如何使用自定义 URL 协议从 Java 类路径加载资源?
Java 中从类路径加载资源的 URL
在 Java 中,可以使用类似的 API 但使用不同的 URL 协议来加载各种资源。这样可以将资源加载过程与应用程序解耦,并简化资源配置。
是否可以利用当前类加载器的协议来获取资源,类似于 Jar 协议,但不指定原始文件或文件夹?
实现
这可以通过实现自定义 URLStreamHandler 和将其注册到 JVM。
基本实现
- 创建 URLStreamHandler: 此处理程序允许使用给定 URL 打开连接。它应该扩展 URLStreamHandler 类并实现 openConnection 方法。实现应该使用提供的类加载器来定位资源并打开到它的连接。
- 代码:以下 Java 代码提供了自定义处理程序实现的示例:
import java.io.IOException; import java.net.URL; import java.net.URLConnection; import java.net.URLStreamHandler; import java.net.URLStreamHandlerFactory; import java.util.HashMap; import java.util.Map; public class ClasspathHandler extends URLStreamHandler { private final ClassLoader classLoader; public ClasspathHandler(ClassLoader classLoader) { this.classLoader = classLoader; } @Override protected URLConnection openConnection(URL u) throws IOException { // Locate the resource using the classloader URL resourceUrl = classLoader.getResource(u.getPath()); // Open the connection to the resource return resourceUrl.openConnection(); } }
-
用法: 自定义处理程序可以与从类路径加载资源的 URL:
new URL("classpath:org/my/package/resource.extension").openConnection();
登录后复制
JVM 处理程序注册
要使处理程序全局可访问,请使用 URLStreamHandlerFactory 将其注册到 JVM:
import java.net.URL; import java.net.URLStreamHandler; import java.net.URLStreamHandlerFactory; import java.util.HashMap; import java.util.Map; public class ClasspathHandlerFactory implements URLStreamHandlerFactory { private final Map<String, URLStreamHandler> protocolHandlers; public ClasspathHandlerFactory() { protocolHandlers = new HashMap<String, URLStreamHandler>(); addHandler("classpath", new ClasspathHandler(ClassLoader.getSystemClassLoader())); } public void addHandler(String protocol, URLStreamHandler handler) { protocolHandlers.put(protocol, handler); } public URLStreamHandler createURLStreamHandler(String protocol) { return protocolHandlers.get(protocol); } }
使用配置好的工厂调用 URL.setURLStreamHandlerFactory() 进行注册它。
许可证
提供的实现已发布到公共领域。作者鼓励共享和公开修改。
附加说明
虽然这种方法提供了灵活性,但考虑潜在的问题也很重要,例如多个 JVM Handler Factory 注册的可能性和Tomcat 使用 JNDI 处理程序。因此,建议在所需的环境中进行测试。
以上是如何使用自定义 URL 协议从 Java 类路径加载资源?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
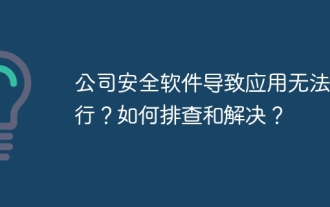
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
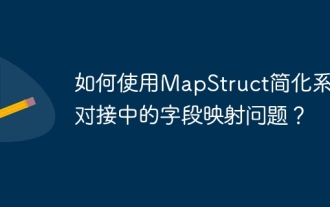
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
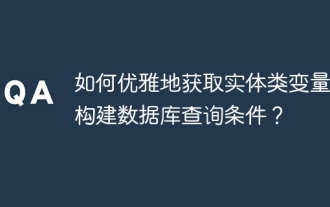
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
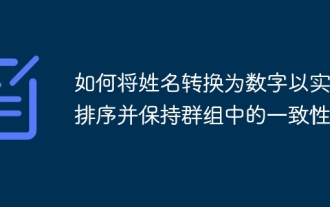
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
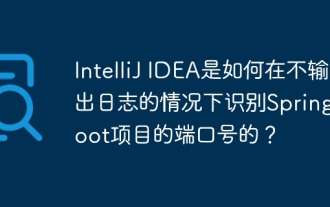
在使用IntelliJIDEAUltimate版本启动Spring...
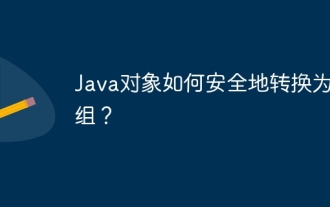
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
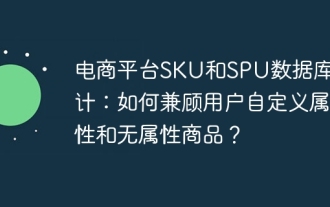
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
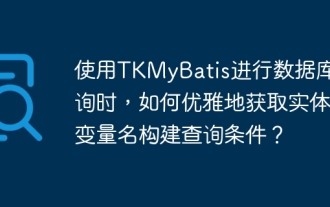
在使用TKMyBatis进行数据库查询时,如何优雅地获取实体类变量名以构建查询条件,是一个常见的难题。本文将针...
