高级 Python 概念 - 元编程
想象一下编写一个Python代码,它可以根据实时数据输入修改自身或动态生成新代码。 元编程是一种强大而先进的编程技术,允许开发人员编写可以操作其他代码的代码,并在运行时生成新代码。 就像我们说的,元数据是数据的数据,元编程也是关于编写操作代码的代码。 因此,本文讨论了提高代码效率和灵活性的元编程功能。 我们将通过提供每个概念的实际示例来了解它的基础、装饰器、元类和动态代码执行。让我们开始吧!
理解元编程
1. 元编程及其在 Python 中的作用
在 Python 中,元编程是指编写计算机程序,协助编写和操作其他程序。该技术允许程序将其他程序视为数据。它生成代码,修改现有代码,并在运行时创建新的编程构造。
2. 元编程和常规编程
在继续讨论元编程概念的技术方面之前,让我们首先看看基于过程步骤的通用或常规编程与高级编程概念有何不同。
3. 使用元编程的好处和风险
元编程为我们提供了一系列好处。让我们来探索一下它们,了解它们在开发过程中的优势。
- 元编程允许程序在运行时修改自身,从而缩短了开发时间。该技术使开发人员能够编写更少的代码,从而使整个开发过程比传统的软件开发方法更加高效。
- 它提供代码重复的解决方案并减少编码时间。众所周知,元编程就是减少开发人员端的代码并创建一种在运行时自动生成代码的方式。
- 程序在运行时动态调整其行为,以响应某些条件和输入数据。这使得软件程序更加强大和灵活。
与优点类似,元编程也有一些缺点,开发人员在使用此技术之前请记住这些缺点。
- 元编程的一个风险是其复杂的语法。
- 由于代码是在运行时动态生成的,因此存在看不见的错误问题。这些错误来自生成的代码,很难跟踪和解决。有时,找到错误的来源和原因变得很困难。
- 计算机程序的执行时间比平时要长,因为 Python 在运行时执行新的元编程代码。
元类:元编程的基础
1. 元类动态创建类的机制
元类定义类的行为和结构。使用 Python 中的元类,您可以轻松自定义类的创建和行为。这是可能的,因为 Python 将一切(包括类)表示为对象。此外,对象是使用类创建的。因此,这个假设的“类”充当另一个类的子类,该类是超类的元类。此外,所有 Python 类都是元类的子类。
注意:
Type 是 python 中默认的元类。它用于动态创建类。
2. 元类‘__new__’和‘__init__’方法
在Python中,元类默认是“类型”类,即用于管理类的创建和行为的基类。在 Python 中创建类时,我们间接使用了“type”类。元类由两个主要方法组成:__new__ 和 __init__。 __new__ 方法用于创建新对象。该方法创建并返回实例,然后将其传递给 __init__ 方法进行初始化。它在 __init__ 方法之前调用,并确保类本身的控件创建。然后,在创建新类后使用 __init__ 方法用进一步的属性和方法对其进行初始化。这种方法与常规的编程方法有很大不同。它允许我们在类创建后修改和设置类级属性。
提示:
new 和 init 方法用于创建自定义类及其行为
3. 示例:创建自定义元类以自定义类创建行为
让我们通过一个简单的 Python 示例来了解如何使用元类主要方法 __new__ 和 __init__ 创建自定义元类来自定义类的创建及其行为。
# Define the metaclass class Meta(type): #define the new method for creating the class instance #cls: metaclass whose instance is being created #name: name of the class #base: means the base class #class_dict: represent the dictionary of attributes for a class def __new__(cls, name, bases, attrs): #making the attributes(method) name as upper case uppercase_attrs = {key.upper(): value for key, value in attrs.items() if not key.startswith('__')} new_class = super().__new__(cls, name, bases, uppercase_attrs) print("Class {name} has been created with Meta") return new_class #the class is initialized def __init__(cls, name, bases, dct): super().__init__(name, bases, dct) print(f"Class {name} initilized with Meta") # Using the metaclass in a new class class MyClass(metaclass=Meta): def my_method(self): print(f"Hello!") # Instantiate MyClass and access its custom attribute obj = MyClass() #here the attribute of the class is change into uppercase i.e. the name of method obj.MY_METHOD()
输出
注意:
请记住,在输出中,“Hello”字符串不会转换为大写,但方法名称“my_method”会转换为“MY_METHOD”,它将打印该字符串。这意味着我们正在将方法名称转换为大写。
装饰器:函数级别的元编程
1. 装饰器作为修改其他函数行为的函数
装饰器是 Python 元编程的关键特性。装饰器是一项强大的功能,允许开发人员修改现有代码而不更改原始源代码。它允许您通过扩展现有功能来添加新功能。装饰器通常在函数上执行,其语法在其代码之前使用“@”符号和装饰器函数名称。在 Python 中,装饰器充当其他函数和类的包装器。装饰器的输入和输出是函数本身,通常在原始函数之前和之后执行功能。
2. 装饰器的语法
装饰器使用 @decorator_name 作为语法。而 Decorator_name 是您作为装饰器创建的函数的名称。
