轻松集成 AI:CopilotKit 使用初学者指南
?什么是副驾驶套件?
CopilotKit 是一个开源框架,可以轻松地将强大的、可用于生产的 AI Copilot 集成到任何应用程序中。借助 CopilotKit,您可以无缝实施自定义 AI 聊天机器人、代理、文本区域等来增强您的产品。
?让我们构建一个应用程序,在其中我们将学习如何将 CopilotKit 集成到我们的应用程序中:-
?这个应用程序是关于什么的?
该应用程序使用 CopilotKit 自动生成抽认卡和测验。只需要求人工智能聊天机器人创建任何主题的抽认卡,它就会立即生成抽认卡和相应的测验。这是学习任何学科的快速有效的方式。
?技术堆栈:
前端:NextJs、Tailwind CSS、shadcdn、Zustand
后端:下一个Js
数据存储:本地存储
?设置
- 继续并安装这些依赖项:
npm install @copilotkit/react-core @copilotkit/react-ui @copilotkit/runtime
- 在应用程序的根级别创建一个 .evn 文件并将以下变量添加到其中:
GROQ_API_KEY=<your_groq_api_key>
?要获取您的 Groq API 密钥,请按照以下步骤操作:
转到 GroqCloud 并通过单击“创建 API 密钥”按钮生成 API 密钥。
?让我们深入了解开发:
后端:对于后端,我们将设置一个 /api/copilotkit 端点。此端点将处理来自前端的请求,提供数据或根据需要进行响应。这个端点是您使用 CopilotKit 为应用程序提供支持所需的全部内容。
import { CopilotRuntime, GroqAdapter, copilotRuntimeNextJSAppRouterEndpoint, } from "@copilotkit/runtime"; import { NextRequest } from "next/server"; import Groq from "groq-sdk"; const groq:Groq = new Groq({ apiKey: process.env.GROQ_API_KEY }) ; const copilotKit = new CopilotRuntime(); const serviceAdapter = new GroqAdapter({ groq, model: "llama3-groq-8b-8192-tool-use-preview" }); export const POST = async (req: NextRequest) => { const { handleRequest } = copilotRuntimeNextJSAppRouterEndpoint({ runtime: copilotKit, serviceAdapter, endpoint: "/api/copilotkit", }); return handleRequest(req); };
前端:
现在,让我们将 CopilotKit 集成到我们的应用程序中。 CopilotKit 提供了几个有用的钩子,在本教程中,我们将重点关注两个重要的钩子:
- useCopilotReadable: useCopilotReadable 挂钩是一个 React 挂钩,为 Copilot 提供应用程序状态和其他相关信息。此外,此挂钩可以管理应用程序内的分层状态,允许您根据需要将父子关系传递给 Copilot。
npm install @copilotkit/react-core @copilotkit/react-ui @copilotkit/runtime
- useCopilotAction: useCopilotAction 挂钩是一个 React 挂钩,使您的副驾驶能够在应用程序中执行操作。您可以使用此挂钩来定义可由应用程序中的 AI 触发的自定义操作。
GROQ_API_KEY=<your_groq_api_key>
- 要实现聊天机器人,您可以使用 @copilotkit/react-ui 包中的 CopilotSidebar 组件。以下是如何进行:
import { CopilotRuntime, GroqAdapter, copilotRuntimeNextJSAppRouterEndpoint, } from "@copilotkit/runtime"; import { NextRequest } from "next/server"; import Groq from "groq-sdk"; const groq:Groq = new Groq({ apiKey: process.env.GROQ_API_KEY }) ; const copilotKit = new CopilotRuntime(); const serviceAdapter = new GroqAdapter({ groq, model: "llama3-groq-8b-8192-tool-use-preview" }); export const POST = async (req: NextRequest) => { const { handleRequest } = copilotRuntimeNextJSAppRouterEndpoint({ runtime: copilotKit, serviceAdapter, endpoint: "/api/copilotkit", }); return handleRequest(req); };
