如何在 AngularJS 中动态添加指令而不出现无限循环?
使用 AngularJS 动态添加指令
在 AngularJS 中构建复杂的 UI 元素时,可能需要动态地将指令添加到 DOM 元素。实现此目的的一种方法是创建一个管理多个指令添加的高阶指令。
使用条件编译实现
一种方法是检查是否在尝试添加所需的指令之前已经添加了它们。此方法涉及在指令的链接函数中设置条件检查:
<code class="javascript">angular.module('app') .directive('superDirective', function ($compile, $injector) { return { restrict: 'A', replace: true, link: function (scope, element, attrs) { if (element.attr('datepicker')) { // Check for existing directive return; } element.attr('datepicker', 'someValue'); // Add directive attribute // Add other directive attributes $compile(element)(scope); } }; });</code>
但是,如果未从元素中正确删除添加的属性,此方法可能会导致无限循环。
优先级和终端指令的解决方案
为了避免无限循环并确保正确的指令执行,优先级的组合可以使用terminal属性:
<code class="javascript">angular.module('app') .directive('commonThings', function ($compile) { return { restrict: 'A', replace: false, terminal: true, // Skips compilation of subsequent directives on this element priority: 1000, // Executes before other directives link: function (scope, element, attrs) { // Add tooltip and tooltip placement directives element.attr('tooltip', '{{dt()}}'); element.attr('tooltip-placement', 'bottom'); // Remove the directive attribute to prevent recursive compilation element.removeAttr("common-things"); element.removeAttr("data-common-things"); // Compile the element again, including the added directives $compile(element)(scope); } }; });</code>
将terminal设置为true可以防止在同一元素上编译后续指令。高优先级可确保自定义指令先于其他指令编译。之后删除指令属性可以防止无限循环。这种方法可确保添加的指令正确执行,而不会造成任何干扰。
以上是如何在 AngularJS 中动态添加指令而不出现无限循环?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
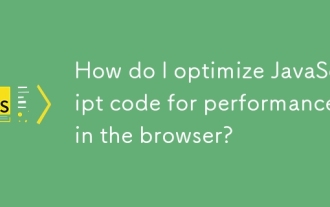
本文讨论了在浏览器中优化JavaScript性能的策略,重点是减少执行时间并最大程度地减少对页面负载速度的影响。
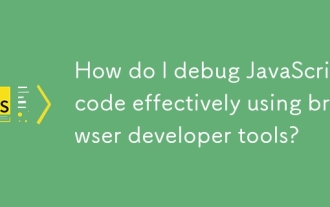
本文讨论了使用浏览器开发人员工具的有效JavaScript调试,专注于设置断点,使用控制台和分析性能。
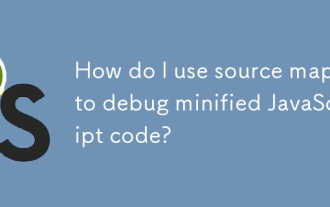
本文说明了如何使用源地图通过将其映射回原始代码来调试JAVASCRIPT。它讨论了启用源地图,设置断点以及使用Chrome DevTools和WebPack之类的工具。
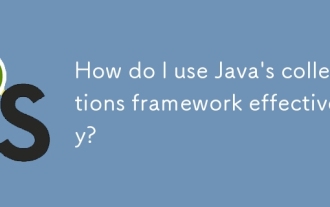
本文探讨了Java收藏框架的有效使用。 它强调根据数据结构,性能需求和线程安全选择适当的收集(列表,设置,地图,队列)。 通过高效优化收集用法
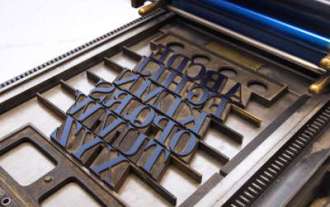
掌握了入门级TypeScript教程后,您应该能够在支持TypeScript的IDE中编写自己的代码,并将其编译成JavaScript。本教程将深入探讨TypeScript中各种数据类型。 JavaScript拥有七种数据类型:Null、Undefined、Boolean、Number、String、Symbol(ES6引入)和Object。TypeScript在此基础上定义了更多类型,本教程将详细介绍所有这些类型。 Null数据类型 与JavaScript一样,TypeScript中的null

本教程将介绍如何使用 Chart.js 创建饼图、环形图和气泡图。此前,我们已学习了 Chart.js 的四种图表类型:折线图和条形图(教程二),以及雷达图和极地区域图(教程三)。 创建饼图和环形图 饼图和环形图非常适合展示某个整体被划分为不同部分的比例。例如,可以使用饼图展示野生动物园中雄狮、雌狮和幼狮的百分比,或不同候选人在选举中获得的投票百分比。 饼图仅适用于比较单个参数或数据集。需要注意的是,饼图无法绘制值为零的实体,因为饼图中扇形的角度取决于数据点的数值大小。这意味着任何占比为零的实体
