如何使用 FastAPI 发布数据后下载文件?
如何使用 FastAPI 在发布数据后下载文件?
使用 FastAPI 和文件处理时,一项常见任务是让用户在提交后下载文件通过 POST 请求获取数据。这可以通过利用 FileResponse 类并确保标头和内容类型的正确配置来实现。
在下面提供的代码中,我们将演示如何设置处理数据并返回音频的 POST 端点文件(MP3)供下载。我们将使用表单数据类型来捕获用户输入并生成音频文件。
选项 1:使用单独的下载端点
在此方法中,我们将为处理文件下载。
<code class="python">from fastapi import FastAPI, Request, Form, File, UploadFile from fastapi.responses import FileResponse, HTMLResponse from fastapi.templating import Jinja2Templates from gtts import gTTS app = FastAPI() templates = Jinja2Templates(directory="templates") def text_to_speech(language: str, text: str) -> str: tts = gTTS(text=text, lang=language, slow=False) filepath = "temp/welcome.mp3" tts.save(filepath) return f"Text to speech conversion successful to {filepath}" @app.get("/") def home(request: Request): return templates.TemplateResponse("index.html", {"request": request}) @app.post("/text2speech") def text2speech(request: Request, message: str = Form(...), language: str = Form(...)): if message and language: output = text_to_speech(language, message) path = "temp/welcome.mp3" filename = os.path.basename(path) headers = {"Content-Disposition": f"attachment; filename={filename}"} return FileResponse(path, headers=headers, media_type="audio/mp3")</code>
GET 端点用作主页,而 POST 端点处理用户输入并生成音频文件。 FileResponse 负责为文件下载设置适当的标头。
在模板中,您可以添加一个链接来触发文件下载:
<code class="html"><!DOCTYPE html> <html> <head> <title>Download File</title> </head> <body> <a href="{{ url_for('text2speech') }}">Download File</a> </body> </html></code>
选项 2:端点内文件下载
或者,您可以在处理数据的同一端点内处理文件下载:
<code class="python">from fastapi import FastAPI, Request, Form app = FastAPI() def text_to_speech(language: str, text: str) -> str: tts = gTTS(text=text, lang=language, slow=False) filepath = "temp/welcome.mp3" tts.save(filepath) return f"Text to speech conversion successful to {filepath}" @app.post("/text2speech") def text2speech(request: Request, message: str = Form(...), language: str = Form(...)): output = text_to_speech(language, message) return {"filepath": filepath}</code>
在模板中,您可以使用 JavaScript 使用从返回的文件路径触发文件下载端点:
<code class="html"><script> const filepath = "{{ filepath }}"; window.location.href = filepath; </script></code>
处理大文件
对于超出内存限制的文件,可以使用 StreamingResponse:
<code class="python">from fastapi.responses import StreamingResponse @app.post("/text2speech") def text2speech(request: Request, message: str = Form(...), language: str = Form(...)): ... def iterfile(): with open(filepath, "rb") as f: yield from f headers = {"Content-Disposition": f"attachment; filename={filename}"} return StreamingResponse(iterfile(), headers=headers, media_type="audio/mp3")</code>
下载后删除文件
为了防止磁盘空间累积,最好在下载文件后将其删除。使用BackgroundTasks,您可以将文件删除安排为后台任务:
<code class="python">from fastapi import BackgroundTasks @app.post("/text2speech") def text2speech(request: Request, background_tasks: BackgroundTasks, ...): ... background_tasks.add_task(os.remove, path=filepath) return FileResponse(filepath, headers=headers, media_type="audio/mp3")</code>
以上是如何使用 FastAPI 发布数据后下载文件?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
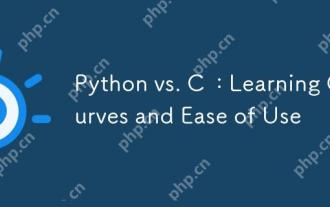
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
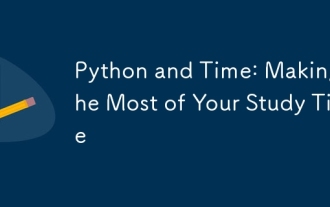
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
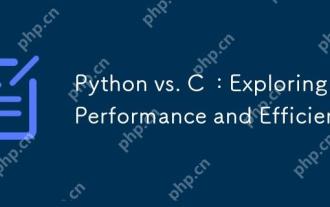
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
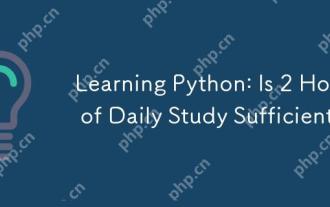
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
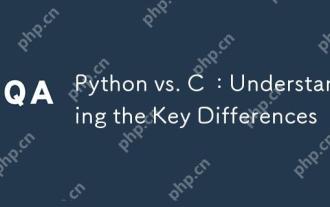
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
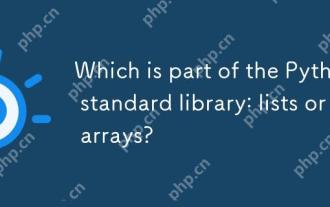
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
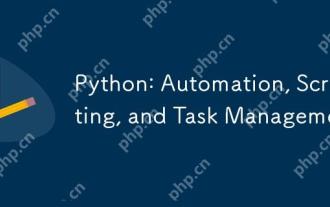
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
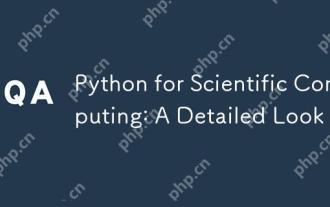
Python在科学计算中的应用包括数据分析、机器学习、数值模拟和可视化。1.Numpy提供高效的多维数组和数学函数。2.SciPy扩展Numpy功能,提供优化和线性代数工具。3.Pandas用于数据处理和分析。4.Matplotlib用于生成各种图表和可视化结果。