- 将所有这些组件放在一起,完整的文件如下所示:
useCopilotReadable({ description: 'A code snippet manager', value: flashcards, });
- 此外,我们需要一个状态管理库来确保每当人工智能采取行动时我们的 UI 都会更新。您可以选择任何状态管理库,但在本教程中,我将使用 Zustand 和本地存储一起进行数据存储。这将充当应用程序状态的全局管理点。
useCopilotAction({ name: "create-flashcards-and-also-quiz-questions-for-those-flashcards", description: `Create a new flashcard along with corresponding quiz questions. Each flashcard should contain a term, description, topic, and relevant tags. Additionally, for each flashcard, generate quiz questions with multiple answer options. The quiz questions should conform to the 'QuizQuestion' interface, where: - Each question contains a string 'question', an array of four 'options', and the 'correctOption' corresponding to the correct answer. `, parameters: [ { name: "flashcards", description: "The flashcards for the given topic", type: "object[]", // Use "array" as the type }, { name: "quiz", description: "The quiz questions for the given topic, adhering to the QuizQuestion interface", type: "object[]", // Use "array" for QuizQuestion[] }, { name:"topic", description: "The title of the topic", type: "string" } ], handler: (args: { flashcards: Flashcard[], quiz: QuizQuestion[], topic: string }) => { addTopics(args); }, });
最终应用截图:
这是我引用的项目:
https://github.com/Niharika0104/learn-using-flash-cards
这里是该项目的现场演示:
https://learn-using-flash-cards.vercel.app/
我希望您喜欢这个关于 CopilotKit 的简短教程。请继续关注更多此类有趣且简洁的教程!
希望在下一场见到大家
尼哈里卡。
以上是轻松集成 AI:CopilotKit 使用初学者指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
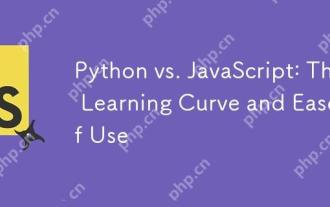
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
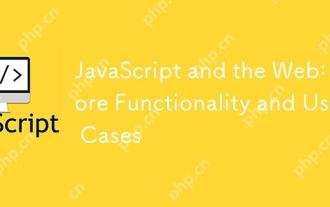
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
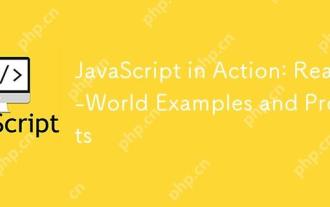
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
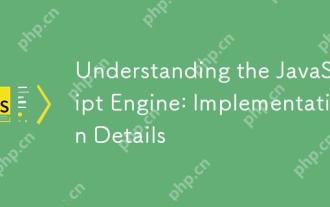
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
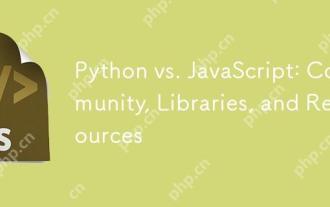
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
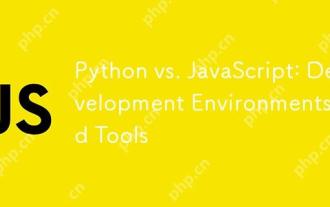
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
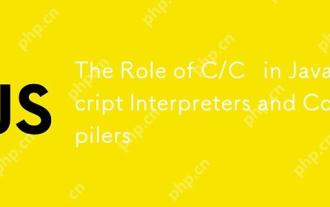
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
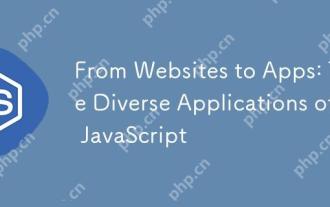
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
