使用 Cookie 处理 PHP 表单的初学者指南
在本指南中,我们将探索使用 cookie 存储用户数据的 PHP 表单处理。 Cookie 是一种在用户浏览器上保存少量数据的方法,可以在不同会话中记住用户偏好或信息。
我们的项目涉及创建一个表单,用户可以在其中输入信息,将提交的数据存储在 cookie 中,然后查看或删除 cookie 数据。在本教程结束时,您将了解如何在 PHP 中设置、检索和删除 cookie。
什么是 Cookie?
Cookie 是存储在用户浏览器上的小文件。它们允许网络服务器存储特定于用户的数据并在后续访问时检索它。在 PHP 中,您可以使用 setcookie() 函数来创建或更新 cookie,并使用 $_COOKIE 超全局变量来读取它们。
项目:带有 Cookie 处理的 PHP 表单
我们将创建一个简单的应用程序:
- 允许用户通过表单提交信息。
- 将提交的数据存储在cookie中。
- 显示存储的cookie数据。
- 提供删除 cookie 的选项。
文件结构
我们的项目包含以下文件:
project-folder/ │ ├── index.php # Form page ├── submit.php # Form handling and cookie storage ├── view_cookie.php # Viewing cookie data ├── delete_cookie.php # Deleting cookie data
第 1 步:创建表单 (index.php)
index.php 文件包含用于用户输入的 HTML 表单,以及用于查看或删除 cookie 数据的按钮。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP Form with Cookie Handling</title> </head> <body> <h1>Submit Your Information</h1> <!-- Form Section for User Input --> <form method="get" action="submit.php"> <label for="name">Name:</label><br> <input type="text"> <hr> <h3> Step 2: Handling Form Submission (submit.php) </h3> <p>The submit.php file processes the form data, validates and sanitizes it, and then stores it in cookies.<br> </p> <pre class="brush:php;toolbar:false"><?php // Initialize error messages and data variables $error_name = ""; $error_age = ""; $error_email = ""; $error_website = ""; $name = $age = $email = $website = $gender = $comments = $hobbies = ""; // Sanitize and validate the form data if ($_SERVER["REQUEST_METHOD"] == "GET") { // Sanitize inputs $name = htmlspecialchars(trim($_GET['name'])); $age = htmlspecialchars(trim($_GET['age'])); $email = htmlspecialchars(trim($_GET['email'])); $website = htmlspecialchars(trim($_GET['website'])); $gender = isset($_GET['gender']) ? $_GET['gender'] : ''; $hobbies = isset($_GET['hobbies']) ? $_GET['hobbies'] : []; $comments = htmlspecialchars(trim($_GET['comments'])); // Validation checks if (empty($name)) { $error_name = "Name is required."; } if (empty($age) || !filter_var($age, FILTER_VALIDATE_INT) || $age <= 0) { $error_age = "Valid age is required."; } if (empty($email) || !filter_var($email, FILTER_VALIDATE_EMAIL)) { $error_email = "Valid email is required."; } if (empty($website) || !filter_var($website, FILTER_VALIDATE_URL)) { $error_website = "Valid website URL is required."; } // If no errors, set cookies if (empty($error_name) && empty($error_age) && empty($error_email) && empty($error_website)) { // Set cookies for the form data setcookie("name", $name, time() + (86400 * 30), "/"); setcookie("age", $age, time() + (86400 * 30), "/"); setcookie("email", $email, time() + (86400 * 30), "/"); setcookie("website", $website, time() + (86400 * 30), "/"); setcookie("gender", $gender, time() + (86400 * 30), "/"); setcookie("hobbies", implode(", ", $hobbies), time() + (86400 * 30), "/"); setcookie("comments", $comments, time() + (86400 * 30), "/"); } } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Form Submission Result</title> </head> <body> <h1>Form Submission Result</h1> <!-- Show Errors if any --> <?php if ($error_name) { echo "<p> <hr> <h3> Step 3: Viewing Cookie Data (view_cookie.php) </h3> <p>This file displays the cookie data stored on the user's browser.<br> </p> <pre class="brush:php;toolbar:false"><!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>View Cookie Data</title> </head> <body> <h1>View Stored Cookie Data</h1> <?php if (isset($_COOKIE['name'])) { echo "<p><strong>Name:</strong> " . $_COOKIE['name'] . "</p>"; echo "<p><strong>Age:</strong> " . $_COOKIE['age'] . "</p>"; echo "<p><strong>Email:</strong> " . $_COOKIE['email'] . "</p>"; echo "<p><strong>Website:</strong> <a href='" . $_COOKIE['website'] . "' target='_blank'>" . $_COOKIE['website'] . "</a></p>"; echo "<p><strong>Gender:</strong> " . $_COOKIE['gender'] . "</p>"; echo "<p><strong>Hobbies:</strong> " . $_COOKIE['hobbies'] . "</p>"; echo "<p><strong>Comments:</strong> " . $_COOKIE['comments'] . "</p>"; } else { echo "<p>No cookie data found!</p>"; } ?> <br><br> <a href="index.php">Go Back</a> </body> </html>
步骤 4:删除 Cookie 数据 (delete_cookie.php)
此文件通过将 Cookie 的过期时间设置为过去来删除 Cookie。
<?php // Deleting cookies by setting their expiration time to past setcookie("name", "", time() - 3600, "/"); setcookie("age", "", time() - 3600, "/"); setcookie("email", "", time() - 3600, "/"); setcookie("website", "", time() - 3600, "/"); setcookie("gender", "", time() - 3600, "/"); setcookie("hobbies", "", time() - 3600, "/"); setcookie("comments", "", time() - 3600, "/"); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Cookie Deleted</title> </head> <body> <h1>Cookies Deleted</h1> <p>All cookies have been deleted successfully.</p> <br><br> <a href="index.php">Go Back</a> </body> </html>
结论
该项目演示了如何在 PHP 中使用 cookie 进行表单处理。通过实施 cookie,您可以保留用户数据并改进 Web 应用程序的功能。尝试此项目并探索 PHP 中 cookie 的更高级用例。
编码愉快! ?
