Axios 与 Fetch:哪个最适合 HTTP 请求?
在 JavaScript 中发出 HTTP 请求的方法有很多,但最流行的两种是 Axios 和本机 fetch() API。在这篇文章中,我们将比较和对比这两种方法,以确定哪一种更适合不同的场景。
HTTP 请求的基本作用
HTTP 请求是与 Web 应用程序中的服务器和 API 进行通信的基础。 Axios 和 fetch() 都被广泛用于有效地促进这些请求。让我们深入研究它们的功能,看看它们如何叠加。
什么是 Axios?
Axios 是一个第三方库,它提供基于 Promise 的 HTTP 客户端来发出 HTTP 请求。它以其简单性和灵活性而闻名,在 JavaScript 社区中得到广泛使用。
axios基本语法
axios(config) .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
Axios 的主要特点:
- 配置灵活性:同时接受 URL 和配置对象。
- 自动数据处理: 自动将数据与 JSON 相互转换。
- 错误处理:自动处理HTTP错误状态代码,将它们传递给catch块。
- 简化响应:直接在响应对象的 data 属性中返回服务器数据。
- 简化的错误管理:提供更简化的错误处理机制。
例子:
axios({ method: 'post', url: 'https://api.example.com/data', data: { key: 'value' } }) .then(response => console.log(response.data)) .catch(error => { if (error.response) { console.error('Server responded with:', error.response.status); } else if (error.request) { console.error('No response received'); } else { console.error('Error:', error.message); } });
为什么使用 Axios?
- 自动 JSON 数据转换: 无缝地将数据与 JSON 相互转换。
- 响应超时:允许设置请求超时。
- HTTP 拦截器: 让您拦截请求和响应。
- 下载进度:跟踪下载和上传的进度。
- 同时请求:同时处理多个请求并合并响应。
什么是抓取?
fetch() 是现代 JavaScript 中的内置 API,所有现代浏览器都支持。它是一个异步 Web API,以 Promise 的形式返回数据。
fetch() 的特点:
- 基本语法: 简单明了,采用 URL 和可选选项对象。
- 向后兼容性:可以在带有polyfills的旧版浏览器中使用。
- 可定制:允许对标头、正文、方法、模式、凭据、缓存、重定向和引用策略进行详细控制。
如何使用axios发出HTTP请求
首先,使用npm或yarn安装Axios:
axios(config) .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
您还可以通过 CDN 包含 Axios:
axios({ method: 'post', url: 'https://api.example.com/data', data: { key: 'value' } }) .then(response => console.log(response.data)) .catch(error => { if (error.response) { console.error('Server responded with:', error.response.status); } else if (error.request) { console.error('No response received'); } else { console.error('Error:', error.message); } });
以下是如何使用 Axios 发出 GET 请求:
npm install axios # or yarn add axios # or pnpm install axios
使用 Fetch 发出 HTTP 请求
由于 fetch() 是内置的,因此您不需要安装任何东西。以下是如何使用 fetch() 发出 GET 请求:
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
请注意:
- 数据处理: Axios 自动将数据与 JSON 相互转换,而使用 fetch() 时,您必须手动调用 response.json()。
- 错误处理: Axios 处理 catch 块内的错误,而 fetch() 仅拒绝网络错误的承诺,而不拒绝 HTTP 状态错误。
fetch 的基本语法
import axios from 'axios'; axios.get('https://example.com/api') .then(response => console.log(response.data)) .catch(error => console.error(error));
主要特点:
- 简单参数: 采用 URL 和可选配置对象。
- 手动数据处理:需要手动将数据转换为字符串。
- 响应对象:返回包含完整响应信息的响应对象。
- 错误处理:需要手动检查 HTTP 错误的响应状态代码。
例子:
fetch('https://example.com/api') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
axios 与 Fetch 的比较
发送带有查询参数的 GET 请求
Axios:
fetch(url, options) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
获取:
fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ key: 'value' }) }) .then(response => { if (!response.ok) throw new Error('HTTP error ' + response.status); return response.json(); }) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
使用 JSON 正文发送 POST 请求
Axios:
axios.get('/api/data', { params: { name: 'Alice', age: 25 } }) .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
获取:
const url = new URL('/api/data'); url.searchParams.append('name', 'Alice'); url.searchParams.append('age', 25); fetch(url) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
设置请求超时
Axios:
axios.post('/api/data', { name: 'Bob', age: 30 }) .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
获取:
fetch('/api/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name: 'Bob', age: 30 }) }) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
使用 async/await 语法
Axios:
axios.get('/api/data', { timeout: 5000 }) // 5 seconds .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
获取:
const controller = new AbortController(); const signal = controller.signal; setTimeout(() => controller.abort(), 5000); // abort after 5 seconds fetch('/api/data', { signal }) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
向后兼容性
Axios:
- 需要安装并包含在您的项目中。
- 通过 Promise 和其他现代 JavaScript 功能的 Polyfills 支持旧版浏览器。
- 积极维护与新环境的兼容性。
获取:
- 现代浏览器的本机支持。
- 可以进行填充以支持旧版浏览器。
- 由浏览器供应商自动更新。
错误处理
Axios:
处理 catch 块中的错误,并将 2xx 之外的任何状态代码视为错误:
async function getData() { try { const response = await axios.get('/api/data'); // handle response } catch (error) { // handle error } }
获取:
需要手动状态检查:
async function getData() { try { const response = await fetch('/api/data'); const data = await response.json(); // handle data } catch (error) { // handle error } }
Axios 与 Fetch:哪个最好?
