数据库迁移对于 Golang 服务,为什么重要?
数据库迁移,为什么重要?
您是否曾经遇到过这样的情况:当您使用更新的数据库架构在生产环境中部署新的更新时,但之后出现错误并需要恢复内容......这就是迁移出现的情况。
数据库迁移有几个关键目的:
- 架构演变:随着应用程序的演变,它们的数据模型也会发生变化。迁移允许开发人员系统地更新数据库架构以反映这些更改,确保数据库结构与应用程序代码匹配。
- 版本控制:迁移提供了一种对数据库架构进行版本控制的方法,允许团队跟踪一段时间内的更改。此版本控制有助于理解数据库的演变并有助于开发人员之间的协作。
- 跨环境的一致性:迁移确保数据库架构在不同环境(开发、测试、生产)中保持一致。这降低了可能导致错误和集成问题的差异风险。
- 回滚功能:许多迁移工具都支持回滚更改,允许开发人员在迁移导致问题时恢复到数据库之前的状态。这增强了开发和部署过程中的稳定性。
- 自动部署:迁移可以作为部署过程的一部分实现自动化,确保将必要的架构更改应用于数据库,而无需手动干预。这简化了发布流程并减少了人为错误。
在golang项目中应用
要使用 GORM 和 MySQL 为 Golang 服务创建全面的生产级设置,以便轻松迁移、更新和回滚,您需要包含迁移工具、处理数据库连接池并确保正确的结构定义。这是一个完整的示例来指导您完成整个过程:
项目结构
/golang-service |-- main.go |-- database | |-- migration.go |-- models | |-- user.go |-- config | |-- config.go |-- migrations | |-- ... |-- go.mod
1.数据库配置(config/config.go)
package config import ( "fmt" "log" "os" "time" "github.com/joho/godotenv" "gorm.io/driver/mysql" "gorm.io/gorm" ) var DB *gorm.DB func ConnectDB() { err := godotenv.Load() if err != nil { log.Fatal("Error loading .env file") } // charset=utf8mb4: Sets the character set to utf8mb4, which supports all Unicode characters, including emojis. // parseTime=True: Tells the driver to automatically parse DATE and DATETIME values into Go's time.Time type. // loc=Local: Uses the local timezone of the server for time-related queries and storage. dsn := fmt.Sprintf( "%s:%s@tcp(%s:%s)/%s?charset=utf8mb4&parseTime=True&loc=Local", os.Getenv("DB_USER"), os.Getenv("DB_PASS"), os.Getenv("DB_HOST"), os.Getenv("DB_PORT"), os.Getenv("DB_NAME"), ) db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic("failed to connect database") } sqlDB, err := db.DB() if err != nil { panic("failed to configure database connection") } // Set connection pool settings sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour) // 1.sqlDB.SetMaxIdleConns(10) // Sets the maximum number of idle (unused but open) connections in the connection pool. // A value of 10 means up to 10 connections can remain idle, ready to be reused. // 2. sqlDB.SetMaxOpenConns(100): // Sets the maximum number of open (active or idle) connections that can be created to the database. // A value of 100 limits the total number of connections, helping to prevent overloading the database. // 3. sqlDB.SetConnMaxLifetime(time.Hour): // Sets the maximum amount of time a connection can be reused before it’s closed. // A value of time.Hour means that each connection will be kept for up to 1 hour, after which it will be discarded and a new connection will be created if needed. DB = db }
2. 数据库迁移(database/migration.go)
package database import ( "golang-service/models" "golang-service/migrations" "gorm.io/gorm" ) func Migrate(db *gorm.DB) { db.AutoMigrate(&models.User{}) // Apply additional custom migrations if needed }
3.模型(models/user.go)
package models import "gorm.io/gorm" type User struct { gorm.Model Name string `json:"name"` }
4.环境配置(.env)
DB_USER=root DB_PASS=yourpassword DB_HOST=127.0.0.1 DB_PORT=3306 DB_NAME=yourdb
5. 主入口点(main.go)
package main import ( "golang-service/config" "golang-service/database" "golang-service/models" "github.com/gin-gonic/gin" "gorm.io/gorm" ) func main() { config.ConnectDB() database.Migrate(config.DB) r := gin.Default() r.POST("/users", createUser) r.GET("/users/:id", getUser) r.Run(":8080") } func createUser(c *gin.Context) { var user models.User if err := c.ShouldBindJSON(&user); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } if err := config.DB.Create(&user).Error; err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } c.JSON(201, user) } func getUser(c *gin.Context) { id := c.Param("id") var user models.User if err := config.DB.First(&user, id).Error; err != nil { c.JSON(404, gin.H{"error": "User not found"}) return } c.JSON(200, user) }
6、说明:
- 数据库配置:管理连接池以获得生产级性能。
- 迁移文件:(在迁移文件夹中)帮助对数据库架构进行版本控制。
- GORM 模型:将数据库表映射到 Go 结构。
- 数据库迁移:(在数据库文件夹中)随着时间的推移更改表的自定义逻辑,以便轻松回滚。
- 测试:您可以使用 httptest 和 testify 为此设置创建集成测试。
7. 创建第一个迁移
