使用 CopilotKit 创建 AI 聊天机器人:使用 Flask 和 React 的分步指南
使用与 Flask 后端集成的 CopilotKit 创建人工智能聊天机器人可能是一个令人兴奋的项目。以下是有关如何设置项目的分步指南,包括前端(使用 React 和 CopilotKit)和后端(使用 Flask)的必要组件。
项目概况
成分
- Flask 后端:处理 API 请求并为前端提供服务。
- React Frontend:使用 CopilotKit 创建交互式 AI 聊天机器人界面。
- AI 集成:连接到 AI 模型(如 Google 的 Gemini)以生成响应。
第 1 步:设置 Flask 后端
1.安装烧瓶
首先创建虚拟环境并安装Flask:
mkdir chatbot-backend cd chatbot-backend python -m venv venv source venv/bin/activate # On Windows use `venv\Scripts\activate` pip install Flask flask-cors
2. 创建 Flask 应用程序
创建一个名为app.py的文件:
from flask import Flask, request, jsonify from flask_cors import CORS import openai # Make sure to install OpenAI SDK if you're using it app = Flask(__name__) CORS(app) # Allow CORS for all domains # Set your OpenAI API key openai.api_key = 'YOUR_OPENAI_API_KEY' @app.route('/api/chat', methods=['POST']) def chat(): user_message = request.json.get('message') # Call the AI model (e.g., OpenAI GPT) response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[{"role": "user", "content": user_message}] ) bot_message = response.choices[0].message['content'] return jsonify({'response': bot_message}) if __name__ == '__main__': app.run(debug=True)
3. 运行 Flask 应用程序
运行你的 Flask 应用:
python app.py
您的后端现在应该在 http://127.0.0.1:5000 上运行。
第 2 步:使用 CopilotKit 设置 React 前端
1. 创建一个 React 应用程序
在新目录中,创建您的 React 应用程序:
npx create-react-app chatbot-frontend cd chatbot-frontend
2.安装CopilotKit
安装 CopilotKit 和 Axios 以进行 API 调用:
npm install @copilotkit/react axios
3. 创建聊天机器人组件
在src目录下创建一个名为Chatbot.js的文件:
import React, { useState, useEffect } from 'react'; import axios from 'axios'; import { CopilotSidebar, useCopilotChat } from '@copilotkit/react'; import './Chatbot.css'; // Importing CSS file for styling const Chatbot = () => { const [message, setMessage] = useState(''); const [chatHistory, setChatHistory] = useState([]); const [selectedPrompt, setSelectedPrompt] = useState(''); const [conversations, setConversations] = useState([]); const [prompts, setPrompts] = useState([]); const [newPrompt, setNewPrompt] = useState(''); const [searchTerm, setSearchTerm] = useState(''); const [showPromptInput, setShowPromptInput] = useState(false); // State to toggle prompt input visibility const { addMessage } = useCopilotChat(); useEffect(() => { // Load conversations and prompts from local storage on component mount const storedChats = JSON.parse(localStorage.getItem('chatHistory')) || []; const storedPrompts = JSON.parse(localStorage.getItem('prompts')) || []; setConversations(storedChats); setPrompts(storedPrompts); // Load the most recent conversation into chat history if available if (storedChats.length > 0) { setChatHistory(storedChats[storedChats.length - 1].history); } }, []); const handleSendMessage = async () => { const userMessage = { sender: 'User', text: message }; const updatedChatHistory = [...chatHistory, userMessage]; setChatHistory(updatedChatHistory); setMessage(''); try { const response = await axios.post('http://127.0.0.1:5000/api/chat', { message }); const botMessage = { sender: 'Bot', text: response.data.response }; updatedChatHistory.push(botMessage); setChatHistory(updatedChatHistory); // Add messages to Copilot addMessage(userMessage); addMessage(botMessage); // Save updated chat history to local storage localStorage.setItem('chatHistory', JSON.stringify(updatedChatHistory)); } catch (error) { console.error('Error sending message:', error); } }; const handlePromptSelect = (prompt) => { setSelectedPrompt(prompt); setMessage(prompt); }; const handleConversationClick = (conversation) => { setChatHistory(conversation.history); }; const handleSaveConversation = () => { const title = prompt("Enter a title for this conversation:"); if (title) { const newConversation = { title, history: chatHistory }; const updatedConversations = [...conversations, newConversation]; setConversations(updatedConversations); localStorage.setItem('chatHistory', JSON.stringify(updatedConversations)); } }; const handleAddPrompt = () => { if (newPrompt.trim()) { const updatedPrompts = [...prompts, newPrompt.trim()]; setPrompts(updatedPrompts); localStorage.setItem('prompts', JSON.stringify(updatedPrompts)); setNewPrompt(''); setShowPromptInput(false); // Hide the input after adding } }; // Function to delete a conversation const handleDeleteConversation = (index) => { const updatedConversations = conversations.filter((_, i) => i !== index); setConversations(updatedConversations); localStorage.setItem('chatHistory', JSON.stringify(updatedConversations)); // Optionally reset chat history if the deleted conversation was currently loaded if (chatHistory === conversations[index].history) { setChatHistory([]); } }; // Filtered prompts based on search term const filteredPrompts = prompts.filter(prompt => prompt.toLowerCase().includes(searchTerm.toLowerCase()) ); // Filtered conversations based on search term const filteredConversations = conversations.filter(conversation => conversation.title.toLowerCase().includes(searchTerm.toLowerCase()) ); return ( <div className="chatbot-container"> <CopilotSidebar title="Recent Conversations"> <input type="text" placeholder="Search Conversations..." value={searchTerm} onChange={(e) => setSearchTerm(e.target.value)} className="search-input" /> {filteredConversations.map((conversation, index) => ( <div key={index} className="conversation-title"> <span onClick={() => handleConversationClick(conversation)}> {conversation.title} </span> <button className="delete-button" onClick={() => handleDeleteConversation(index)}> ?️ {/* Delete icon */} </button> </div> ))} <button onClick={handleSaveConversation} className="save-button">Save Conversation</button> </CopilotSidebar> <div className="chat-area"> <h2>AI Chatbot</h2> <div className="chat-history"> {chatHistory.map((chat, index) => ( <div key={index} className={`message ${chat.sender === 'User' ? 'user' : 'bot'}`}> <strong>{chat.sender}:</strong> {chat.text} </div> ))} </div> <div className="input-area"> <select value={selectedPrompt} onChange={(e) => handlePromptSelect(e.target.value)} className="prompt-select" > <option value="">Select a prompt...</option> {filteredPrompts.map((prompt, index) => ( <option key={index} value={prompt}>{prompt}</option> ))} </select> {/* Button to toggle visibility of the new prompt input */} <button onClick={() => setShowPromptInput(!showPromptInput)} className="toggle-prompt-button"> {showPromptInput ? "Hide Prompt Input" : "Add New Prompt"} </button> {/* New Prompt Input Field */} {showPromptInput && ( <input type="text" value={newPrompt} onChange={(e) => setNewPrompt(e.target.value)} placeholder="Add a new prompt..." className="new-prompt-input" /> )} {/* Button to add the new prompt */} {showPromptInput && ( <button onClick={handleAddPrompt} className="add-prompt-button">Add Prompt</button> )} {/* Message Input Field */} <input type="text" value={message} onChange={(e) => setMessage(e.target.value)} placeholder="Type your message..." className="message-input" /> <button onClick={handleSendMessage} className="send-button">Send</button> </div> </div> </div> ); }; export default Chatbot;
4.更新App.js
将 src/App.js 的内容替换为:
import React from 'react'; import Chatbot from './Chatbot'; function App() { return ( <div className="App"> <Chatbot /> </div> ); } export default App;
5. 创建Chatbot.css