以上是使用 Cookie 处理 PHP 表单的初学者指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
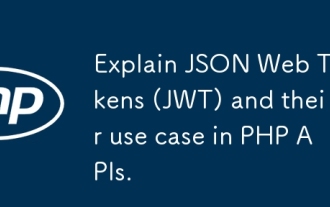
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
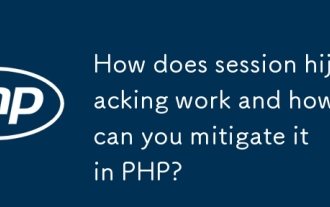
会话劫持可以通过以下步骤实现:1.获取会话ID,2.使用会话ID,3.保持会话活跃。在PHP中防范会话劫持的方法包括:1.使用session_regenerate_id()函数重新生成会话ID,2.通过数据库存储会话数据,3.确保所有会话数据通过HTTPS传输。
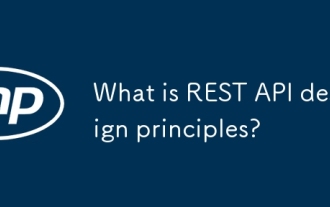
RESTAPI设计原则包括资源定义、URI设计、HTTP方法使用、状态码使用、版本控制和HATEOAS。1.资源应使用名词表示并保持层次结构。2.HTTP方法应符合其语义,如GET用于获取资源。3.状态码应正确使用,如404表示资源不存在。4.版本控制可通过URI或头部实现。5.HATEOAS通过响应中的链接引导客户端操作。
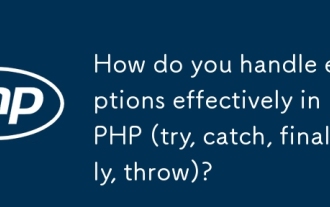
在PHP中,异常处理通过try,catch,finally,和throw关键字实现。1)try块包围可能抛出异常的代码;2)catch块处理异常;3)finally块确保代码始终执行;4)throw用于手动抛出异常。这些机制帮助提升代码的健壮性和可维护性。
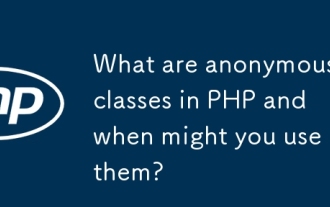
匿名类在PHP中的主要作用是创建一次性使用的对象。1.匿名类允许在代码中直接定义没有名字的类,适用于临时需求。2.它们可以继承类或实现接口,增加灵活性。3.使用时需注意性能和代码可读性,避免重复定义相同的匿名类。
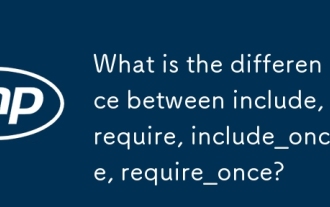
在PHP中,include,require,include_once,require_once的区别在于:1)include产生警告并继续执行,2)require产生致命错误并停止执行,3)include_once和require_once防止重复包含。这些函数的选择取决于文件的重要性和是否需要防止重复包含,合理使用可以提高代码的可读性和可维护性。
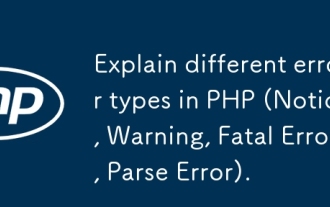
PHP中有四种主要错误类型:1.Notice:最轻微,不会中断程序,如访问未定义变量;2.Warning:比Notice严重,不会终止程序,如包含不存在文件;3.FatalError:最严重,会终止程序,如调用不存在函数;4.ParseError:语法错误,会阻止程序执行,如忘记添加结束标签。
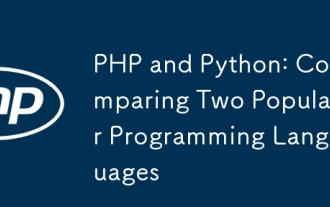
PHP和Python各有优势,选择依据项目需求。1.PHP适合web开发,尤其快速开发和维护网站。2.Python适用于数据科学、机器学习和人工智能,语法简洁,适合初学者。
