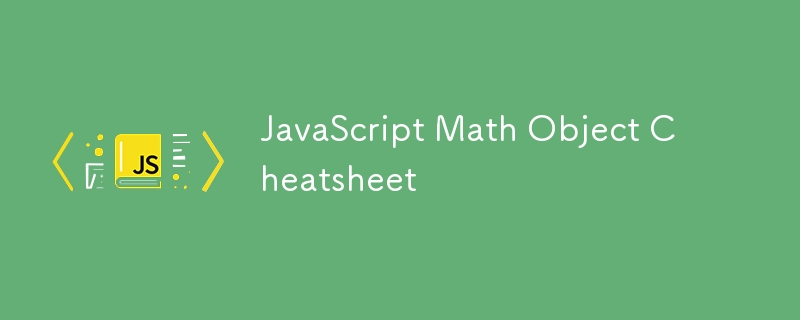
JavaScript 中的 Math 对象提供了一组用于执行数学任务的属性和方法。这是 Math 对象的综合备忘单。
属性
Math 对象有一组常量:
Property |
Description |
Value (Approx.) |
Math.E |
Euler's number |
2.718 |
Math.LN2 |
Natural logarithm of 2 |
0.693 |
Math.LN10 |
Natural logarithm of 10 |
2.302 |
Math.LOG2E |
Base 2 logarithm of Math.E
|
1.442 |
Math.LOG10E |
Base 10 logarithm of Math.E
|
0.434 |
Math.PI |
Ratio of a circle's circumference to its diameter |
3.14159 |
Math.SQRT1_2 |
Square root of 1/2 |
0.707 |
Math.SQRT2 |
Square root of 2 |
1.414 |
方法
1.舍入方法
Method |
Description |
Example |
Math.round(x) |
Rounds to the nearest integer |
Math.round(4.5) → 5
|
Math.floor(x) |
Rounds down to the nearest integer |
Math.floor(4.7) → 4
|
Math.ceil(x) |
Rounds up to the nearest integer |
Math.ceil(4.1) → 5
|
Math.trunc(x) |
Removes the decimal part (truncates) |
Math.trunc(4.9) → 4
|
2.随机数生成
Method |
Description |
Example |
Math.random() |
Generates a random number between 0 and 1 (exclusive) |
Math.random() → 0.53
|
Custom Random Int Generator |
Random integer between min and max
|
Math.floor(Math.random() * (max - min 1)) min |
3.算术方法
Method |
Description |
Example |
Math.abs(x) |
Absolute value |
Math.abs(-7) → 7
|
Math.pow(x, y) |
Raises x to the power of y
|
Math.pow(2, 3) → 8
|
Math.sqrt(x) |
Square root of x
|
Math.sqrt(16) → 4
|
Math.cbrt(x) |
Cube root of x
|
Math.cbrt(27) → 3
|
Math.hypot(...values) |
Square root of the sum of squares of arguments |
Math.hypot(3, 4) → 5
|
4.指数和对数方法
Method |
Description |
Example |
Math.exp(x) |
e^x |
Math.exp(1) → 2.718
|
Math.log(x) |
Natural logarithm (ln(x)) |
Math.log(10) → 2.302
|
Math.log2(x) |
Base 2 logarithm of x
|
Math.log2(8) → 3
|
Math.log10(x) |
Base 10 logarithm of x
|
Math.log10(100) → 2
|
5.三角函数
Method |
Description |
Example |
Math.sin(x) |
Sine of x (x in radians) |
Math.sin(Math.PI / 2) → 1
|
Math.cos(x) |
Cosine of x (x in radians) |
Math.cos(0) → 1
|
Math.tan(x) |
Tangent of x (x in radians) |
Math.tan(Math.PI / 4) → 1
|
Math.asin(x) |
Arcsine of x (returns radians) |
Math.asin(1) → 1.57
|
Math.acos(x) |
Arccosine of x
|
Math.acos(1) → 0
|
Math.atan(x) |
Arctangent of x
|
Math.atan(1) → 0.785
|
Math.atan2(y, x) |
Arctangent of y / x
|
Math.atan2(1, 1) → 0.785
|
6.最小值、最大值和钳位
Method |
Description |
Example |
Math.max(...values) |
Returns the largest value |
Math.max(5, 10, 15) → 15
|
Math.min(...values) |
Returns the smallest value |
Math.min(5, 10, 15) → 5
|
Custom Clamping |
Restrict a value to a range |
Math.min(Math.max(x, min), max) |
7.其他方法
Method |
Description |
Example |
Math.sign(x) |
Returns 1, -1, or 0 based on sign of x
|
Math.sign(-10) → -1
|
Math.fround(x) |
Nearest 32-bit floating-point number |
Math.fround(5.5) → 5.5
|
Math.clz32(x) |
Counts leading zero bits in 32-bit binary |
Math.clz32(1) → 31
|
示例
1 到 100 之间的随机整数
const randomInt = Math.floor(Math.random() * 100) + 1;
console.log(randomInt);
登录后复制
计算圆面积
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
登录后复制
登录后复制
将度数转换为弧度
const degrees = 90;
const radians = degrees * (Math.PI / 180);
console.log(radians); // 1.57
登录后复制
找到数组中最大的数字
const nums = [5, 3, 9, 1];
console.log(Math.max(...nums)); // 9
登录后复制
数学对象的扩展用例
Math 对象有许多实际应用。以下列出了常见场景和示例,以说明如何有效使用它。
1.随机化
生成一个范围内的随机整数
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(getRandomInt(1, 10)); // Random number between 1 and 10