.chatbot-container { display: flex; height: 100vh; } .chat-area { margin-left: 20px; flex-grow: 1; padding: 20px; background-color: #f9f9f9; border-radius: 8px; box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1); } .chat-history { border: 1px solid #ccc; padding: 10px; height: calc(100% - 120px); /* Adjust height based on input area */ overflow-y: scroll; border-radius: 8px; } .message { margin-bottom: 10px; } .user { text-align: right; } .bot { text-align: left; } .input-area { display: flex; align-items: center; } .prompt-select { margin-right: 10px; } .message-input { flex-grow: 1; padding: 10px; border-radius: 4px; border: 1px solid #ccc; } .send-button { padding: 10px 15px; background-color: #007bff; color: white; border: none; border-radius: 4px; cursor: pointer; } .send-button:hover { background-color: #0056b3; } .conversation-title { padding: 10px; cursor: pointer; } .conversation-title:hover { background-color: #f0f0f0; } .save-button { margin-top: 10px; padding: 5px 10px; background-color: #28a745; color: white; border: none; border-radius: 4px; cursor: pointer; } .save-button:hover { background-color: #218838; } .prompt-select, .new-prompt-input, .message-input { margin-right: 10px; } .search-input { margin-bottom: 10px; } .new-prompt-input { flex-grow: 1; /* Allow it to take remaining space */ padding: 10px; border-radius: 4px; border: 1px solid #ccc; } .add-prompt-button, .send-button, .save-button { padding: 10px 15px; background-color: #007bff; color: white; border: none; border-radius: 4px; cursor: pointer; } .add-prompt-button:hover, .send-button:hover, .save-button:hover { background-color: #0056b3; /* Darker shade for hover */ } .conversation-title { padding: 10px; cursor: pointer; } .conversation-title:hover { background-color: #f0f0f0; /* Highlight on hover */ } .toggle-prompt-button { margin-right: 10px; padding: 10px 15px; background-color: #17a2b8; /* Different color for visibility */ color: white; border: none; border-radius: 4px; cursor: pointer; } .toggle-prompt-button:hover { background-color: #138496; /* Darker shade for hover */ }
5.运行React应用程序
启动你的 React 应用程序:
npm start
您的前端现在应该在 http://localhost:3000 上运行。
第 3 步:测试您的聊天机器人
- 确保您的 Flask 后端和 React 前端都在运行。
- 打开浏览器并导航至 http://localhost:3000。
- 在输入字段中输入消息并查看 AI 聊天机器人的响应。
要在您的 dev.to 帖子中包含 GitHub 存储库 URL,以发布有关使用 CopilotKit、Flask 和 React 构建聊天机器人的信息,您可以将其格式化如下:
使用 CopilotKit、Flask 和 React 构建 AI 聊天机器人
在这篇文章中,我们将逐步介绍使用 CopilotKit 作为前端、Flask 作为后端、React 作为用户界面来创建交互式 AI 聊天机器人的步骤。
GitHub 存储库
您可以在 GitHub 上找到该项目的完整代码:Chatbot with CopilotKit
结论
您现在已经有了一个使用 CopilotKit 作为前端、使用 Flask 作为后端构建的基本 AI 聊天机器人!您可以通过添加用户身份验证、保存聊天历史记录或改进 UI/UX 设计等功能来增强此项目。
以上是使用 CopilotKit 创建 AI 聊天机器人:使用 Flask 和 React 的分步指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
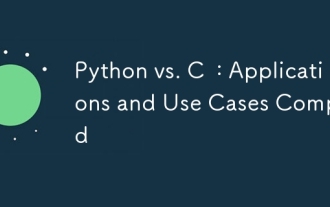
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。 Python以简洁和强大的生态系统着称,C 则以高性能和底层控制能力闻名。
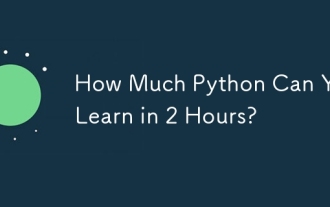
两小时内可以学到Python的基础知识。1.学习变量和数据类型,2.掌握控制结构如if语句和循环,3.了解函数的定义和使用。这些将帮助你开始编写简单的Python程序。
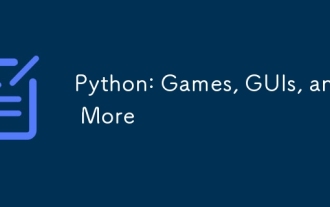
Python在游戏和GUI开发中表现出色。1)游戏开发使用Pygame,提供绘图、音频等功能,适合创建2D游戏。2)GUI开发可选择Tkinter或PyQt,Tkinter简单易用,PyQt功能丰富,适合专业开发。
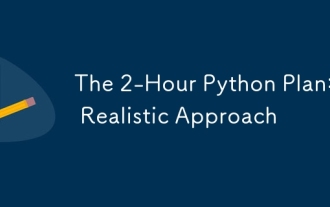
2小时内可以学会Python的基本编程概念和技能。1.学习变量和数据类型,2.掌握控制流(条件语句和循环),3.理解函数的定义和使用,4.通过简单示例和代码片段快速上手Python编程。
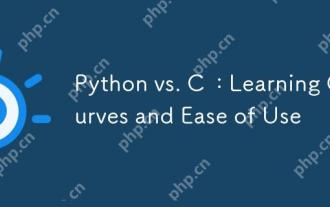
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
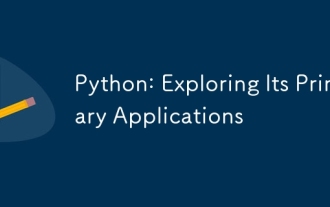
Python在web开发、数据科学、机器学习、自动化和脚本编写等领域有广泛应用。1)在web开发中,Django和Flask框架简化了开发过程。2)数据科学和机器学习领域,NumPy、Pandas、Scikit-learn和TensorFlow库提供了强大支持。3)自动化和脚本编写方面,Python适用于自动化测试和系统管理等任务。
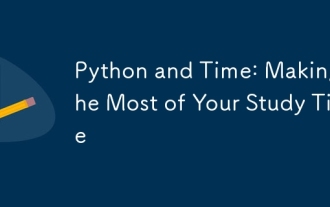
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
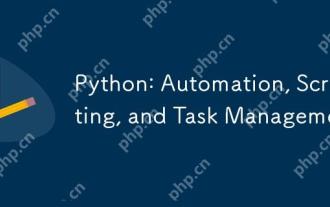
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
