如何在 Go 中提取分隔符之间的子字符串?
在 Go 中提取分隔符之间的字符串
在 Go 中,你可能会遇到需要从较大字符串中提取特定子字符串的情况,基于已知的分隔符或特定字符。
考虑以下字符串:
<h1>Hello World!</h1>
提取“Hello World!”使用 Go 从此字符串中,您可以采用以下技术:
package main
import (
"fmt"
"strings"
)
func main() {
str := "<h1>Hello World!</h1>"
// Find the index of the starting delimiter
startIndex := strings.Index(str, "<h1>")
// If the delimiter is not found, return an empty string
if startIndex == -1 {
fmt.Println("No starting delimiter found")
return
}
// Adjust the starting index to omit the delimiter
startIndex += len("<h1>")
// Find the index of the ending delimiter
endIndex := strings.Index(str, "</h1>")
// If the delimiter is not found, return an empty string
if endIndex == -1 {
fmt.Println("No ending delimiter found")
return
}
// Extract the substring between the delimiters
result := str[startIndex:endIndex]
// Print the extracted string
fmt.Println(result)
}
此代码查找输入字符串中开始和结束分隔符的索引。然后,它调整起始索引以考虑分隔符并提取分隔符之间的子字符串。然后将提取的子字符串打印到控制台。
一般情况下,您可以修改代码中提供的分隔符字符串,以从任何字符串中提取特定的子字符串。
以上是如何在 Go 中提取分隔符之间的子字符串?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
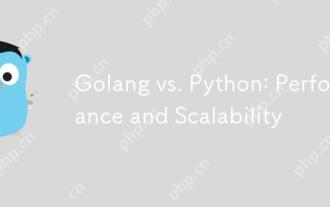
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
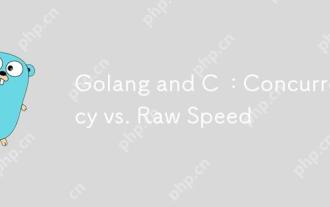
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
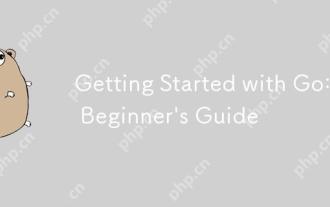
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
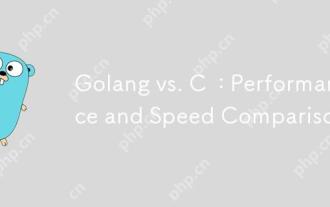
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
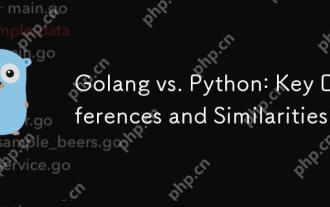
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
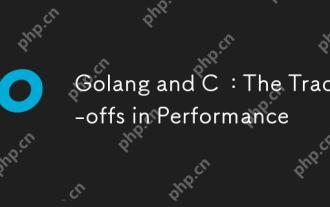
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
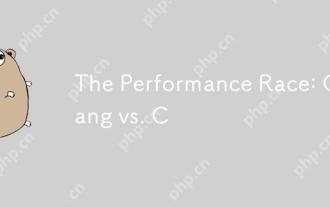
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
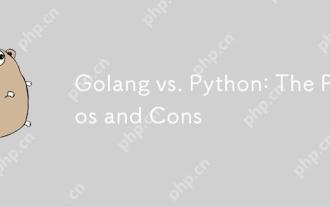
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
