关于 Javascript Promise 的有趣事实
Promise 始终是异步的
Promise 的回调总是在同步代码之后执行
const promise = Promise.resolve(); promise.then(() => console.log('async')); console.log('sync'); //sync //async
连锁承诺返回新承诺
Promise 每次调用时都会返回一个新的 Promise
const p = Promise.resolve(); const chain = p.then(() => {}); console.log(p === chain); //false
就这样永远()
承诺支持无限链接
Promise.resolve(1) .then(value => value + 1) .then(value => value + 1) .then(value => console.log(value)); // 3
您可以将回调转换为承诺
您可以包装使用回调的旧代码,以与现代异步/等待一起使用
function asyncOperation(callback) { setTimeout(() => callback(null, 'Im a callback'), 1000); } const promisified = () => new Promise((resolve, reject) => { asyncOperation((err, result) => { if (err) reject(err); else resolve(result); }); }); promisified().then(result => console.log(result)); // "Im a callback"
Promise.resolve() 并不总是创建新的 Promise
如果您传递一个非 Promise 值,Promise.resolve() 会将其包装为已解决的 Promise。但如果你传递一个 Promise,它只会返回相同的 Promise。
const p1 = Promise.resolve('Hello'); const p2 = Promise.resolve(p1); console.log(p1 === p2); // true
您可以处理链中任何位置的错误
Promise.reject('Error!') .then(() => console.log('This will not run')) .then(() => console.log('This will also not run')) .catch(err => console.log('Caught:', err)) .then(() => console.log('This will run'));
finally() 不传递值
finally() 方法不会接收或修改已解析的值。它用于清理资源并运行,无论 Promise 解决还是拒绝。
Promise.resolve('resolved') .then(value => console.log(value)) .finally(() => console.log('Cleanup')) //resolved //cleanup
承诺一旦确定就不可更改
一旦 Promise 被解决(解决或拒绝),它的状态就是不可变的。此后无法更改,即使您再次尝试解决/拒绝它。
const p = new Promise((resolve, reject) => { resolve('First'); resolve('Second'); }); p.then(value => console.log(value)); //"First" (only the first value is used)
您可以链接 catch() 来处理特定错误
Promise.reject('type C error') .catch(err => { if (err === 'type A error') console.log('handle type A'); throw err; }) .catch(err => { if (err === 'type B error') console.log('handle type B'); throw err; }) .catch(err => { if (err === 'type C error') console.log('handle type C'); throw err; })
您可以将await 与非promise 值一起使用
async function demo() { const result = await 42; //not a promise console.log(result); } demo(); //42
就是这样!感谢您阅读本文。下次见!
以上是关于 Javascript Promise 的有趣事实的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
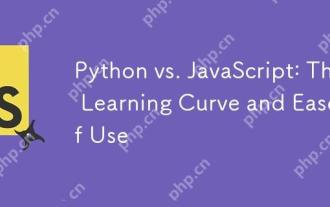
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
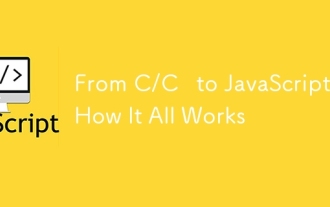
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
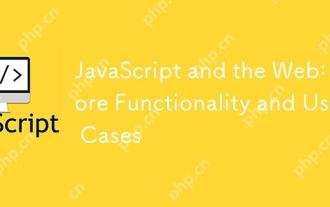
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
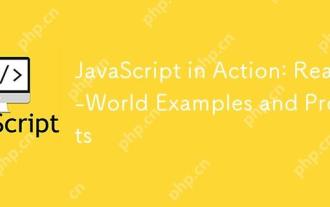
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
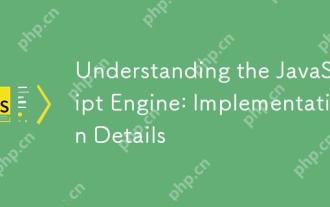
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
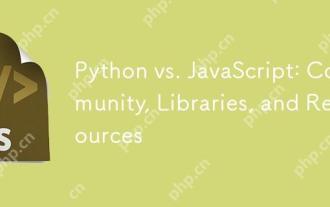
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
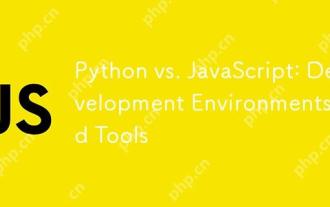
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
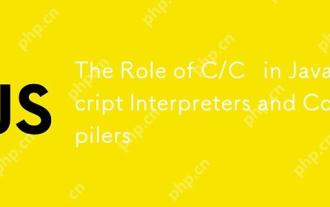
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
