如何使用 Go 中的自定义 UnmarshalJSON 函数将嵌套 JSON 数据展平为单级结构?
展平嵌套 JSON
将嵌套 JSON 数据展平为单级结构是数据处理中的常见任务。以下是如何使用自定义 UnmarshalJSON 函数在 Go 中实现此目的:
UnmarshalJSON 函数
自定义 UnmarshalJSON 函数允许 Go 结构体处理 JSON 数据的解组。这是使用 UnmarshalJSON 实现更新的社交结构:
<code class="go">type Social struct { GooglePlusPlusOnes uint32 `Social:"GooglePlusOne"` TwitterTweets uint32 `json:"Twitter"` LinkedinShares uint32 `json:"LinkedIn"` PinterestPins uint32 `json:"Pinterest"` StumbleuponStumbles uint32 `json:"StumbleUpon"` DeliciousBookmarks uint32 `json:"Delicious"` // Custom unmarshalling for the Facebook fields FacebookLikes uint32 `json:"-"` FacebookShares uint32 `json:"-"` FacebookComments uint32 `json:"-"` FacebookTotal uint32 `json:"-"` } // UnmarshalJSON implements the Unmarshaler interface for custom JSON unmarshalling func (s *Social) UnmarshalJSON(data []byte) error { type FacebookAlias Facebook aux := &struct { Facebook FacebookAlias `json:"Facebook"` }{} if err := json.Unmarshal(data, aux); err != nil { return err } s.FacebookLikes = aux.Facebook.FacebookLikes s.FacebookShares = aux.Facebook.FacebookShares s.FacebookComments = aux.Facebook.FacebookComments s.FacebookTotal = aux.Facebook.FacebookTotal return nil }</code>
用法
使用更新的社交结构,您现在可以解组 JSON 文档并将其展平:
<code class="go">package main import ( "encoding/json" "fmt" ) type Social struct { // ... (same as before) } func (s *Social) UnmarshalJSON(data []byte) error { // ... (same as before) } func main() { var jsonBlob = []byte(`[ {"StumbleUpon":0,"Reddit":0,"Facebook":{"commentsbox_count":4691,"click_count":0,"total_count":298686,"comment_count":38955,"like_count":82902,"share_count":176829},"Delicious":0,"GooglePlusOne":275234,"Buzz":0,"Twitter":7346788,"Diggs":0,"Pinterest":40982,"LinkedIn":0} ]`) var social []Social err := json.Unmarshal(jsonBlob, &social) if err != nil { fmt.Println("error:", err) } fmt.Printf("%+v", social) }</code>
此代码将输出您想要的扁平 JSON 结构,并将 Facebook 字段合并到顶级社交结构中。
以上是如何使用 Go 中的自定义 UnmarshalJSON 函数将嵌套 JSON 数据展平为单级结构?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
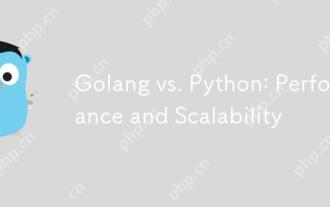
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
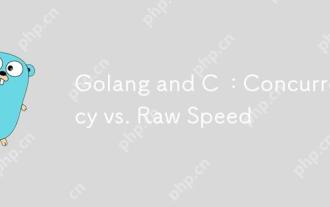
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
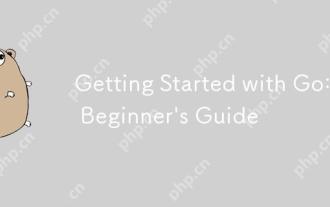
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
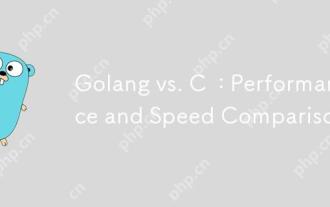
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
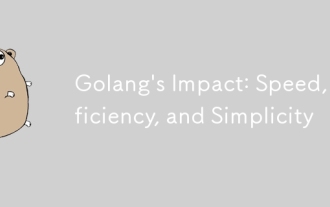
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
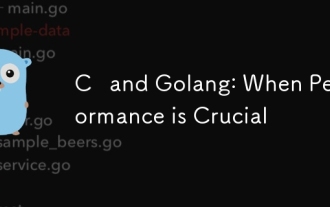
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
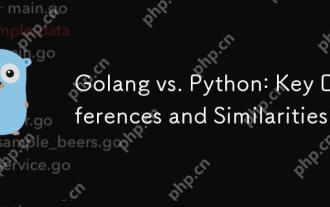
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
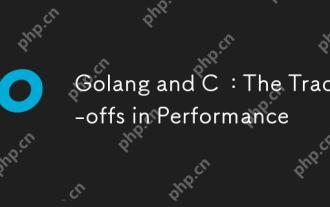
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
