如何在 JavaScript 中使用 Promise 实现异步链接?
JavaScript ES6 中使用 Promise 进行异步链接
提供的 JavaScript 代码片段尝试在循环内创建 Promise,但由于同步而失败循环的性质。为了解决这个问题,我们要求按顺序创建和解析每个 Promise,从而实现异步。
有多种方法可以实现此链接:
使用 For 循环和初始 Promise:
初始化一个立即解决的 Promise,然后将新的 Promise 作为每个先前的 Promise 链接到此初始 Promise
const delay = ms => new Promise(resolve => setTimeout(resolve, ms)); for (let i = 0, p = Promise.resolve(); i < 10; i++) { p = p.then(() => delay(Math.random() * 1000)) .then(() => console.log(i)); }
将 Array.reduce 与初始 Promise 结合使用:
与 for 循环方法类似,使用 Array.reduce 来链接 Promise,从一个初始 Promise 开始最初的承诺。
[...Array(10).keys()].reduce( (p, i) => p.then(() => delay(Math.random() * 1000)).then(() => console.log(i)), Promise.resolve() );
递归地使用链接函数:
定义一个函数,将自身调用为解析回调(如承诺链),并将当前索引作为参数传递。
const chainPromises = (i = 0) => { if (i >= 10) return; delay(Math.random() * 1000).then(() => { console.log(i); chainPromises(i + 1); }); }; chainPromises();
使用Async/Await (ES2017):
使用 async/await 暂停执行
const asyncPromises = async () => { for (let i = 0; i < 10; i++) { await delay(Math.random() * 1000); console.log(i); } }; asyncPromises();
使用 For Await...Of (ES2020):
与 async/await 类似,使用 for await.. .of 语法来迭代异步可迭代。
const asyncPromises = async () => { for await (let i of [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) { await delay(Math.random() * 1000); console.log(i); } }; asyncPromises();
以上是如何在 JavaScript 中使用 Promise 实现异步链接?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
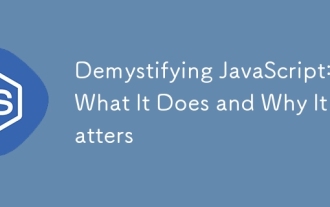
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
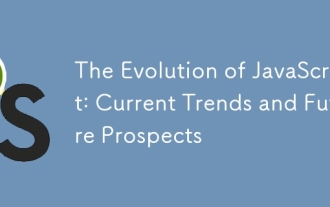
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
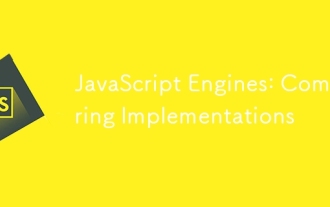
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
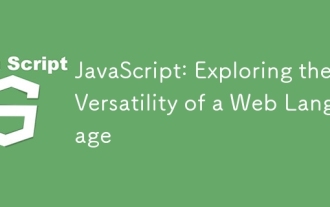
JavaScript是现代Web开发的核心语言,因其多样性和灵活性而广泛应用。1)前端开发:通过DOM操作和现代框架(如React、Vue.js、Angular)构建动态网页和单页面应用。2)服务器端开发:Node.js利用非阻塞I/O模型处理高并发和实时应用。3)移动和桌面应用开发:通过ReactNative和Electron实现跨平台开发,提高开发效率。
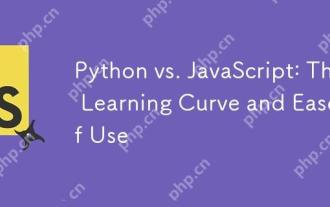
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
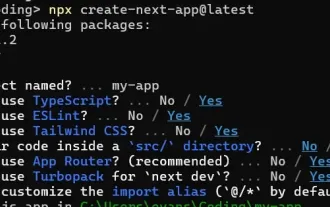
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库
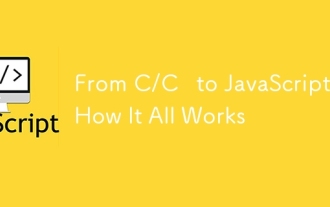
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
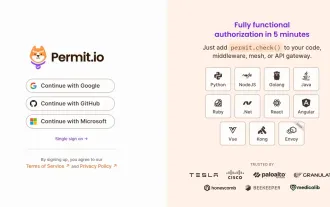
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
