如何使用Go通道来实现观察者模式?
Go 语言中的观察者模式
在很多编程场景中,都需要一个对象在事件发生时通知多个订阅者。这种模式通常称为观察者模式。在 Go 中,通道为实现此模式提供了一个优雅的解决方案。
下面的代码示例演示了一个工作示例:
<code class="go">type Publisher struct { listeners []chan *Msg } type Subscriber struct { Channel chan *Msg } func (p *Publisher) Sub(c chan *Msg) { p.appendListener(c) } func (p *Publisher) Pub(m *Msg) { for _, c := range p.listeners { c <- Msg } } func (s *Subscriber) ListenOnChannel() { for { data := <-s.Channel // Process data } } func main() { publisher := &Publisher{} subscribers := []*Subscriber{ &Subscriber{make(chan *Msg)}, &Subscriber{make(chan *Msg)}, // Additional subscribers can be added here } for _, sub := range subscribers { publisher.Sub(sub.Channel) go sub.ListenOnChannel() } publisher.Pub(&Msg{"Event Notification"}) // Pause the main function to allow subscribers to process messages time.Sleep(time.Second) } type Msg struct { Message string }</code>
在此示例中,发布者持有一段侦听器通道,代表订阅的对象。 Pub 方法通过将数据发送到所有侦听器的通道来通知它们。每个订阅者在其专用通道上连续监听传入的数据以进行处理。
以上是如何使用Go通道来实现观察者模式?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
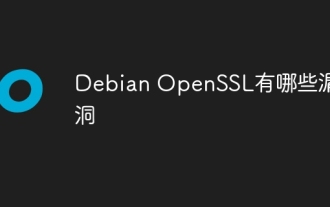
OpenSSL,作为广泛应用于安全通信的开源库,提供了加密算法、密钥和证书管理等功能。然而,其历史版本中存在一些已知安全漏洞,其中一些危害极大。本文将重点介绍Debian系统中OpenSSL的常见漏洞及应对措施。DebianOpenSSL已知漏洞:OpenSSL曾出现过多个严重漏洞,例如:心脏出血漏洞(CVE-2014-0160):该漏洞影响OpenSSL1.0.1至1.0.1f以及1.0.2至1.0.2beta版本。攻击者可利用此漏洞未经授权读取服务器上的敏感信息,包括加密密钥等。
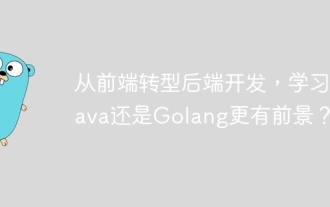
后端学习路径:从前端转型到后端的探索之旅作为一名从前端开发转型的后端初学者,你已经有了nodejs的基础,...
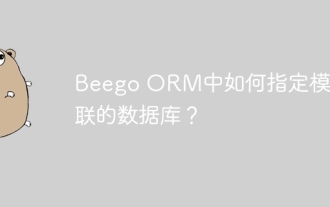
在BeegoORM框架下,如何指定模型关联的数据库?许多Beego项目需要同时操作多个数据库。当使用Beego...
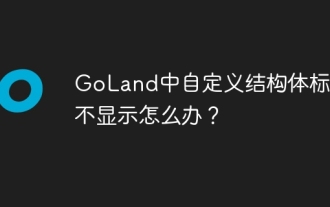
GoLand中自定义结构体标签不显示怎么办?在使用GoLand进行Go语言开发时,很多开发者会遇到自定义结构体标签在�...
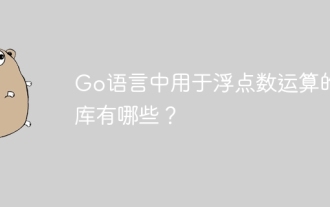
Go语言中用于浮点数运算的库介绍在Go语言(也称为Golang)中,进行浮点数的加减乘除运算时,如何确保精度是�...
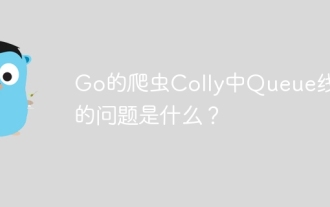
Go爬虫Colly中的Queue线程问题探讨在使用Go语言的Colly爬虫库时,开发者常常会遇到关于线程和请求队列的问题。�...
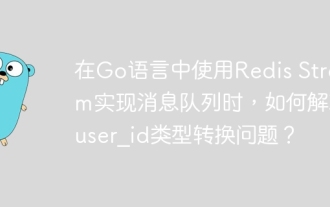
Go语言中使用RedisStream实现消息队列时类型转换问题在使用Go语言与Redis...

本文介绍如何在Debian系统上配置MongoDB实现自动扩容,主要步骤包括MongoDB副本集的设置和磁盘空间监控。一、MongoDB安装首先,确保已在Debian系统上安装MongoDB。使用以下命令安装:sudoaptupdatesudoaptinstall-ymongodb-org二、配置MongoDB副本集MongoDB副本集确保高可用性和数据冗余,是实现自动扩容的基础。启动MongoDB服务:sudosystemctlstartmongodsudosys