-
对于生产环境,我们可以使用像 golang-migrate 这样的迁移库来应用、回滚或重做迁移。
安装golang-migrate:
go install -tags 'mysql' github.com/golang-migrate/migrate/v4/cmd/migrate@latest
登录后复制登录后复制 -
为用户表生成迁移文件
migrate create -ext=sql -dir=./migrations -seq create_users_table
登录后复制登录后复制运行命令后,我们将得到一对 .up.sql (用于更新架构)和 down.sql (用于稍后可能的回滚)。数字000001是自动生成的迁移索引。
/golang-service |-- migrations | |-- 000001_create_users_table.down.sql | |-- 000001_create_users_table.up.sql
登录后复制添加相关sql命令到.up文件和.down文件。
000001_create_users_table.up.sql
CREATE TABLE users ( id BIGINT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, created_at DATETIME, updated_at DATETIME, deleted_at DATETIME);
登录后复制000001_create_users_table.down.sql
DROP TABLE IF EXISTS users;
登录后复制运行向上迁移并使用以下命令将更改应用到数据库(-verbose 标志以查看更多日志详细信息):
migrate -path ./migrations -database "mysql://user:password@tcp(localhost:3306)/dbname" -verbose up
登录后复制如果我们遇到迁移问题,我们可以使用以下命令查看当前的迁移版本及其状态:
migrate -path ./migrations -database "mysql://user:password@tcp(localhost:3306)/dbname" version
登录后复制如果由于某些原因导致迁移失败,我们可以考虑使用强制(谨慎使用)命令以及脏迁移的版本号。如果版本是1(可以在migrations或schema_migrations表中检查),我们将运行:
migrate -path ./migrations -database "mysql://user:password@tcp(localhost:3306)/dbname" force 1
登录后复制
8.改变计划
-
在某个时间点,我们可能想添加新功能,其中一些可能需要更改数据方案,例如我们想向用户表添加电子邮件字段。我们将按照以下方式进行。
进行新的迁移,将电子邮件列添加到用户表
migrate create -ext=sql -dir=./migrations -seq add_email_to_users
登录后复制现在我们有了一对新的 .up.sql 和 .down.sql
/golang-service |-- migrations | |-- 000001_create_users_table.down.sql | |-- 000001_create_users_table.up.sql | |-- 000002_add_email_to_users.down.sql | |-- 000002_add_email_to_users.up.sql
登录后复制 -
将以下内容添加到*_add_email_to_users.*.sql文件
000002_add_email_to_users.up.sql
/golang-service |-- main.go |-- database | |-- migration.go |-- models | |-- user.go |-- config | |-- config.go |-- migrations | |-- ... |-- go.mod
登录后复制登录后复制登录后复制000002_add_email_to_users.down.sql
package config import ( "fmt" "log" "os" "time" "github.com/joho/godotenv" "gorm.io/driver/mysql" "gorm.io/gorm" ) var DB *gorm.DB func ConnectDB() { err := godotenv.Load() if err != nil { log.Fatal("Error loading .env file") } // charset=utf8mb4: Sets the character set to utf8mb4, which supports all Unicode characters, including emojis. // parseTime=True: Tells the driver to automatically parse DATE and DATETIME values into Go's time.Time type. // loc=Local: Uses the local timezone of the server for time-related queries and storage. dsn := fmt.Sprintf( "%s:%s@tcp(%s:%s)/%s?charset=utf8mb4&parseTime=True&loc=Local", os.Getenv("DB_USER"), os.Getenv("DB_PASS"), os.Getenv("DB_HOST"), os.Getenv("DB_PORT"), os.Getenv("DB_NAME"), ) db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic("failed to connect database") } sqlDB, err := db.DB() if err != nil { panic("failed to configure database connection") } // Set connection pool settings sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour) // 1.sqlDB.SetMaxIdleConns(10) // Sets the maximum number of idle (unused but open) connections in the connection pool. // A value of 10 means up to 10 connections can remain idle, ready to be reused. // 2. sqlDB.SetMaxOpenConns(100): // Sets the maximum number of open (active or idle) connections that can be created to the database. // A value of 100 limits the total number of connections, helping to prevent overloading the database. // 3. sqlDB.SetConnMaxLifetime(time.Hour): // Sets the maximum amount of time a connection can be reused before it’s closed. // A value of time.Hour means that each connection will be kept for up to 1 hour, after which it will be discarded and a new connection will be created if needed. DB = db }
登录后复制登录后复制登录后复制再次运行向上迁移命令以更新数据模式
package database import ( "golang-service/models" "golang-service/migrations" "gorm.io/gorm" ) func Migrate(db *gorm.DB) { db.AutoMigrate(&models.User{}) // Apply additional custom migrations if needed }
登录后复制登录后复制登录后复制我们还需要更新 golang users 结构(添加 Email 字段)以使其与新模式保持同步..
