如何根据特定属性高效地从ArrayList中检索对象?
根据属性从ArrayList中检索对象
在面向对象编程中,经常会遇到需要通过属性来定位对象的场景集合中的特定属性。这在 Java EE 等框架中特别有用,您可能在其中使用 POJO 并需要从内存缓存中检索对象。
考虑一个场景,其中您正在管理 Carnet 对象列表,每个都拥有 codeTitre、nomTitre 和 codeIsin 等属性。假设您需要根据其 codeIsin 查找特定的 Carnet 对象。为了实现这一点,您可以利用 Java 流的强大功能:
public static Carnet findByCodeIsIn(Collection<Carnet> listCarnet, String codeIsIn) { return listCarnet.stream().filter(carnet -> codeIsIn.equals(carnet.getCodeIsin())).findFirst().orElse(null); }
此代码片段利用流来有效地搜索 Carnet 对象集合,过滤掉那些与给定 codeIsIn 条件不匹配的对象。通过利用 findFirst() 方法,您可以检索第一个匹配的对象,如果没有找到对象,则返回 null。
您还可能会遇到需要查找具有不同属性或跨多种类型对象的对象的情况。为了解决这个问题,您可以引入像 FindUtils 这样的实用程序类:
public final class FindUtils { public static <T> T findByProperty(Collection<T> col, Predicate<T> filter) { return col.stream().filter(filter).findFirst().orElse(null); } }
这个实用程序类提供了一个通用方法,可用于根据任何指定的条件搜索对象。
此外,您可以为每种对象类型创建特定的实用程序类,例如 CarnetUtils:
public final class CarnetUtils { public static Carnet findByCodeTitre(Collection<Carnet> listCarnet, String codeTitre) { return FindUtils.findByProperty(listCarnet, carnet -> codeTitre.equals(carnet.getCodeTitre())); } // Similar methods for finding by other properties }
通过使用这些实用程序类,您可以轻松灵活地搜索 ArrayList 中的对象,无论其具体属性如何。
以上是如何根据特定属性高效地从ArrayList中检索对象?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
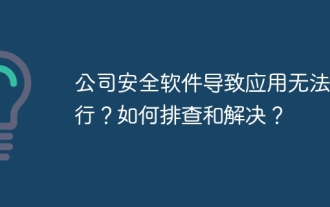
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
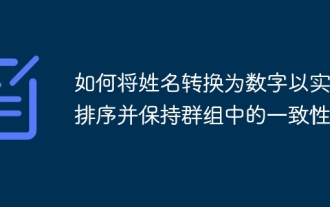
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
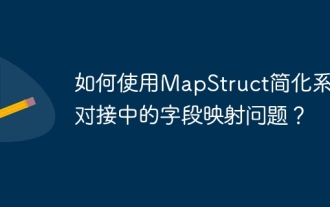
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
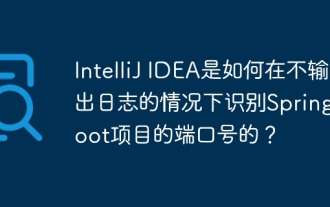
在使用IntelliJIDEAUltimate版本启动Spring...
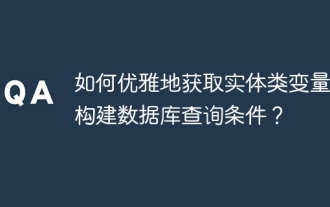
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
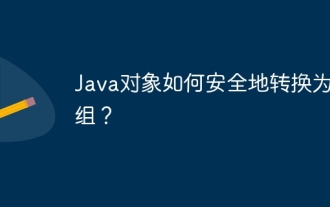
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
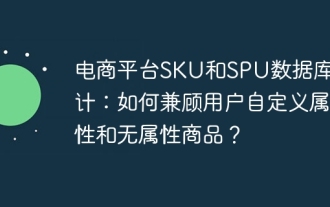
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
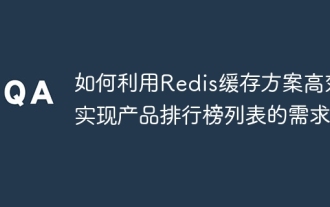
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
