我什么时候应该选择 C 中的引用和指针?
何时在 C 中使用引用和指针
在 C 中通过引用或指针传递数据会带来一个常见的困境。为了清楚起见,让我们深入研究每个选项:
参考文献
- 优点:参考文献提供了路过的易用性-值语义,同时保持引用传递的效率。不能重新赋值,保证函数执行过程中的稳定性。
- 缺点:引用不能设置为空,不方便表示缺失的值或传递函数参数默认值。
指针
- 优点:指针允许按引用传递或按值传递,具体取决于关于具体的需要。它们提供了取消引用以访问底层值的灵活性。
- 缺点:指针可以指向 null,需要仔细处理和检查有效性。它们还引入了复杂的语法,尤其是在使用指针算术时。
一般准则
作为一般经验法则,“当可以时使用引用,并且必要时给予指点。”以下是常见场景的细分:
-
通过引用传递:
- 传递内置类型(int、double 等) )
- 传递类对象(如果复制是昂贵)
- 传递shared_ptr对象(以避免不必要的复制)
-
通过指针传递:
- 当需要传递空值时
- 当需要修改对象的地址(例如,用于动态内存分配)
- 当您需要将指针传递给成员函数时(需要特殊语法)
具体示例
提供的代码片段演示了引用和指针:
map<string, shared_ptr<vector<string>> > adjacencyMap; vector<string>* myFriends = new vector<string>(); myFriends->push_back(string("a")); myFriends->push_back(string("v")); myFriends->push_back(string("g")); adjacencyMap["s"] = shared_ptr<vector<string>>(myFriends);
在这种情况下,使用向量的引用 (myFriends) 允许直接操作,而无需复制开销。然而,由于 myFriends 是动态分配的,因此它是通过指针访问的,避免了悬空指针问题。
请记住,引用和指针之间的选择取决于情况的具体要求。通过了解每种方法的优点和缺点,您可以做出明智的决策,优化代码性能和清晰度。
以上是我什么时候应该选择 C 中的引用和指针?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
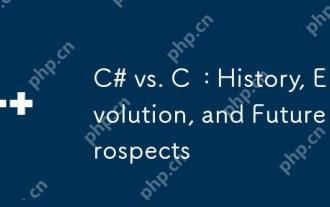
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
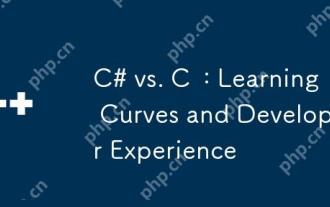
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
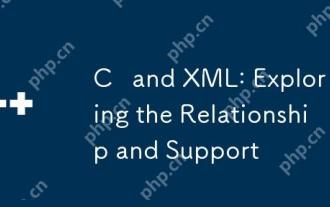
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
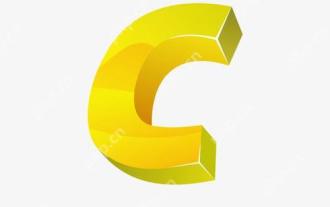
静态分析在C 中的应用主要包括发现内存管理问题、检查代码逻辑错误和提高代码安全性。1)静态分析可以识别内存泄漏、双重释放和未初始化指针等问题。2)它能检测未使用变量、死代码和逻辑矛盾。3)静态分析工具如Coverity能发现缓冲区溢出、整数溢出和不安全API调用,提升代码安全性。
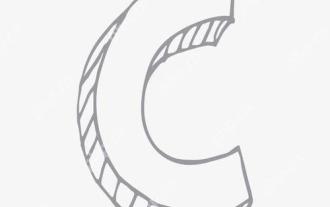
使用C 中的chrono库可以让你更加精确地控制时间和时间间隔,让我们来探讨一下这个库的魅力所在吧。C 的chrono库是标准库的一部分,它提供了一种现代化的方式来处理时间和时间间隔。对于那些曾经饱受time.h和ctime折磨的程序员来说,chrono无疑是一个福音。它不仅提高了代码的可读性和可维护性,还提供了更高的精度和灵活性。让我们从基础开始,chrono库主要包括以下几个关键组件:std::chrono::system_clock:表示系统时钟,用于获取当前时间。std::chron
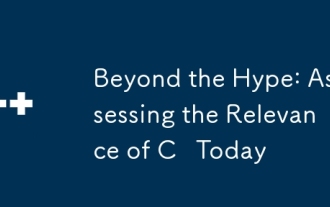
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
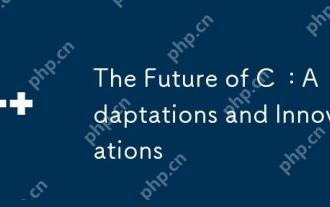
C 的未来将专注于并行计算、安全性、模块化和AI/机器学习领域:1)并行计算将通过协程等特性得到增强;2)安全性将通过更严格的类型检查和内存管理机制提升;3)模块化将简化代码组织和编译;4)AI和机器学习将促使C 适应新需求,如数值计算和GPU编程支持。
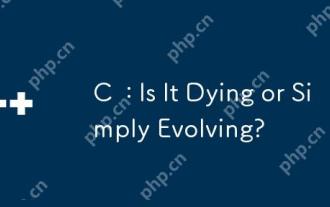
1)c relevantduetoItsAverity and效率和效果临界。2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
