解决 CORS 问题的方法
要解决 CORS 问题,您需要在 Web 服务器(如 Apache 或 Nginx)、后端(如 Django、Go 或 Node.js)中添加适当的标头,或在前端框架(如 React 或 Next.js)中。以下是每个平台的步骤:
1. 网络服务器
阿帕奇
您可以在 Apache 的配置文件(例如 .htaccess、httpd.conf 或 apache2.conf)或特定虚拟主机配置中配置 CORS 标头。
添加以下行以启用 CORS:
<IfModule mod_headers.c> Header set Access-Control-Allow-Origin "*" Header set Access-Control-Allow-Methods "GET, POST, PUT, DELETE, OPTIONS" Header set Access-Control-Allow-Headers "Content-Type, Authorization" </IfModule>
- 要对特定域应用 CORS:
Header set Access-Control-Allow-Origin "https://example.com"
- 如果需要凭据:
Header set Access-Control-Allow-Credentials "true"
确保 mod_headers 模块已启用。如果没有,请使用以下命令启用它:
sudo a2enmod headers sudo systemctl restart apache2
Nginx
在 Nginx 中,您可以在 nginx.conf 或特定服务器块中配置 CORS 标头。
添加以下行:
server { location / { add_header Access-Control-Allow-Origin "*"; add_header Access-Control-Allow-Methods "GET, POST, PUT, DELETE, OPTIONS"; add_header Access-Control-Allow-Headers "Content-Type, Authorization"; } # Optional: Add for handling preflight OPTIONS requests if ($request_method = OPTIONS) { add_header Access-Control-Allow-Origin "*"; add_header Access-Control-Allow-Methods "GET, POST, OPTIONS, PUT, DELETE"; add_header Access-Control-Allow-Headers "Authorization, Content-Type"; return 204; } }
- 如果需要凭据:
add_header Access-Control-Allow-Credentials "true";
然后重新启动Nginx:
sudo systemctl restart nginx
2. 后端框架
姜戈
在 Django 中,您可以使用 django-cors-headers 包添加 CORS 标头。
- 安装包:
pip install django-cors-headers
- 将“corsheaders”添加到 settings.py 中的 INSTALLED_APPS 中:
INSTALLED_APPS = [ ... 'corsheaders', ]
- 将 CORS 中间件添加到您的中间件中:
MIDDLEWARE = [ 'corsheaders.middleware.CorsMiddleware', 'django.middleware.common.CommonMiddleware', ... ]
- 在settings.py中设置允许的来源:
CORS_ALLOWED_ORIGINS = [ "https://example.com", ]
- 允许所有来源:
CORS_ALLOW_ALL_ORIGINS = True
- 如果需要凭据:
CORS_ALLOW_CREDENTIALS = True
- 允许特定的标头或方法:
CORS_ALLOW_HEADERS = ['Authorization', 'Content-Type'] CORS_ALLOW_METHODS = ['GET', 'POST', 'PUT', 'DELETE', 'OPTIONS']
Go(Go 语言)
在 Go 中,您可以在 HTTP 处理程序中手动处理 CORS,或者使用像 rs/cors 这样的中间件。
使用 rs/cors 中间件:
- 安装包:
go get github.com/rs/cors
- 在您的应用程序中使用它:
package main import ( "net/http" "github.com/rs/cors" ) func main() { mux := http.NewServeMux() // Example handler mux.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) }) // CORS middleware handler := cors.New(cors.Options{ AllowedOrigins: []string{"https://example.com"}, // Or use * for all AllowedMethods: []string{"GET", "POST", "PUT", "DELETE", "OPTIONS"}, AllowedHeaders: []string{"Authorization", "Content-Type"}, AllowCredentials: true, }).Handler(mux) http.ListenAndServe(":8080", handler) }
Node.js (Express)
