JavaScript 的有趣之处以及 TypeScript 如何让它变得更好
JavaScript 是我们都喜欢的语言,对吧?它灵活、轻便,并且可以随处运行。但尽管它很伟大,但说实话,它可能很奇怪。那种奇怪的感觉会让你在看到一些不应该起作用的东西后质疑自己的理智。
在本文中,我们将探讨 JavaScript 中的一些怪癖 - 那些在您最意想不到的时候让您感到惊讶的行为。幸运的是,对于开发者来说,有一位身穿闪亮盔甲的骑士,叫做TypeScript。我们在这里向您展示如何通过使这些 JavaScript 的怪异更易于管理来避免您烦恼。
1. == vs === 大辩论
JavaScript 为我们提供了两种相等性:== 或松散相等和 === 或严格相等。
console.log(0 == '0'); // true console.log(0 === '0'); // false
等等,什么?是的,JavaScript 使 0 和 '0' 在 == 时被视为相等,但在 === 时则不然。这是因为 == 在进行比较之前会进行 类型强制 或类型转换。它试图为您提供帮助,将该字符串转换为数字,但是这个帮助会导致错误。
想象一下在用户输入上使用 == 来检查数字。当类型不同导致难以追踪的意外行为时,您可能会得到正确的结果。为什么这很重要?因为 JavaScript 的强制类型通常会起作用,直到它破坏了一些重要的东西。
TypeScript 如何提供帮助
TypeScript 已经强制执行开箱即用的类型安全。如果你比较两个不同类型的东西,在你运行任何代码之前它就会对你大喊大叫:
let a: number = 0; let b: string = '0'; console.log(a === b); // TypeScript Error: This comparison is invalid
将数字与字符串进行比较时任何惊喜都消失了。 TypeScript 确保您始终比较苹果和苹果,或者在这种情况下进行数字与数字的比较。
2. 神秘的 undefined 与 null
undefined 和 null 都表示什么都没有,但方式略有不同。 undefined 是 JavaScript 分配给尚未初始化的变量的值,而 null 用于当您有意分配空值时。它们各不相同,但又相似得令人困惑。
let foo; console.log(foo); // undefined let bar = null; console.log(bar); // null
除非你很小心,否则你可能最终会检查其中一个而不是另一个,这会导致一些令人困惑的错误。
if (foo == null) { console.log("This catches both undefined and null"); }
这可行,但如果您没有清楚地区分两者,可能会导致微妙的错误。
TypeScript 如何提供帮助
TypeScript 鼓励您明确而准确地判断某些内容是否可以为 null 或未定义。它通过让您显式处理这两种情况来实现这一点,这样您就可以确定发生了什么:
let foo: number | undefined; let bar: number | null = null; // TypeScript will enforce these constraints foo = null; // Error bar = 5; // No problem!
使用 TypeScript,您可以决定允许哪些类型,这样就不会意外地混合类型。这种严格性可以保护您免受忘记检查 null 或未定义的错误的影响。
3. NaN(非数字)的奇怪情况
你遇到过可怕的 NaN 吗?它是 Not-a-Number, 的缩写,当您尝试执行没有意义的数学运算时,它会弹出。
console.log(0 / 0); // NaN console.log("abc" - 5); // NaN
这里有一个问题:NaN 实际上是数字类型。没错,不是数字是一个数字!
console.log(typeof NaN); // "number"
如果您没有明确检查 NaN,这可能会导致一些真正奇怪的结果。更糟糕的是,NaN 永远不会等于它自己,所以你不能轻易地比较它来检查它是否存在。
console.log(NaN === NaN); // false
TypeScript 如何提供帮助
TypeScript 可以通过在编译时强制执行适当的类型检查和捕获错误操作来缓解此问题。如果 TypeScript 可以推断某个操作将返回 NaN,那么它甚至可以在代码运行之前抛出错误。
let result: number = 0 / 0; // Warning: Possible 'NaN'
TypeScript 还可以帮助您缩小 NaN 可能出现的时间和位置,从而鼓励更好地处理数值。
4. 狂野这
JavaScript 中的 this 是最强大但最容易被误解的概念之一。这个值完全取决于如何调用函数,这可能会在某些上下文中导致意外的行为。
const person = { name: 'Alice', greet() { console.log('Hello, ' + this.name); } }; setTimeout(person.greet, 1000); // Uh-oh, what happened here?