登录后复制
打乱数组
function shuffleArray(arr) {
return arr.sort(() => Math.random() - 0.5);
}
console.log(shuffleArray([1, 2, 3, 4, 5])); // Shuffled array
登录后复制
模拟掷骰子
function rollDice() {
return Math.floor(Math.random() * 6) + 1; // Random number between 1 and 6
}
console.log(rollDice());
登录后复制
2.几何和形状
计算圆的面积
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
登录后复制
登录后复制
计算三角形的斜边
const a = 3, b = 4;
const hypotenuse = Math.hypot(a, b);
console.log(hypotenuse); // 5
登录后复制
将度数转换为弧度
function degreesToRadians(degrees) {
return degrees * (Math.PI / 180);
}
console.log(degreesToRadians(90)); // 1.57
登录后复制
3.金融与商业
复利公式
function compoundInterest(principal, rate, time, n) {
return principal * Math.pow((1 + rate / n), n * time);
}
console.log(compoundInterest(1000, 0.05, 10, 12)); // 47.01
登录后复制
四舍五入货币值
const amount = 19.56789;
const rounded = Math.round(amount * 100) / 100; // Round to 2 decimal places
console.log(rounded); // 19.57
登录后复制
计算折扣
function calculateDiscount(price, discount) {
return Math.floor(price * (1 - discount / 100));
}
console.log(calculateDiscount(200, 15)); // 0
登录后复制
4.游戏和动画
模拟抛硬币
function coinToss() {
return Math.random() < 0.5 ? 'Heads' : 'Tails';
}
console.log(coinToss());
登录后复制
平滑动画的缓动函数
function easeOutQuad(t) {
return t * (2 - t); // Simple easing function
}
console.log(easeOutQuad(0.5)); // 0.75
登录后复制
二维网格中的随机生成坐标
function randomCoordinates(gridSize) {
const x = Math.floor(Math.random() * gridSize);
const y = Math.floor(Math.random() * gridSize);
return { x, y };
}
console.log(randomCoordinates(10)); // e.g., {x: 7, y: 2}
登录后复制
5.数据分析
求数组中的最大值和最小值
const scores = [85, 90, 78, 92, 88];
console.log(Math.max(...scores)); // 92
console.log(Math.min(...scores)); // 78
登录后复制
标准化数据
function normalize(value, min, max) {
return (value - min) / (max - min);
}
console.log(normalize(75, 0, 100)); // 0.75
登录后复制
6.物理与工程
计算自由落体后的速度
const gravity = 9.8; // m/s^2
const time = 3; // seconds
const velocity = gravity * time;
console.log(velocity); // 29.4 m/s
登录后复制
钟摆周期
function pendulumPeriod(length) {
return 2 * Math.PI * Math.sqrt(length / 9.8);
}
console.log(pendulumPeriod(1)); // 2.006 seconds
登录后复制
7.数字操纵
将数字限制在某个范围内
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
}
console.log(clamp(15, 10, 20)); // 15
console.log(clamp(5, 10, 20)); // 10
登录后复制
将负数转换为正数
console.log(Math.abs(-42)); // 42
登录后复制
查找数字的整数部分
console.log(Math.trunc(4.9)); // 4
console.log(Math.trunc(-4.9)); // -4
登录后复制
8.解决问题
检查一个数字是否是 2 的幂
function isPowerOfTwo(n) {
return Math.log2(n) % 1 === 0;
}
console.log(isPowerOfTwo(8)); // true
console.log(isPowerOfTwo(10)); // false
登录后复制
生成斐波那契数
function fibonacci(n) {
const phi = (1 + Math.sqrt(5)) / 2;
return Math.round((Math.pow(phi, n) - Math.pow(-phi, -n)) / Math.sqrt(5));
}
console.log(fibonacci(10)); // 55
登录后复制
9.杂项
生成随机颜色 (RGB)
function getRandomColor() {
const r = Math.floor(Math.random() * 256);
const g = Math.floor(Math.random() * 256);
const b = Math.floor(Math.random() * 256);
return `rgb(${r}, ${g}, ${b})`;
}
console.log(getRandomColor()); // e.g., rgb(123, 45, 67)
登录后复制
根据出生日期计算年龄
function calculateAge(birthYear) {
const currentYear = new Date().getFullYear();
return currentYear - birthYear;
}
console.log(calculateAge(1990)); // e.g., 34
登录后复制
以上是JavaScript 数学对象备忘单的详细内容。更多信息请关注PHP中文网其他相关文章!