如何修改 Go Map 值中的结构体字段?
寻址映射值
在 Go 中,尝试直接修改映射值中的结构体字段,如下例所示,将会导致编译错误:
import ( "fmt" ) type pair struct { a float64 b float64 } func main() { // Create a map where values are of the "pair" type. dictionary := make(map[string]pair) // Add an element to the map. dictionary["xxoo"] = pair{5.0, 2.0} fmt.Println(dictionary["xxoo"]) // Output: {5 2} // Attempt to modify a field within the map value. dictionary["xxoo"].b = 5.0 // Error: cannot assign to dictionary["xxoo"].b }
遇到此错误消息是因为映射值不可寻址。可寻址性是Go中的一个基本概念,它指的是定位变量内存地址的能力。无法间接修改不可寻址值,因为尝试访问不可寻址值的结构体字段会导致编译错误。
要解决此问题,主要有两种方法:
使用指针值
一种方法是使用指针值作为映射值。这种间接寻址使得值可寻址,从而允许字段修改。下面是一个示例:
import ( "fmt" ) type pair struct { a float64 b float64 } func main() { // Create a map where values are pointers to "pair" structs. dictionary := make(map[string]*pair) // Add an element to the map. dictionary["xxoo"] = &pair{5.0, 2.0} fmt.Println(dictionary["xxoo"]) // Output: &{5 2} // Modify a field within the pointed-to struct. dictionary["xxoo"].b = 5.0 fmt.Println(dictionary["xxoo"].b) // Output: 5 }
值复制或替换
或者,您可以通过复制值或完全替换它来处理不可寻址的值。以下是两个示例:
// Value Copying dictionary["xxoo"] = pair{5.0, 5.0}
// Value Replacement p := dictionary["xxoo"] p.b = 5.0 dictionary["xxoo"] = p
这两种方法都允许您修改映射中的“pair”结构。
以上是如何修改 Go Map 值中的结构体字段?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
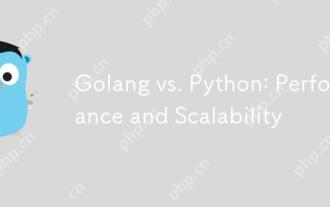
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
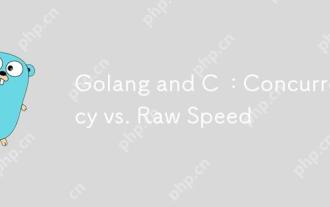
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
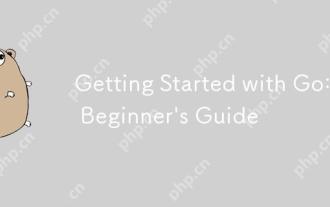
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
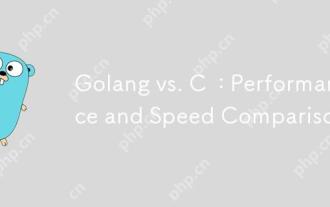
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
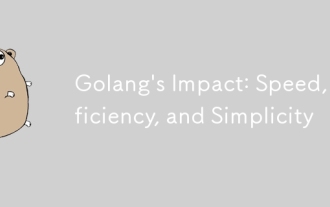
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
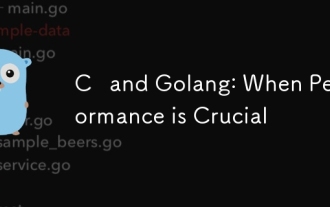
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
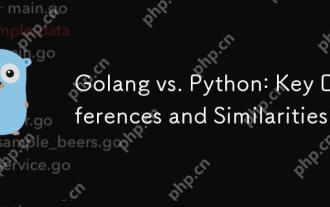
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
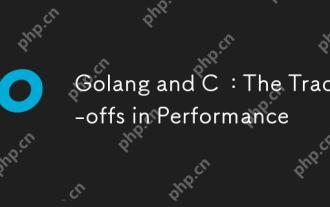
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
