简化 NestJS 中的文件上传:无需磁盘存储即可高效内存解析 CSV 和 XLSX
NestJS 中的轻松文件解析:管理内存中的 CSV 和 XLSX 上传,以提高速度、安全性和可扩展性
介绍
在 Web 应用程序中处理文件上传是一项常见任务,但处理不同的文件类型并确保正确处理它们可能具有挑战性。通常,开发人员需要解析上传的文件而不将其保存到服务器,这对于降低服务器存储成本并确保敏感数据不会被不必要地保留尤为重要。在本文中,我们将逐步介绍创建自定义 NestJS 模块来处理专门针对 CSV 和 XLS/XLSX 文件的文件上传的过程,并且我们将使用 Node.js 流在内存中解析这些文件,因此不会有静态文件在服务器上创建。
为什么选择 NestJS?
NestJS 是一个渐进式 Node.js 框架,它利用 TypeScript 并提供开箱即用的应用程序架构,使您能够构建高度可测试、可扩展、松散耦合且易于维护的应用程序。通过使用 NestJS,我们可以利用其模块化结构、强大的依赖注入系统和广泛的生态系统。
第 1 步:设置项目
在深入研究代码之前,让我们先建立一个新的 NestJS 项目。如果您还没有安装 NestJS CLI:
npm install -g @nestjs/cli
创建一个新的 NestJS 项目:
nest new your-super-name
导航到项目目录:
cd your-super-name
第2步:安装所需的软件包
我们需要安装一些额外的软件包来处理文件上传和解析:
npm install @nestjs/platform-express multer exceljsfile-type
- Multer:处理multipart/form-data的中间件,主要用于上传文件。
- Exlesjs:用于解析 CSV/XLS/XLSX 文件的强大库。
- File-Type:用于检测流或缓冲区的文件类型的库。
步骤 3:创建 Multer 存储引擎而不保存文件
为了自定义文件上传过程,我们将创建一个自定义 Multer 存储引擎。该引擎将确保仅接受 CSV 和 XLS/XLSX 文件,使用 Node.js 流在内存中解析它们,并返回解析后的数据,而不将任何文件保存到磁盘。
为我们的引擎创建一个新文件:
import { PassThrough } from 'stream'; import * as fileType from 'file-type'; import { BadRequestException } from '@nestjs/common'; import { Request } from 'express'; import { Workbook } from 'exceljs'; import { createParserCsvOrXlsx } from './parser-factory.js'; const ALLOWED_MIME_TYPES = [ 'text/csv', 'application/vnd.ms-excel', 'text/comma-separated-values', 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet', 'application/vnd.ms-excel', ] as const; export class CsvOrXlsxMulterEngine { private destKey: string; private maxFileSize: number; constructor(opts: { destKey: string; maxFileSize: number }) { this.destKey = opts.destKey; this.maxFileSize = opts.maxFileSize; } async _handleFile(req: Request, file: any, cb: any) { try { const contentLength = Number(req.headers['content-length']); if ( typeof contentLength === 'number' && contentLength > this.maxFileSize ) { throw new Error(`Max file size is ${this.maxFileSize} bytes.`); } const fileStream = await fileType.fileTypeStream(file.stream); const mime = fileStream.fileType?.mime ?? file.mimetype; if (!ALLOWED_MIME_TYPES.includes(mime)) { throw new BadRequestException('File must be *.csv or *.xlsx'); } const replacementStream = new PassThrough(); fileStream.pipe(replacementStream); const parser = createParserCsvOrXlsx(mime); const data = await parser.read(replacementStream); cb(null, { [this.destKey]: mime === 'text/csv' ? data : (data as Workbook).getWorksheet(), }); } catch (error) { cb(error); } } _removeFile(req: Request, file: any, cb: any) { cb(null); } }
此自定义存储引擎检查文件的 MIME 类型并确保它是 CSV 或 XLS/XLSX 文件。然后,它使用 Node.js 流完全在内存中处理该文件,因此不会在服务器上创建临时文件。这种方法既高效又安全,尤其是在处理敏感数据时。
第 4 步:创建解析器工厂
解析器工厂负责根据文件类型确定合适的解析器。
为我们的解析器创建一个新文件:
import excel from 'exceljs'; export function createParserCsvOrXlsx(mime: string) { const workbook = new excel.Workbook(); return [ 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet', 'application/vnd.ms-excel', ].includes(mime) ? workbook.xlsx : workbook.csv; }
此工厂函数检查 MIME 类型并返回适当的解析器(xlsx 或 csv)。
第5步:在NestJS控制器中配置Multer
接下来,让我们创建一个控制器来使用我们的自定义存储引擎处理文件上传。
生成一个新的控制器:
nest g controller files
在files.controller.ts中,使用Multer和自定义存储引擎配置文件上传:
import { Controller, Post, UploadedFile, UseInterceptors, } from '@nestjs/common'; import { FileInterceptor } from '@nestjs/platform-express'; import { Worksheet } from 'exceljs'; import { CsvOrXlsxMulterEngine } from '../../shared/multer-engines/csv-xlsx/engine.js'; import { FilesService } from './files.service.js'; const MAX_FILE_SIZE_IN_MiB = 1000000000; // Only for test @Controller('files') export class FilesController { constructor(private readonly filesService: FilesService) {} @UseInterceptors( FileInterceptor('file', { storage: new CsvOrXlsxMulterEngine({ maxFileSize: MAX_FILE_SIZE_IN_MiB, destKey: 'worksheet', }), }), ) @Post() create(@UploadedFile() data: { worksheet: Worksheet }) { return this.filesService.format(data.worksheet); } }
