然而,今天我决定忍住,至少尝试一下。它并不像我想象的那么糟糕,它与 Javascript 有相似之处。让我们先了解一下基础知识。
本博客假设您了解 javascript
基本的
数据类型
与动态类型语言 javascript 不同,C# 是静态类型语言:变量的数据类型在编译时就已知,这意味着程序员必须在变量使用时指定变量的数据类型。声明。
1 2 3 4 5 6 |
|
1 2 3 4 5 |
|
列表/数组
注意:如果使用方法1和2,则无法添加或延长长度
声明和分配List方法1
1 2 |
|
声明和分配List方法2
1 |
|
声明和分配List方法3
1 2 3 |
|
声明和分配多维列表
“,”的数量将决定尺寸
1 2 3 4 |
|
IEnumerable/数组
专门用于枚举或循环的数组。
你可能会问,“和列表有什么区别?”。答案是:
IEnumerable 和 List 之间的一个重要区别(除了一个是接口,另一个是具体类)是 IEnumerable 是只读的,而 List 不是。
1 2 3 |
|
字典/对象
1 2 3 4 5 |
|
运营商
C# 中的运算符的行为与 javascript 非常相似,所以我不会在这里描述它
条件句
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
循环
?使用 foreach 会比常规 for 循环快得多
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
方法
C# 首先是一种面向 OOP 的语言。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
声明命名空间和模型
命名空间用于组织目的,通常用于组织类
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
从 C# 10 开始,我们也可以这样声明命名空间
1 2 3 4 5 6 7 8 9 10 |
|
导入命名空间
1 |
|
以上是C# 基础:从 javascript 开发人员的角度来看的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
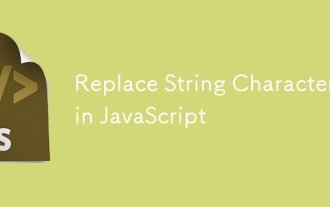
JavaScript字符串替换方法详解及常见问题解答 本文将探讨两种在JavaScript中替换字符串字符的方法:在JavaScript代码内部替换和在网页HTML内部替换。 在JavaScript代码内部替换字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 该方法仅替换第一个匹配项。要替换所有匹配项,需使用正则表达式并添加全局标志g: str = str.replace(/fi
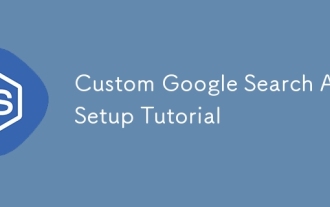
本教程向您展示了如何将自定义的Google搜索API集成到您的博客或网站中,提供了比标准WordPress主题搜索功能更精致的搜索体验。 令人惊讶的是简单!您将能够将搜索限制为Y
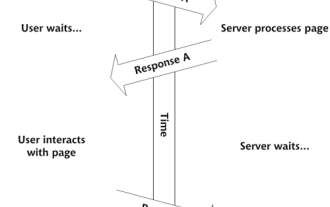
因此,在这里,您准备好了解所有称为Ajax的东西。但是,到底是什么? AJAX一词是指用于创建动态,交互式Web内容的一系列宽松的技术。 Ajax一词,最初由Jesse J创造
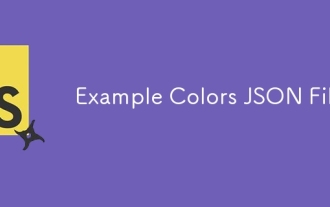
本文系列在2017年中期进行了最新信息和新示例。 在此JSON示例中,我们将研究如何使用JSON格式将简单值存储在文件中。 使用键值对符号,我们可以存储任何类型的
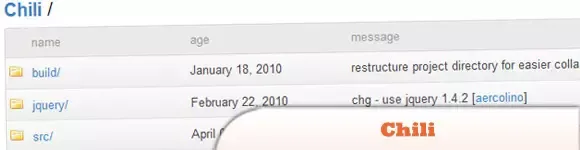
增强您的代码演示:开发人员的10个语法荧光笔 在您的网站或博客上共享代码片段是开发人员的常见实践。 选择合适的语法荧光笔可以显着提高可读性和视觉吸引力。 t
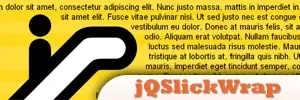
利用轻松的网页布局:8个基本插件 jQuery大大简化了网页布局。 本文重点介绍了简化该过程的八个功能强大的JQuery插件,对于手动网站创建特别有用
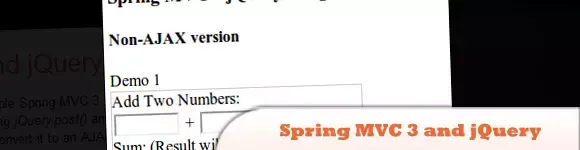
本文介绍了关于JavaScript和JQuery模型视图控制器(MVC)框架的10多个教程的精选选择,非常适合在新的一年中提高您的网络开发技能。 这些教程涵盖了来自Foundatio的一系列主题
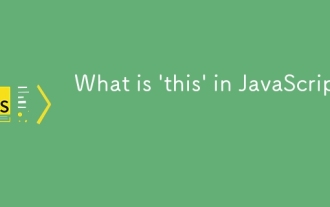
核心要点 JavaScript 中的 this 通常指代“拥有”该方法的对象,但具体取决于函数的调用方式。 没有当前对象时,this 指代全局对象。在 Web 浏览器中,它由 window 表示。 调用函数时,this 保持全局对象;但调用对象构造函数或其任何方法时,this 指代对象的实例。 可以使用 call()、apply() 和 bind() 等方法更改 this 的上下文。这些方法使用给定的 this 值和参数调用函数。 JavaScript 是一门优秀的编程语言。几年前,这句话可
