如何使用 Python 的 `json.JSONDecoder.raw_decode` 高效地从单个文件中提取多个 JSON 对象?
从单个文件中迭代提取多个 JSON 对象
处理包含多个 JSON 对象的 JSON 文件时,找到一种有效的方法至关重要从每个对象中提取特定的数据元素。
一种方法是利用 Python 的 json.JSONDecoder.raw_decode 函数。此函数允许您解码包含多个对象的大型 JSON 字符串,即使它们没有包装在根数组中。
首先,您需要从 JSON 文档中删除所有前导空格。之后,您可以在循环中使用 raw_decode 来逐个提取对象。该函数返回解析对象结束的最后位置以及对象本身。
这是演示此方法的代码片段:
<code class="python">from json import JSONDecoder, JSONDecodeError import re NOT_WHITESPACE = re.compile(r'\S') def decode_stacked(document, pos=0, decoder=JSONDecoder()): while True: match = NOT_WHITESPACE.search(document, pos) if not match: return pos = match.start() try: obj, pos = decoder.raw_decode(document, pos) except JSONDecodeError: # handle error raise yield obj</code>
使用此方法,您可以解码 JSON 字符串具有多个对象并将特定元素提取到数据框中。例如,如果您的 JSON 文件包含以下结构:
<code class="json">{"ID":"12345","Timestamp":"20140101", "Usefulness":"Yes", "Code":[{"event1":"A","result":"1"},…]} {"ID":"1A35B","Timestamp":"20140102", "Usefulness":"No", "Code":[{"event1":"B","result":"1"},…]} {"ID":"AA356","Timestamp":"20140103", "Usefulness":"No", "Code":[{"event1":"B","result":"0"},…]} …</code>
您的代码可以使用以下循环来提取“时间戳”和“有用性”值:
<code class="python">import pandas as pd data = [] for obj in decode_stacked(json_string): data.append([obj["Timestamp"], obj["Usefulness"]]) df = pd.DataFrame(data, columns=["Timestamp", "Usefulness"])</code>
This方法提供了一种灵活有效的方法来从单个文件中提取多个 JSON 对象,允许您将复杂 JSON 结构中的数据收集为表格格式。
以上是如何使用 Python 的 `json.JSONDecoder.raw_decode` 高效地从单个文件中提取多个 JSON 对象?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
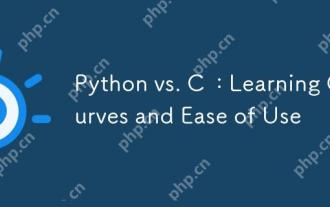
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
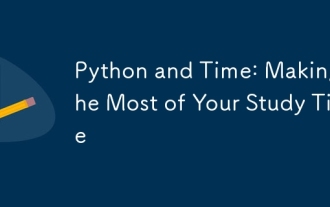
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
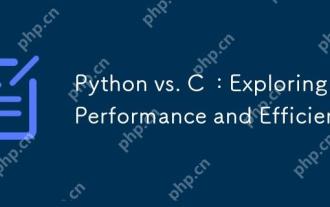
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
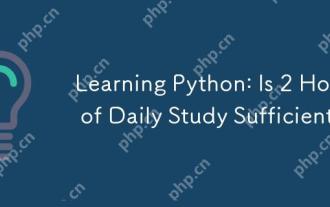
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
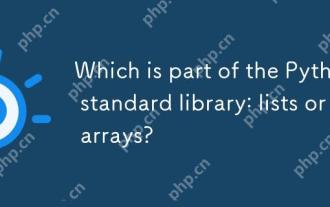
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
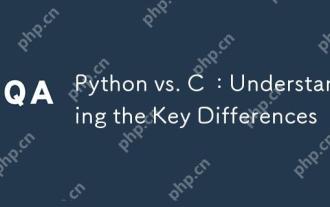
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
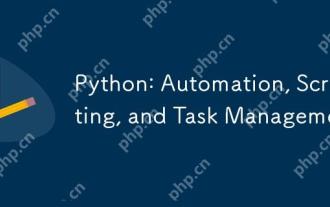
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
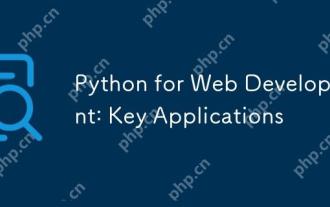
Python在Web开发中的关键应用包括使用Django和Flask框架、API开发、数据分析与可视化、机器学习与AI、以及性能优化。1.Django和Flask框架:Django适合快速开发复杂应用,Flask适用于小型或高度自定义项目。2.API开发:使用Flask或DjangoRESTFramework构建RESTfulAPI。3.数据分析与可视化:利用Python处理数据并通过Web界面展示。4.机器学习与AI:Python用于构建智能Web应用。5.性能优化:通过异步编程、缓存和代码优