该控制器设置一个端点来处理文件上传。上传的文件由 CsvOrXlsxMulterEngine 处理,解析后的数据在响应中返回,而不会保存到磁盘。
第 6 步:设置模块
最后,我们需要设置一个模块来包含我们的控制器。
生成一个新模块:
nest g module files
在files.module.ts中,导入控制器:
import { Module } from '@nestjs/common'; import { FilesController } from './files.controller.js'; import { FilesService } from './files.service.js'; @Module({ providers: [FilesService], controllers: [FilesController], }) export class FilesModule {}
确保将此模块导入到您的 AppModule 中:
第 7 步:使用 HTML 测试文件上传
为了测试文件上传功能,我们可以创建一个简单的 HTML 页面,允许用户上传 CSV 或 XLS/XLSX 文件。此页面会将文件发送到我们的 /api/files 端点,该端点将在内存中进行解析和处理。
这是用于测试文件上传的基本 HTML 文件:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>File Upload</title> </head> <body> <h1>Upload a File (CSV or XLSX)</h1> <form action="/api/files" method="post" enctype="multipart/form-data"> <label for="file">Choose file:</label> <input type="file" id="file" name="file" accept=".csv, .xlsx" required> <br><br> <button type="submit">Upload</button> </form> </body> </html>
为了渲染文件上传的 HTML 页面,我们首先需要安装一个名为 @nestjs/serve-static 的附加 NestJS 模块。您可以通过运行以下命令来完成此操作:
npm install @nestjs/serve-static
安装后,我们需要在AppModule中配置该模块:
import { Module } from '@nestjs/common'; import { join } from 'path'; import { ServeStaticModule } from '@nestjs/serve-static'; import { FilesModule } from './modules/files/files.module.js'; @Module({ imports: [ FilesModule, ServeStaticModule.forRoot({ rootPath: join(new URL('..', import.meta.url).pathname, 'public'), serveRoot: '/', }), ], }) export class AppModule {}
此设置将允许我们从公共目录提供静态文件。现在,我们可以通过在浏览器中导航到http://localhost:3000来打开文件上传页面。
上传您的文件
To upload a file, follow these steps:
- Choose a file by clicking on the ‘Choose file’ button.
- Click on the ‘Upload’ button to start the upload process.
Once the file is uploaded successfully, you should see a confirmation that the file has been uploaded and formatted.
Note: I haven’t included code for formatting the uploaded file, as this depends on the library you choose for processing CSV or XLS/XLSX files. You can view the complete implementation on GitHub.
Comparing Pros and Cons of In-Memory File Processing
When deciding whether to use in-memory file processing or saving files to disk, it’s important to understand the trade-offs.
Pros of In-Memory Processing:
No Temporary Files on Disk:
- Security: Sensitive data isn’t left on the server’s disk, reducing the risk of data leaks.
- Resource Efficiency: The server doesn’t need to allocate disk space for temporary files, which can be particularly useful in environments with limited storage.
Faster Processing:
- Performance: Parsing files in memory can be faster since it eliminates the overhead of writing and reading files from disk.
- Reduced I/O Operations: Fewer disk I/O operations means lower latency and potent ially higher throughput for file processing.
Simplified Cleanup:
- No Cleanup Required: Since files aren’t saved to disk, there’s no need to manage or clean up temporary files, simplifying the codebase.
Cons of In-Memory Processing:
Memory Usage:
- High Memory Consumption: Large files can consume significant amounts of memory, which might lead to out-of-memory errors if the server doesn’t have enough resources.
- Scalability: Handling large files or multiple file uploads simultaneously may require careful memory management and scaling strategies.
File Size Limitations:
- Limited by Memory: The maximum file size that can be processed is limited by the available memory on the server. This can be a signific ant drawback for applications dealing with very large files.
Complexity in Error Handling:
- Error Management: Managing errors in streaming data can be more complex than handling files on disk, especially in cases where partial data might need to be recovered or analyzed.
