为什么提供的用于颜色量化的 Java 代码很难有效地减少颜色,特别是在将颜色超过 256 的图像减少到 256 时,导致出现明显的错误,例如 re
有效的 GIF/图像颜色量化
在 Java 编程中,颜色量化在优化图像或 GIF 文件的调色板方面起着至关重要的作用。此过程涉及减少颜色数量,同时保持原始图像的视觉可接受的表示。
问题陈述:
提供的代码在减少颜色方面似乎效率低下有效地。当将超过 256 种颜色的图像减少到 256 种颜色时,会产生明显的错误,例如红色变成蓝色。这表明该算法难以识别和保留图像中的重要颜色。
推荐算法:
- 中值切割:该算法根据中值颜色值递归地将颜色空间分为两半,创建二叉树。然后,它选择颜色变化最小的子树作为叶节点,代表最终的调色板。
- 基于群体: 该算法根据颜色在颜色中的总体(频率)对颜色进行排序。图像并通过选择前“n”个最常见的颜色来创建调色板。
- k-Means:此算法将颜色空间划分为“k”个簇,其中每个簇由其平均颜色值表示。然后使用簇质心形成调色板。
示例实现:
以下是 Java 中中值切割算法的示例实现:
import java.util.Arrays; import java.util.Comparator; import java.awt.image.BufferedImage; public class MedianCutQuantizer { public static void quantize(BufferedImage image, int colors) { int[] pixels = image.getRGB(0, 0, image.getWidth(), image.getHeight(), null, 0, image.getWidth()); Arrays.sort(pixels); // Sort pixels by red, green, and blue channel values // Create a binary tree representation of the color space TreeNode root = new TreeNode(pixels); // Recursively divide the color space and create the palette TreeNode[] palette = new TreeNode[colors]; for (int i = 0; i < colors; i++) { palette[i] = root; root = divide(root); } // Replace pixels with their corresponding palette colors for (int i = 0; i < pixels.length; i++) { pixels[i] = getClosestColor(pixels[i], palette); } image.setRGB(0, 0, image.getWidth(), image.getHeight(), pixels, 0, image.getWidth()); } private static TreeNode divide(TreeNode node) { // Find the median color value int median = node.getMedianValue(); // Create two new nodes, one for each half of the color range TreeNode left = new TreeNode(); TreeNode right = new TreeNode(); // Divide the pixels into two halves for (int i = node.start; i < node.end; i++) { if (node.pixels[i] <= median) { left.addPixel(node.pixels[i]); } else { right.addPixel(node.pixels[i]); } } return left.count > right.count ? left : right; } private static int getClosestColor(int pixel, TreeNode[] palette) { int minDistance = Integer.MAX_VALUE; int closestColor = 0; for (TreeNode node : palette) { int distance = getDistance(pixel, node.getAverageValue()); if (distance < minDistance) { minDistance = distance; closestColor = node.getAverageValue(); } } return closestColor; } // Utility methods private static int getDistance(int color1, int color2) { int r1 = (color1 >> 16) & 0xFF; int g1 = (color1 >> 8) & 0xFF; int b1 = color1 & 0xFF; int r2 = (color2 >> 16) & 0xFF; int g2 = (color2 >> 8) & 0xFF; int b2 = color2 & 0xFF; return (r1 - r2) * (r1 - r2) + (g1 - g2) * (g1 - g2) + (b1 - b2) * (b1 - b2); } private static class TreeNode { int start; int end; int count; int[] pixels; Integer averageValue; public TreeNode() { this(new int[0], 0, 0); } public TreeNode(int[] pixels, int start, int end) { this.pixels = pixels; this.start = start; this.end = end; count = end - start; } public int getMedianValue() { return pixels[(start + end) / 2]; } public int getAverageValue() { if (averageValue == null) { int r = 0; int g = 0; int b = 0; for (int i = start; i < end; i++) { int pixel = pixels[i]; r += (pixel >> 16) & 0xFF; g += (pixel >> 8) & 0xFF; b += pixel & 0xFF; } averageValue = (r / count) << 16 | (g / count) << 8 | b / count; } return averageValue; } public void addPixel(int pixel) { int[] newPixels = new int[pixels.length + 1]; System.arraycopy(pixels, start, newPixels, start, end); newPixels[end] = pixel; pixels = newPixels; end++; count = end - start; averageValue = null; } } }
使用此实现或其他类似算法可以显着改进 Java 应用程序中的颜色量化过程,将图像颜色减少到 256 或更少时,可以获得视觉上可接受的结果。
以上是为什么提供的用于颜色量化的 Java 代码很难有效地减少颜色,特别是在将颜色超过 256 的图像减少到 256 时,导致出现明显的错误,例如 re的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
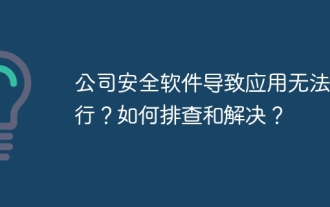
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
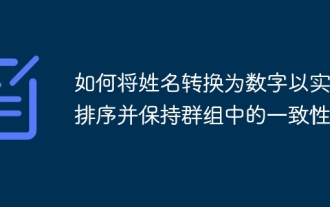
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
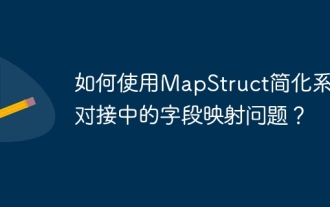
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
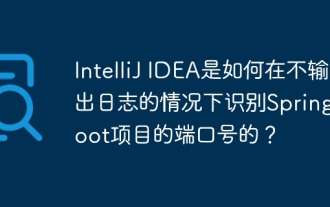
在使用IntelliJIDEAUltimate版本启动Spring...
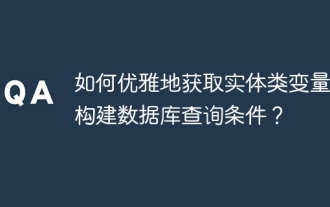
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
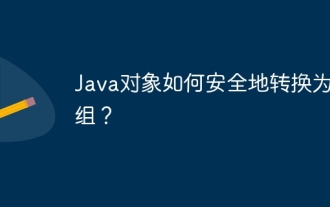
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
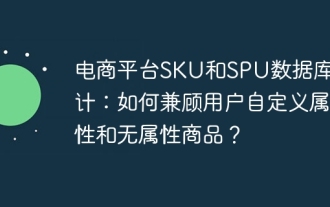
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
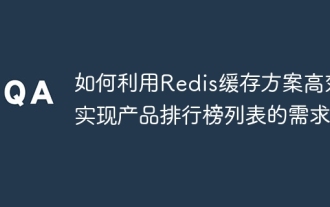
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