您可能期望在一秒钟后看到“Hello, Alice”打印出来,但相反,您会得到 Hello, undefined。为什么?因为setTimeout里面的this指的是全局对象,而不是person对象。
TypeScript 如何提供帮助
TypeScript 可以通过使用没有自己的 this 的箭头函数来帮助您避免此类问题,并保留它们所在对象的上下文。
const person = { name: 'Alice', greet: () => { console.log('Hello, ' + person.name); // Always refers to 'person' } }; setTimeout(person.greet, 1000); // No more surprises!
这种行为不会再出乎意料了。 TypeScript 迫使您考虑上下文并帮助您正确绑定它,从而降低出现奇怪的未定义错误的风险。
5. Function Hoisting: When Order Does Not Matter
JavaScript functions are hoisted to the top of the scope; that means you can invoke them even before you have declared them in your code. This is kind of a cool trick, but can also be confusing if you are not paying attention to what's going on.
greet(); function greet() { console.log('Hello!'); }
While this can be convenient, it can also cause confusion, especially when you're trying to debug your code.
This works just fine, because of function declaration hoisting. But it can make your code harder to follow, especially for other developers (or yourself after a few months away from the project).
How TypeScript Helps
TypeScript does not change how hoisting works but it gives you clearer feedback about your code's structure. If you accidentally called a function before it is defined, TypeScript will let you know immediately.
greet(); // Error: 'greet' is used before it’s defined function greet() { console.log('Hello!'); }
TypeScript forces you to do some cleanup, where your functions are declared before they are used. It makes your code much more maintainable this way.
Wrapping It Up
JavaScript is an amazing language, but it can certainly be quirky at times. By using TypeScript, you can tame some of JavaScript’s weirdest behaviors and make your code safer, more reliable, and easier to maintain. Whether you’re working with null and undefined, taming this, or preventing NaN disasters, TypeScript gives you the tools to avoid the headaches that can arise from JavaScript’s flexible—but sometimes unpredictable—nature.
So next time you find yourself puzzling over a strange JavaScript quirk, remember: TypeScript is here to help!
Happy coding!
以上是JavaScript 的有趣之处以及 TypeScript 如何让它变得更好的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
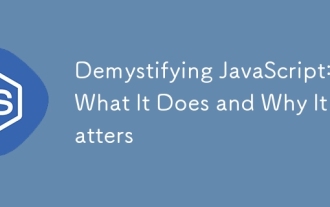
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
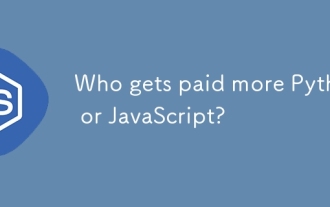
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
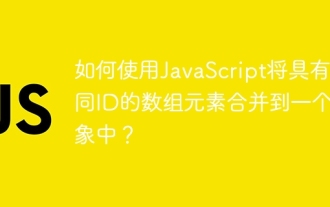
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
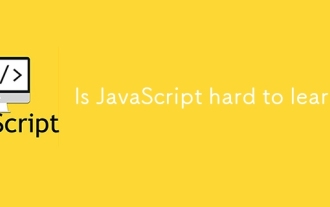
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
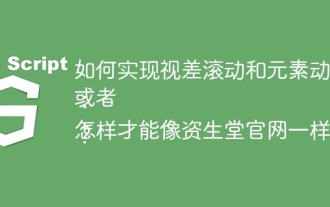
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
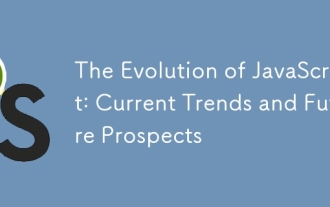
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
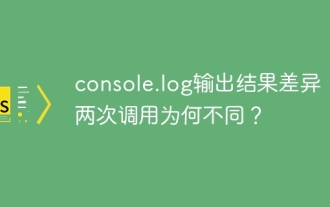
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