When to Use In-Memory Processing:
Small to Medium Files: If your application deals with relatively small files, in-memory processing can offer speed and simplicity.
Security-Sensitive Applications: When handling sensitive data that shouldn’t be stored on disk, in-memory processing can reduce the risk of data breaches.
High-Performance Scenarios: Applications that require high throughput and minimal latency may benefit from the reduced overhead of in-memory processing.
When to Consider Disk-Based Processing:
Large Files: If your application needs to process very large files, disk-based processing may be necessary to avoid running out of memory.
Resource-Constrained Environments: In cases where server memory is limited, processing files on disk can prevent memory exhaustion and allow for better resource management.
Persistent Storage Needs: If you need to retain a copy of the uploaded file for auditing, backup, or later retrieval, saving files to disk is necessary.
Integration with External Storage Services: For large files, consider uploading them to external storage services like AWS S3, Google Cloud
- Storage, or Azure Blob Storage. These services allow you to offload storage from your server, and you can process the files in the cloud or retrieve them for in-memory processing as needed.
Scalability: Cloud storage solutions can handle massive files and provide redundancy, ensuring that your data is safe and easily accessible from multiple geographic locations.
Cost Efficiency: Using cloud storage can be more cost-effective for handling large files, as it reduces the need for local server resources and provides pay-as-you-go pricing.
结论
在本文中,我们在 NestJS 中创建了一个自定义文件上传模块,用于处理 CSV 和 XLS/XLSX 文件,在内存中解析它们,并返回解析后的数据,而不将任何文件保存到磁盘。这种方法利用了 Node.js 流的强大功能,使其既高效又安全,因为服务器上不会留下任何临时文件。
我们还探讨了内存中文件处理与将文件保存到磁盘的优缺点。虽然内存处理提供速度、安全性和简单性,但在采用此方法之前考虑内存使用和潜在的文件大小限制非常重要。
无论您是构建企业应用程序还是小型项目,正确处理文件上传和解析都至关重要。通过此设置,您就可以很好地掌握 NestJS 中的文件上传,而无需担心不必要的服务器存储或数据安全问题。
请随时在下面的评论部分分享您的想法和改进!
如果您喜欢本文或发现这些工具很有用,请务必在 Dev.to 上关注我,以获取有关编码和开发的更多见解和技巧。我定期分享有用的内容,让您的编码之旅更加顺利。
在 X (Twitter) 上关注我,我在这里分享更多关于编程和技术的有趣想法、更新和讨论!不要错过 - 点击这些关注按钮。
您还可以在 LinkedIn 上关注我,获取专业见解、最新项目的更新以及有关编码、技术趋势等的讨论。不要错过可以帮助您提高开发技能的有价值的内容 - 让我们联系!
以上是简化 NestJS 中的文件上传:无需磁盘存储即可高效内存解析 CSV 和 XLSX的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
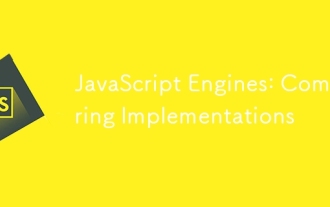
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
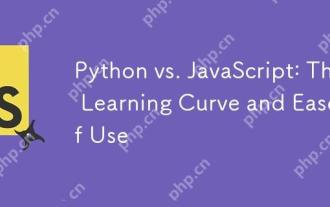
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
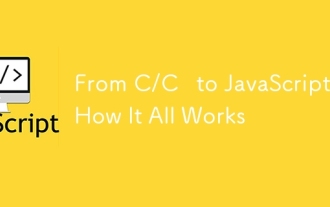
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
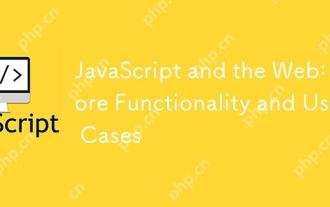
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
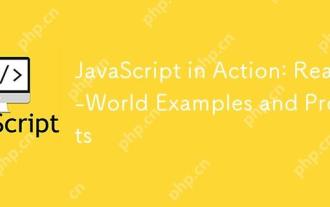
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
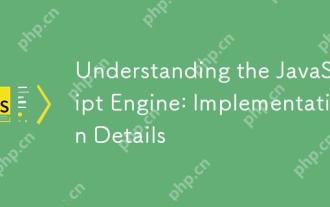
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
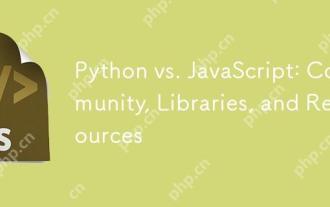
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
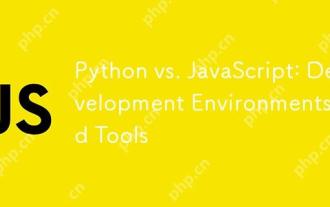
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