在 Express (Node.js) 中,您可以使用 cors 中间件。
- 安装cors包:
npm install cors
- 在 Express 应用中添加中间件:
const express = require('express'); const cors = require('cors'); const app = express(); // Enable CORS for all routes app.use(cors()); // To allow specific origins app.use(cors({ origin: 'https://example.com', methods: ['GET', 'POST', 'PUT', 'DELETE'], allowedHeaders: ['Authorization', 'Content-Type'], credentials: true })); // Example route app.get('/', (req, res) => { res.send('Hello World'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
3. 前端框架
反应
在 React 中,CORS 由后端处理,但在开发过程中,您可以代理 API 请求以避免 CORS 问题。
- 向 package.json 添加代理:
{ "proxy": "http://localhost:5000" }
这将在开发期间将请求代理到在端口 5000 上运行的后端服务器。
对于生产,后端应该处理 CORS。如果需要,请使用 http-proxy-middleware 等工具进行更多控制。
Next.js
在 Next.js 中,您可以在 API 路由中配置 CORS。
- 为 API 路由创建自定义中间件:
export default function handler(req, res) { res.setHeader('Access-Control-Allow-Origin', '*'); // Allow all origins res.setHeader('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS'); res.setHeader('Access-Control-Allow-Headers', 'Authorization, Content-Type'); if (req.method === 'OPTIONS') { // Handle preflight request res.status(200).end(); return; } // Handle the actual request res.status(200).json({ message: 'Hello from Next.js' }); }
- 在next.config.js中,您还可以修改响应头:
module.exports = { async headers() { return [ { source: '/(.*)', // Apply to all routes headers: [ { key: 'Access-Control-Allow-Origin', value: '*', // Allow all origins }, { key: 'Access-Control-Allow-Methods', value: 'GET, POST, PUT, DELETE, OPTIONS', }, { key: 'Access-Control-Allow-Headers', value: 'Authorization, Content-Type', }, ], }, ]; }, };
在哪里添加标头的摘要:
- Web 服务器(Apache、Nginx):在服务器配置文件中进行配置(例如 .htaccess、nginx.conf)。
-
后端框架:
- Django:使用 django-cors-headers。
- Go:手动添加标头或使用 rs/cors 等中间件。
- Node.js (Express):使用 cors 中间件。
- 前端:在开发中,使用代理设置(如 React 的代理或 Next.js 自定义标头)来避免 CORS 问题,但始终在生产中的后端处理 CORS。
以上是解决 CORS 问题的方法的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
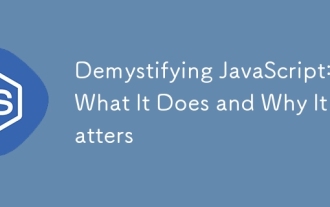
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
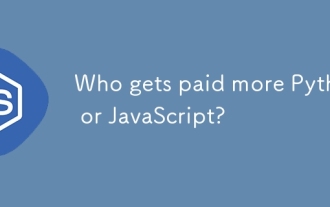
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
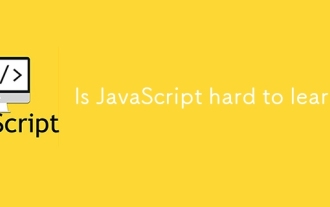
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
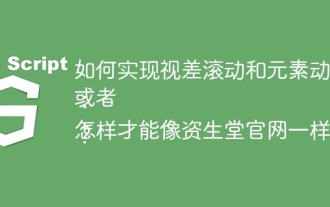
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
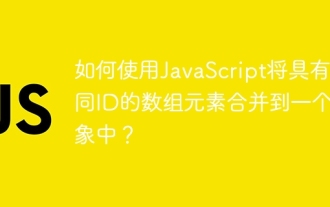
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
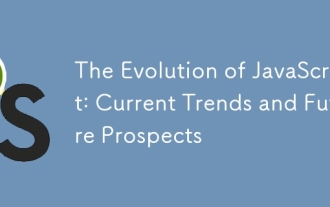
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
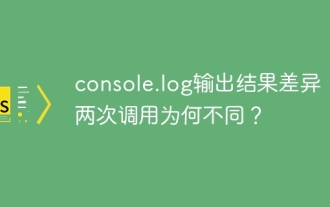
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