# Define the metaclass class Meta(type): #define the new method for creating the class instance #cls: metaclass whose instance is being created #name: name of the class #base: means the base class #class_dict: represent the dictionary of attributes for a class def __new__(cls, name, bases, attrs): #making the attributes(method) name as upper case uppercase_attrs = {key.upper(): value for key, value in attrs.items() if not key.startswith('__')} new_class = super().__new__(cls, name, bases, uppercase_attrs) print("Class {name} has been created with Meta") return new_class #the class is initialized def __init__(cls, name, bases, dct): super().__init__(name, bases, dct) print(f"Class {name} initilized with Meta") # Using the metaclass in a new class class MyClass(metaclass=Meta): def my_method(self): print(f"Hello!") # Instantiate MyClass and access its custom attribute obj = MyClass() #here the attribute of the class is change into uppercase i.e. the name of method obj.MY_METHOD()
语法也使用如下,它显示装饰器将一个函数作为参数并将结果保存到另一个函数中。
@decorator_name def function_name():
3. 创建和使用装饰器向函数添加功能的插图
下面是一个使用装饰器将一个函数的字符串转换为大写的示例,这意味着将大写功能添加到函数中:
Function_name = decorator_name(function_name)
输出
“检查”模块:内省与反思
1. 用于内省和反思的 `Inspect` 模块简介
在元编程世界中,检查和反思是关键术语。执行检查是为了检查程序中对象的类型和属性,并在运行时提供有关它的报告。相反,反射涉及在运行时修改对象的结构和行为。这两个语言特性使Python成为强类型动态语言。我们可以使用“inspect”模块在元编程中执行检查和反射。该模块提供了各种用于自省的功能,包括有关对象的类型和属性、源代码和调用堆栈的信息。
2. 如何使用“inspect”模块在运行时检查和修改对象
让我们了解一下,使用“inspect”模块进行内省和反射,结合其他Python功能,我们可以在元编程中在运行时检查和修改对象。我们将一步步学习:
1. 使用“inspect”模块检查对象
# Define the metaclass class Meta(type): #define the new method for creating the class instance #cls: metaclass whose instance is being created #name: name of the class #base: means the base class #class_dict: represent the dictionary of attributes for a class def __new__(cls, name, bases, attrs): #making the attributes(method) name as upper case uppercase_attrs = {key.upper(): value for key, value in attrs.items() if not key.startswith('__')} new_class = super().__new__(cls, name, bases, uppercase_attrs) print("Class {name} has been created with Meta") return new_class #the class is initialized def __init__(cls, name, bases, dct): super().__init__(name, bases, dct) print(f"Class {name} initilized with Meta") # Using the metaclass in a new class class MyClass(metaclass=Meta): def my_method(self): print(f"Hello!") # Instantiate MyClass and access its custom attribute obj = MyClass() #here the attribute of the class is change into uppercase i.e. the name of method obj.MY_METHOD()
输出
2. 在运行时修改对象
@decorator_name def function_name():
输出
这是您在运行时动态检查和执行修改的方法。将检查模块与 Python 的内置函数(如 setattr 和 delattr)结合使用将允许开发人员编写可以在运行时更改的灵活且自适应的代码。
提示:
setattr 和 delattr 都是用于动态更改对象属性的 Python 函数。在这些函数中,setattr 用于设置和更改属性,delattr 用于从对象中删除属性。
3. 内省与反思的实际用例
调试和代码分析
正如我们所知,调试比第一次编写代码更加忙碌和耗时。开发人员调试代码以验证并找到缺陷来源,以便在早期阶段进行处理。然而,当我们无法识别其来源时,它是一个非常异构的过程。因此,内省和反射对于调试代码非常有用。它通过提供对象性质的详细信息(包括其行为)在运行时动态检查对象。它提供对象属性值和意外值的详细信息,并解释对象的状态如何随时间变化。为了更清楚地说明这一点,让我们举一个例子。
Function_name = decorator_name(function_name)
输出
总结
综上,我们讨论了Python的高级概念,那就是元编程。众所周知,元编程是扩展和修改Python语言本身行为的技术。它可以帮助您编写可以修改和生成其他函数的函数。我们可以使用不同的方法执行元编程,例如元类允许我们使用默认类型类,然后使用装饰器,它充当另一个函数的包装器并转向技术预先调试代码。因此,无论您在何处学习 Python 高级概念,都不要忘记了解元编程的重要性。我希望本指南对您有所帮助。感谢您的阅读。快乐编码!