没有明确的答案,这取决于您的要求:
- 如果您需要自动 JSON 数据转换、HTTP 拦截器和高级错误处理等功能,请使用 Axios。
- 如果您想要一个具有广泛自定义选项的本机、轻量级解决方案,请使用 fetch()。
使用 EchoAPI 生成 Axios/Fetch 代码
EchoAPI 是一个一体化协作 API 开发平台,提供用于设计、调试、测试和模拟 API 的工具。 EchoAPI 可以自动生成用于发出 HTTP 请求的 Axios 代码。
使用 EchoAPI 生成 Axios 代码的步骤:
1. 打开EchoAPI并创建一个新请求。
2. 输入API端点、标头和参数,然后单击“代码片段”。
3. 选择“生成客户端代码”。
4. 将生成的 Axios 代码复制并粘贴到您的项目中。
结论
Axios 和 fetch() 都是在 JavaScript 中发出 HTTP 请求的强大方法。选择最适合您的项目需求和偏好的一种。使用 EchoAPI 等工具可以增强您的开发工作流程,确保您的代码准确高效。快乐编码!
以上是Axios 与 Fetch:哪个最适合 HTTP 请求?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
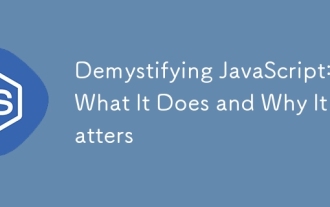
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
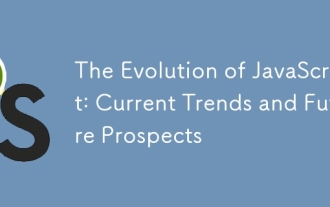
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
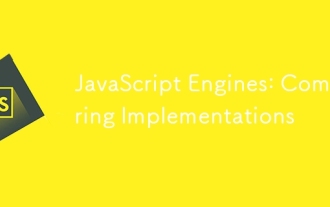
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
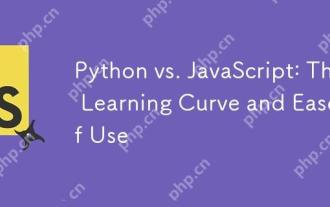
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
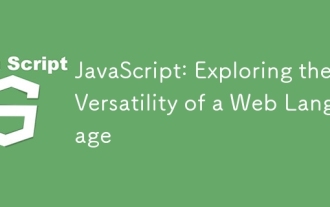
JavaScript是现代Web开发的核心语言,因其多样性和灵活性而广泛应用。1)前端开发:通过DOM操作和现代框架(如React、Vue.js、Angular)构建动态网页和单页面应用。2)服务器端开发:Node.js利用非阻塞I/O模型处理高并发和实时应用。3)移动和桌面应用开发:通过ReactNative和Electron实现跨平台开发,提高开发效率。
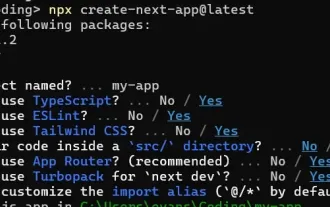
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库
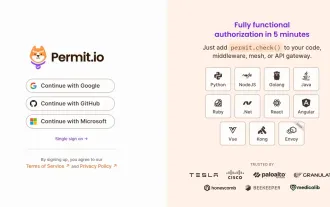
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
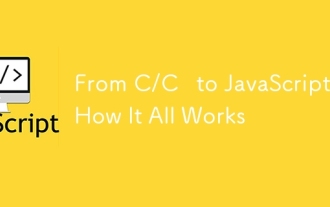
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
