如何在 Java 中高效实现基于网格的像素编辑器,特别是对于大型网格,而不依赖于每个单元格的 JButton?
用 Java 实现基于网格的像素编辑器
为了提高 Spark 编程熟练程度,开发人员经常着手创建像素编辑器等基础应用程序。像素编辑器的核心功能涉及用户选择颜色并修改画布上的网格单元,类似于流行的图像编辑器。
经常出现的一个问题是用于实现这种基于网格的 Java 组件的最佳选择系统。虽然使用 JButton 作为单个单元可能看起来很直观,但它可能变得低效且不切实际,特别是对于较大的网格。
幸运的是,存在更有效的方法。通过利用drawImage()方法并缩放鼠标坐标,开发人员可以创建相当大的像素。
为了演示此技术,请考虑以下示例:
Grid.java
<code class="java">// Import required Java library import java.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Point; import java.awt.event.MouseEvent; import java.awt.event.MouseMotionListener; import java.awt.image.BufferedImage; import javax.swing.Icon; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.UIManager; /** * This class extends JPanel to create a grid-based pixel editor. * @see <a href="https://stackoverflow.com/questions/2900801">Original question</a> */ public class Grid extends JPanel implements MouseMotionListener { // Create buffered image for drawing private final BufferedImage img; // Image and panel dimensions private int imgW, imgH, paneW, paneH; public Grid(String name) { // Initialize basic attributes super(true); // Get the image icon and its dimensions Icon icon = UIManager.getIcon(name); imgW = icon.getIconWidth(); imgH = icon.getIconHeight(); // Set preferred size for the panel this.setPreferredSize(new Dimension(imgW * 10, imgH * 10)); // Create a BufferedImage for the image img = new BufferedImage(imgW, imgH, BufferedImage.TYPE_INT_ARGB); // Get Graphics2D object for drawing Graphics2D g2d = (Graphics2D) img.getGraphics(); // Draw the image icon on the BufferedImage icon.paintIcon(null, g2d, 0, 0); // Dispose the Graphics2D object g2d.dispose(); // Add MouseMotionListener to the panel this.addMouseMotionListener(this); } @Override protected void paintComponent(Graphics g) { // Get current panel dimensions paneW = this.getWidth(); paneH = this.getHeight(); // Draw the image on the panel with scaling g.drawImage(img, 0, 0, paneW, paneH, null); } @Override public void mouseMoved(MouseEvent e) { // Calculate mouse coordinates scaled to image size Point p = e.getPoint(); int x = p.x * imgW / paneW; int y = p.y * imgH / paneH; // Get the pixel color at the calculated scaled coordinates int c = img.getRGB(x, y); // Set tooltip text with color information this.setToolTipText(x + "," + y + ": " + String.format("%08X", c)); } @Override public void mouseDragged(MouseEvent e) { // Mouse drag functionality is not implemented in this example } // Helper method to create the GUI private static void create() { JFrame f = new JFrame(); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new Grid("Tree.closedIcon")); f.pack(); f.setVisible(true); } // Main method to run the application public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { create(); } }); } }</code>
通过利用此技术,开发人员可以轻松创建具有大型可扩展网格的像素编辑器,在保持效率的同时增强用户体验。
以上是如何在 Java 中高效实现基于网格的像素编辑器,特别是对于大型网格,而不依赖于每个单元格的 JButton?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
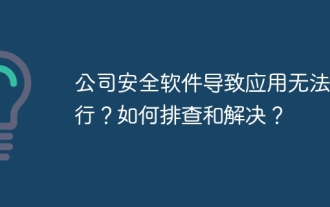
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
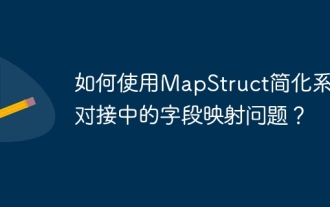
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
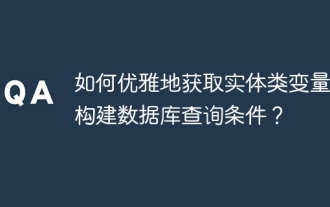
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
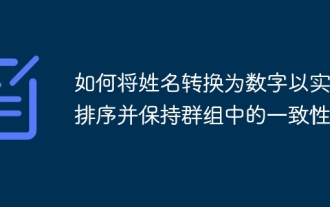
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
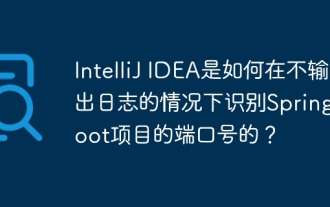
在使用IntelliJIDEAUltimate版本启动Spring...
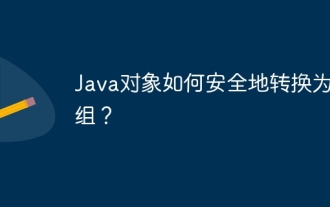
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
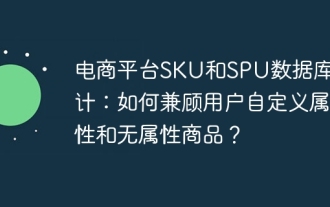
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
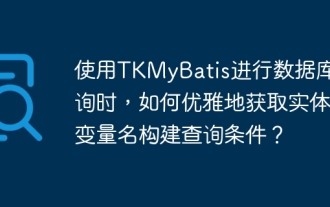
在使用TKMyBatis进行数据库查询时,如何优雅地获取实体类变量名以构建查询条件,是一个常见的难题。本文将针...
