JavaScript 和 React Native 中的 Promise:创建、使用和常见场景
处理异步任务在 JavaScript 中至关重要,尤其是在像 React Native 这样的环境中,数据获取、动画和用户交互需要无缝工作。 Promise 提供了一种管理异步操作的强大方法,使代码更具可读性和可维护性。本博客将介绍如何在 JavaScript 中创建和使用 Promise,以及与 React Native 相关的实际示例。
什么是承诺?
JavaScript 中的Promise 是一个表示异步操作最终完成(或失败)的对象。它允许我们以更同步的方式处理异步代码,避免经典的“回调地狱”。 Promise 具有三种状态:
- 待处理:初始状态,既不满足也不拒绝。
- 已完成:操作成功完成。
- 已拒绝:操作失败。
创造一个承诺
为了创建 Promise,我们使用 Promise 构造函数,它采用带有两个参数的单个函数(执行器函数):
-
解决:当操作成功完成时,调用此函数来履行承诺。
- 拒绝:如果发生错误,调用此函数拒绝承诺。
function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { const success = true; // Simulating success/failure if (success) { resolve({ data: "Sample data fetched" }); } else { reject("Error: Data could not be fetched."); } }, 2000); // Simulate a 2-second delay }); }
- 我们创建一个 Promise,通过 setTimeout 模拟获取数据。
- 如果 success 变量为 true,我们将使用一些数据调用resolve();否则,我们调用reject()并显示错误消息。
将 Promise 与 .then()、.catch() 和 .finally() 一起使用
一旦创建了 Promise,我们就可以使用以下方法处理其结果:
-
.then() 处理成功的解决方案,
- .catch() 处理错误,以及
- .finally() 在 Promise 解决后执行代码,无论结果如何。
fetchData() .then((result) => console.log("Data:", result.data)) // Handle success .catch((error) => console.error("Error:", error)) // Handle failure .finally(() => console.log("Fetch attempt completed")); // Finalize
-
如果 Promise 得到解决,则调用 .then() 并打印数据。
- .catch() 处理承诺被拒绝时发生的任何错误。
- .finally() 无论 Promise 是否已解决或拒绝都会运行。
React Native 中 Promise 的实际用例
1. 从 API 获取数据
在 React Native 中,数据获取是一种常见的异步任务,可以通过 Promise 进行有效管理。
function fetchData(url) { return fetch(url) .then(response => { if (!response.ok) throw new Error("Network response was not ok"); return response.json(); }) .then(data => console.log("Fetched data:", data)) .catch(error => console.error("Fetch error:", error)); } // Usage fetchData("https://api.example.com/data");
用例:从 REST API 或其他网络请求获取数据,我们需要处理成功的响应和错误。
2. 使用 Promise 进行顺序异步操作
有时,一个异步任务依赖于另一个异步任务。 Promise 使得按顺序链接操作变得容易。
function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { const success = true; // Simulating success/failure if (success) { resolve({ data: "Sample data fetched" }); } else { reject("Error: Data could not be fetched."); } }, 2000); // Simulate a 2-second delay }); }
用例:用于登录用户,然后根据其身份获取个人资料数据。
3. 使用 Promise.all() 处理多个 Promise
如果您有多个可以并行执行的独立 Promise,Promise.all() 允许您等待所有这些 Promise 得到解决或其中任何一个被拒绝。
fetchData() .then((result) => console.log("Data:", result.data)) // Handle success .catch((error) => console.error("Error:", error)) // Handle failure .finally(() => console.log("Fetch attempt completed")); // Finalize
用例:同时获取多个资源,例如从单独的 API 端点获取帖子和评论。
4. 使用 Promise.race() 竞赛 Promise
使用 Promise.race(),第一个解决(解决或拒绝)的 Promise 决定结果。当您想要为长时间运行的任务设置超时时,这非常有用。