package models import "gorm.io/gorm" type User struct { gorm.Model Name string `json:"name"` }
登录后复制登录后复制登录后复制
9. 回滚迁移:
如果由于某些原因我们在新更新的模式中出现错误,并且需要回滚,这种情况下我们将使用 down 命令:
DB_USER=root DB_PASS=yourpassword DB_HOST=127.0.0.1 DB_PORT=3306 DB_NAME=yourdb
数字 1 表示我们要回滚 1 个迁移。
这里我们还需要手动更新 golang users 结构(删除 Email 字段)以反映数据架构更改。
package main import ( "golang-service/config" "golang-service/database" "golang-service/models" "github.com/gin-gonic/gin" "gorm.io/gorm" ) func main() { config.ConnectDB() database.Migrate(config.DB) r := gin.Default() r.POST("/users", createUser) r.GET("/users/:id", getUser) r.Run(":8080") } func createUser(c *gin.Context) { var user models.User if err := c.ShouldBindJSON(&user); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } if err := config.DB.Create(&user).Error; err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } c.JSON(201, user) } func getUser(c *gin.Context) { id := c.Param("id") var user models.User if err := config.DB.First(&user, id).Error; err != nil { c.JSON(404, gin.H{"error": "User not found"}) return } c.JSON(200, user) }
10. 与 Makefile 一起使用
为了简化迁移和回滚的过程,我们可以添加一个Makefile。
go install -tags 'mysql' github.com/golang-migrate/migrate/v4/cmd/migrate@latest
Makefile 内容如下。
migrate create -ext=sql -dir=./migrations -seq create_users_table
现在我们只需在 CLI 上运行 make migrate_up 或 make migrate_down 即可进行迁移和回滚。
11.注意事项:
- 回滚期间数据丢失:回滚删除列或表的迁移可能会导致数据丢失,因此在运行回滚之前始终备份数据。
- CI/CD 集成:将迁移过程集成到 CI/CD 管道中,以在部署期间自动执行架构更改。
- 数据库备份:安排定期数据库备份,以防止迁移错误时丢失数据。
关于数据库备份
在回滚迁移或进行可能影响数据库的更改之前,以下是需要考虑的一些关键点。
- 架构更改:如果迁移涉及更改架构(例如,添加或删除列、更改数据类型),则回滚到之前的迁移可能会导致存储在这些更改的列或表中的任何数据丢失.