额外参考
Python 检查模块
Python 中的元类
装饰器
以上是高级 Python 概念 - 元编程的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
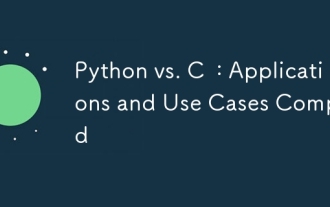
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。 Python以简洁和强大的生态系统着称,C 则以高性能和底层控制能力闻名。
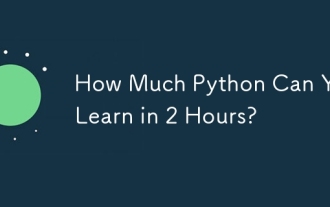
两小时内可以学到Python的基础知识。1.学习变量和数据类型,2.掌握控制结构如if语句和循环,3.了解函数的定义和使用。这些将帮助你开始编写简单的Python程序。
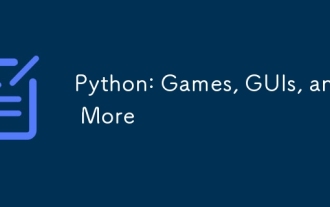
Python在游戏和GUI开发中表现出色。1)游戏开发使用Pygame,提供绘图、音频等功能,适合创建2D游戏。2)GUI开发可选择Tkinter或PyQt,Tkinter简单易用,PyQt功能丰富,适合专业开发。
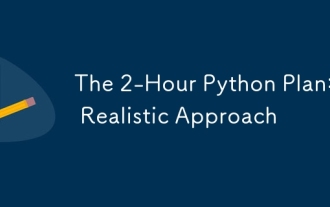
2小时内可以学会Python的基本编程概念和技能。1.学习变量和数据类型,2.掌握控制流(条件语句和循环),3.理解函数的定义和使用,4.通过简单示例和代码片段快速上手Python编程。
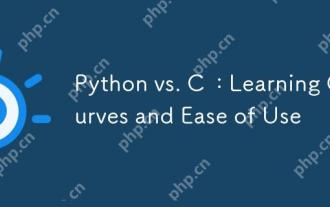
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
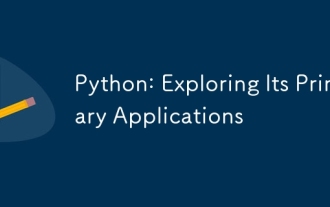
Python在web开发、数据科学、机器学习、自动化和脚本编写等领域有广泛应用。1)在web开发中,Django和Flask框架简化了开发过程。2)数据科学和机器学习领域,NumPy、Pandas、Scikit-learn和TensorFlow库提供了强大支持。3)自动化和脚本编写方面,Python适用于自动化测试和系统管理等任务。
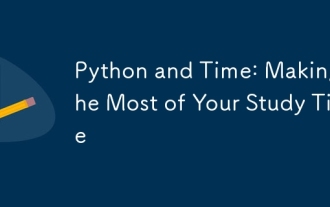
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
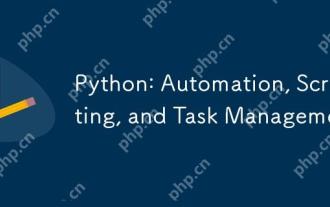
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