function fetchData(url) { return fetch(url) .then(response => { if (!response.ok) throw new Error("Network response was not ok"); return response.json(); }) .then(data => console.log("Fetched data:", data)) .catch(error => console.error("Fetch error:", error)); } // Usage fetchData("https://api.example.com/data");
用例:为网络请求设置超时,这样如果服务器缓慢或无响应,它们就不会无限期挂起。
5. 使用 Promise.allSettled() 处理混合结果
Promise.allSettled() 等待所有 Promise 解决,无论它们是解决还是拒绝。当您需要所有承诺的结果时(即使有些承诺失败),这很有用。
function authenticateUser() { return new Promise((resolve) => { setTimeout(() => resolve({ userId: 1, name: "John Doe" }), 1000); }); } function fetchUserProfile(user) { return new Promise((resolve) => { setTimeout(() => resolve({ ...user, profile: "Profile data" }), 1000); }); } // Chain promises authenticateUser() .then(user => fetchUserProfile(user)) .then(profile => console.log("User Profile:", profile)) .catch(error => console.error("Error:", error));
用例:在执行多个请求(其中某些请求可能会失败)时很有用,例如获取可选数据源或进行多个 API 调用。
高级技术:将回调转换为 Promise
较旧的代码库或某些库可能使用回调而不是承诺。您可以将这些回调包装在 Promise 中,将它们转换为现代的基于 Promise 的函数。
示例:将回调包装在 Promise 中
const fetchPosts = fetch("https://api.example.com/posts").then(res => res.json()); const fetchComments = fetch("https://api.example.com/comments").then(res => res.json()); Promise.all([fetchPosts, fetchComments]) .then(([posts, comments]) => { console.log("Posts:", posts); console.log("Comments:", comments); }) .catch(error => console.error("Error fetching data:", error));
用例:此技术允许您以承诺友好的方式使用基于回调的遗留代码,使其与现代异步/等待语法兼容。
概括
Promise 是管理 JavaScript 和 React Native 中异步操作的强大工具。通过了解如何在各种场景中创建、使用和处理 Promise,您可以编写更清晰、更易于维护的代码。以下是常见用例的快速回顾:
- API 请求:从服务器获取数据并进行错误处理。
- 连锁操作:按顺序执行依赖任务。
- 并行操作:使用 Promise.all() 同时运行多个 Promise。
- 超时和竞赛:使用 Promise.race() 限制请求持续时间。
- 混合结果:使用 Promise.allSettled() 处理可能部分失败的任务。
- 转换回调:将基于回调的函数包装在 Promise 中以与现代语法兼容。
通过有效地利用 Promise,您可以使 JavaScript 和 React Native 中的异步编程更干净、更可预测且更健壮。
以上是JavaScript 和 React Native 中的 Promise:创建、使用和常见场景的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
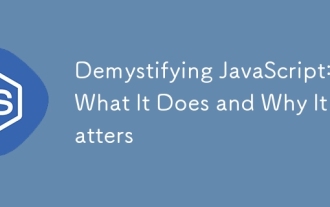
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
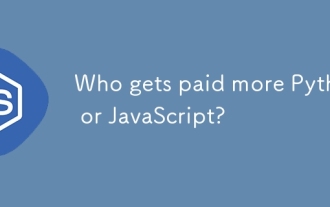
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
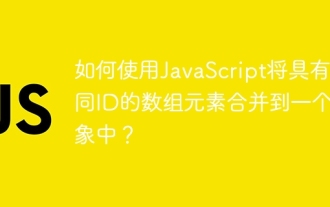
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
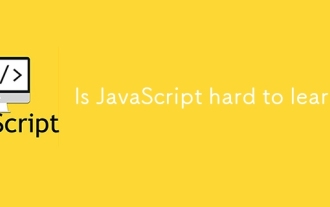
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
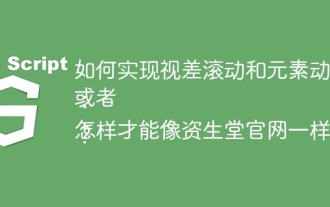
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
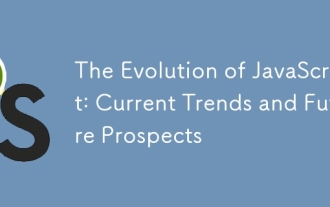
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
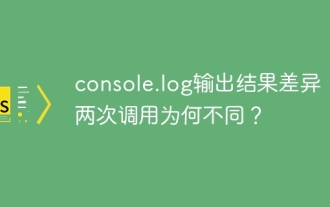
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