- 数据删除:如果迁移包含删除数据的命令(例如删除表或截断表),则回滚将执行相应的“向下”迁移,这可能会永久删除该数据。
- 事务处理:如果您的迁移工具支持事务,则回滚可能会更安全,因为更改是在事务中应用的。但是,如果您在事务之外手动运行 SQL 命令,则存在丢失数据的风险。
- 数据完整性:如果您以取决于当前架构的方式修改了数据,则回滚可能会使您的数据库处于不一致的状态。
所以备份数据至关重要。这是一个简短的指南:
-
数据库转储:
使用特定于数据库的工具创建数据库的完整备份。对于 MySQL,您可以使用:
/golang-service |-- main.go |-- database | |-- migration.go |-- models | |-- user.go |-- config | |-- config.go |-- migrations | |-- ... |-- go.mod
登录后复制登录后复制登录后复制这将创建一个文件 (backup_before_rollback.sql),其中包含 dbname 数据库的所有数据和架构。
-
导出特定表:
如果您只需要备份某些表,请在 mysqldump 命令中指定它们:
package config import ( "fmt" "log" "os" "time" "github.com/joho/godotenv" "gorm.io/driver/mysql" "gorm.io/gorm" ) var DB *gorm.DB func ConnectDB() { err := godotenv.Load() if err != nil { log.Fatal("Error loading .env file") } // charset=utf8mb4: Sets the character set to utf8mb4, which supports all Unicode characters, including emojis. // parseTime=True: Tells the driver to automatically parse DATE and DATETIME values into Go's time.Time type. // loc=Local: Uses the local timezone of the server for time-related queries and storage. dsn := fmt.Sprintf( "%s:%s@tcp(%s:%s)/%s?charset=utf8mb4&parseTime=True&loc=Local", os.Getenv("DB_USER"), os.Getenv("DB_PASS"), os.Getenv("DB_HOST"), os.Getenv("DB_PORT"), os.Getenv("DB_NAME"), ) db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic("failed to connect database") } sqlDB, err := db.DB() if err != nil { panic("failed to configure database connection") } // Set connection pool settings sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour) // 1.sqlDB.SetMaxIdleConns(10) // Sets the maximum number of idle (unused but open) connections in the connection pool. // A value of 10 means up to 10 connections can remain idle, ready to be reused. // 2. sqlDB.SetMaxOpenConns(100): // Sets the maximum number of open (active or idle) connections that can be created to the database. // A value of 100 limits the total number of connections, helping to prevent overloading the database. // 3. sqlDB.SetConnMaxLifetime(time.Hour): // Sets the maximum amount of time a connection can be reused before it’s closed. // A value of time.Hour means that each connection will be kept for up to 1 hour, after which it will be discarded and a new connection will be created if needed. DB = db }
登录后复制登录后复制登录后复制 验证备份:
确保备份文件已创建并检查其大小或打开它以确保它包含必要的数据。安全地存储备份:
将备份副本保存在安全位置,例如云存储或单独的服务器,以防止回滚过程中数据丢失。
云端备份
要在使用 Golang 并部署在 AWS EKS 上时备份 MySQL 数据,您可以按照以下步骤操作:
-
使用mysqldump进行数据库备份:
使用 Kubernetes cron 作业创建 MySQL 数据库的 mysqldump。
package database import ( "golang-service/models" "golang-service/migrations" "gorm.io/gorm" ) func Migrate(db *gorm.DB) { db.AutoMigrate(&models.User{}) // Apply additional custom migrations if needed }
登录后复制登录后复制登录后复制将其存储在持久卷或 S3 存储桶中。
-
使用 Kubernetes CronJob 实现自动化:
使用 Kubernetes CronJob 自动化 mysqldump 过程。
YAML 配置示例:yaml
package models import "gorm.io/gorm" type User struct { gorm.Model Name string `json:"name"` }
登录后复制登录后复制登录后复制
` 使用 AWS RDS 自动备份(如果使用 RDS):
如果您的 MySQL 数据库位于 AWS RDS 上,您可以利用 RDS 自动备份和快照。
设置备份保留期并手动拍摄快照或使用 Lambda 函数自动拍摄快照。使用 Velero 备份持久卷 (PV):
使用 Kubernetes 备份工具 Velero 来备份保存 MySQL 数据的持久卷。
在您的 EKS 集群上安装 Velero 并将其配置为备份到 S3。
通过使用这些方法,您可以确保您的 MySQL 数据得到定期备份和安全存储。
以上是数据库迁移对于 Golang 服务,为什么重要?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
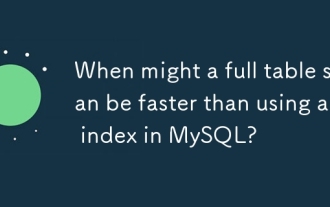
全表扫描在MySQL中可能比使用索引更快,具体情况包括:1)数据量较小时;2)查询返回大量数据时;3)索引列不具备高选择性时;4)复杂查询时。通过分析查询计划、优化索引、避免过度索引和定期维护表,可以在实际应用中做出最优选择。
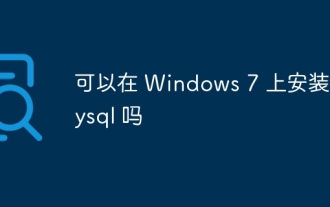
是的,可以在 Windows 7 上安装 MySQL,虽然微软已停止支持 Windows 7,但 MySQL 仍兼容它。不过,安装过程中需要注意以下几点:下载适用于 Windows 的 MySQL 安装程序。选择合适的 MySQL 版本(社区版或企业版)。安装过程中选择适当的安装目录和字符集。设置 root 用户密码,并妥善保管。连接数据库进行测试。注意 Windows 7 上的兼容性问题和安全性问题,建议升级到受支持的操作系统。
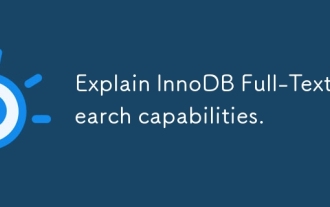
InnoDB的全文搜索功能非常强大,能够显着提高数据库查询效率和处理大量文本数据的能力。 1)InnoDB通过倒排索引实现全文搜索,支持基本和高级搜索查询。 2)使用MATCH和AGAINST关键字进行搜索,支持布尔模式和短语搜索。 3)优化方法包括使用分词技术、定期重建索引和调整缓存大小,以提升性能和准确性。
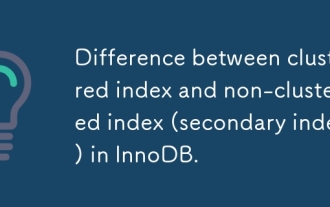
聚集索引和非聚集索引的区别在于:1.聚集索引将数据行存储在索引结构中,适合按主键查询和范围查询。2.非聚集索引存储索引键值和数据行的指针,适用于非主键列查询。
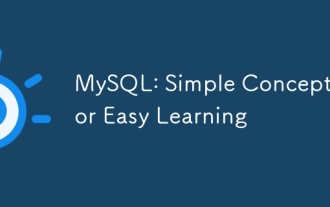
MySQL是一个开源的关系型数据库管理系统。1)创建数据库和表:使用CREATEDATABASE和CREATETABLE命令。2)基本操作:INSERT、UPDATE、DELETE和SELECT。3)高级操作:JOIN、子查询和事务处理。4)调试技巧:检查语法、数据类型和权限。5)优化建议:使用索引、避免SELECT*和使用事务。
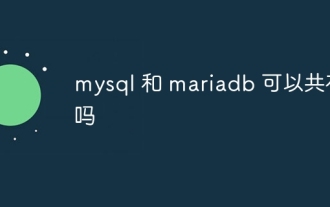
MySQL 和 MariaDB 可以共存,但需要谨慎配置。关键在于为每个数据库分配不同的端口号和数据目录,并调整内存分配和缓存大小等参数。连接池、应用程序配置和版本差异也需要考虑,需要仔细测试和规划以避免陷阱。在资源有限的情况下,同时运行两个数据库可能会导致性能问题。
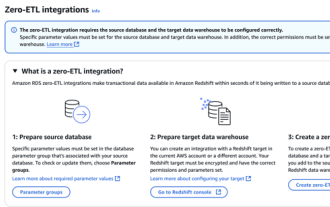
数据集成简化:AmazonRDSMySQL与Redshift的零ETL集成高效的数据集成是数据驱动型组织的核心。传统的ETL(提取、转换、加载)流程复杂且耗时,尤其是在将数据库(例如AmazonRDSMySQL)与数据仓库(例如Redshift)集成时。然而,AWS提供的零ETL集成方案彻底改变了这一现状,为从RDSMySQL到Redshift的数据迁移提供了简化、近乎实时的解决方案。本文将深入探讨RDSMySQL零ETL与Redshift集成,阐述其工作原理以及为数据工程师和开发者带来的优势。
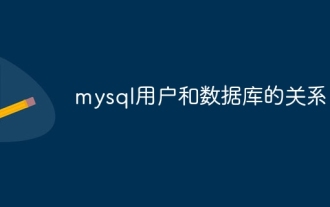
MySQL 数据库中,用户和数据库的关系通过权限和表定义。用户拥有用户名和密码,用于访问数据库。权限通过 GRANT 命令授予,而表由 CREATE TABLE 命令创建。要建立用户和数据库之间的关系,需创建数据库、创建用户,然后授予权限。
