JavaScript 中的密码学:实用指南
密码学通过将数据转换为只有目标接收者可以理解的格式来保护数据。它对于保护密码、在线交易和敏感通信的安全至关重要。下面,您将了解加密、散列以及使用 JavaScript 来实现它们。
什么是密码学?
密码学将可读数据(明文)转换为不可读的格式(密文)。只有授权方才能逆转该过程。
关键概念:
- 加密:将明文转换为密文。
- 解密:使用密钥将密文反转回明文。
加密类型
1. 对称加密
使用相同的密钥进行加密和解密。密钥必须在发送者和接收者之间安全地共享。 AES 是一种广泛使用的对称加密算法,它通过将数据转换为不可读的格式来保护数据。它依赖于密钥并支持 128、192 或 256 位密钥长度,提供针对未经授权的访问的强大保护。 AES 对于以下方面至关重要:
- 保护互联网通信:保护 HTTPS 等在线交互。
- 保护敏感数据:确保存储和传输的机密性。
- 加密文件:保证个人和专业信息的安全。
AES 的关键要素
AES 的关键元素包括密钥 和初始化向量(IV)。密钥是各方之间共享的秘密值,决定数据的加密和解密方式,并且必须始终保持机密。 IV 是与密钥一起使用的随机值,以确保相同的明文加密为不同的密文,从而增加随机性以防止模式识别。虽然 IV 可以公开,但绝不能用相同的密钥重复使用。这些元素共同使 AES 能够有效应对网络威胁,使其成为数据安全的基石。
示例:AES(高级加密标准)
AES 使用共享密钥和初始化向量 (IV) 加密数据以增加随机性。
const crypto = require('crypto'); const algorithm = 'aes-256-cbc'; const key = crypto.randomBytes(32); const iv = crypto.randomBytes(16); function encrypt(text) { const cipher = crypto.createCipheriv(algorithm, key, iv); let encrypted = cipher.update(text, 'utf8', 'hex'); encrypted += cipher.final('hex'); return { encrypted, iv: iv.toString('hex'), key: key.toString('hex') }; } function decrypt(encrypted, ivHex, keyHex) { const decipher = crypto.createDecipheriv(algorithm, Buffer.from(keyHex, 'hex'), Buffer.from(ivHex, 'hex')); let decrypted = decipher.update(encrypted, 'hex', 'utf8'); decrypted += decipher.final('utf8'); return decrypted; } // Usage const message = "Secret Message"; const encryptedData = encrypt(message); console.log("Encrypted:", encryptedData); const decryptedMessage = decrypt(encryptedData.encrypted, encryptedData.iv, encryptedData.key); console.log("Decrypted:", decryptedMessage);
2. 非对称加密
要创建安全的加密系统,非对称加密通常是解决方案。它使用两个密钥:公钥用于加密,私钥用于解密。此设置无需共享单个密钥即可实现安全通信。
它是如何运作的
密钥对生成
生成公钥-私钥对。公钥是公开共享的,而私钥则是保密的。加密
接收者的公钥对数据进行加密。只有他们的私钥才能解密,即使被拦截也能保证数据的安全。解密
接收者使用其私钥解密数据。
const crypto = require('crypto'); const algorithm = 'aes-256-cbc'; const key = crypto.randomBytes(32); const iv = crypto.randomBytes(16); function encrypt(text) { const cipher = crypto.createCipheriv(algorithm, key, iv); let encrypted = cipher.update(text, 'utf8', 'hex'); encrypted += cipher.final('hex'); return { encrypted, iv: iv.toString('hex'), key: key.toString('hex') }; } function decrypt(encrypted, ivHex, keyHex) { const decipher = crypto.createDecipheriv(algorithm, Buffer.from(keyHex, 'hex'), Buffer.from(ivHex, 'hex')); let decrypted = decipher.update(encrypted, 'hex', 'utf8'); decrypted += decipher.final('utf8'); return decrypted; } // Usage const message = "Secret Message"; const encryptedData = encrypt(message); console.log("Encrypted:", encryptedData); const decryptedMessage = decrypt(encryptedData.encrypted, encryptedData.iv, encryptedData.key); console.log("Decrypted:", decryptedMessage);
密码学中的散列
哈希将数据转换为固定长度、不可逆的字符串(哈希)。它通常用于验证数据完整性和安全存储密码。
流行的哈希算法:
- SHA-256:安全且广泛使用。
- SHA-3:较新且增强的安全性。
- MD5 和 SHA-1:由于漏洞而已弃用。
Node.js 中对字符串进行哈希处理的示例
const crypto = require('crypto'); // Generate keys const { publicKey, privateKey } = crypto.generateKeyPairSync('rsa', { modulusLength: 2048 }); const data = "Confidential Data"; // Encrypt const encrypted = crypto.publicEncrypt(publicKey, Buffer.from(data)); console.log("Encrypted:", encrypted.toString('base64')); // Decrypt const decrypted = crypto.privateDecrypt(privateKey, encrypted); console.log("Decrypted:", decrypted.toString());
加密与散列
Feature | Encryption | Hashing |
---|---|---|
Process | Two-way (encrypt/decrypt) | One-way |
Purpose | Data confidentiality | Data integrity |
Reversible | Yes | No |
Example | AES, RSA | SHA-256, bcrypt |
实例:项目中的非对称加密
在我的 Whisper 项目中,我们使用非对称加密来保护匿名聊天消息。消息使用收件人的公钥进行加密,确保只有收件人才能使用其私钥对其进行解密。
对于客户端React实现,我们使用crypto-js进行加密和解密:
const crypto = require('crypto'); const algorithm = 'aes-256-cbc'; const key = crypto.randomBytes(32); const iv = crypto.randomBytes(16); function encrypt(text) { const cipher = crypto.createCipheriv(algorithm, key, iv); let encrypted = cipher.update(text, 'utf8', 'hex'); encrypted += cipher.final('hex'); return { encrypted, iv: iv.toString('hex'), key: key.toString('hex') }; } function decrypt(encrypted, ivHex, keyHex) { const decipher = crypto.createDecipheriv(algorithm, Buffer.from(keyHex, 'hex'), Buffer.from(ivHex, 'hex')); let decrypted = decipher.update(encrypted, 'hex', 'utf8'); decrypted += decipher.final('utf8'); return decrypted; } // Usage const message = "Secret Message"; const encryptedData = encrypt(message); console.log("Encrypted:", encryptedData); const decryptedMessage = decrypt(encryptedData.encrypted, encryptedData.iv, encryptedData.key); console.log("Decrypted:", decryptedMessage);
解密使用私钥:
const crypto = require('crypto'); // Generate keys const { publicKey, privateKey } = crypto.generateKeyPairSync('rsa', { modulusLength: 2048 }); const data = "Confidential Data"; // Encrypt const encrypted = crypto.publicEncrypt(publicKey, Buffer.from(data)); console.log("Encrypted:", encrypted.toString('base64')); // Decrypt const decrypted = crypto.privateDecrypt(privateKey, encrypted); console.log("Decrypted:", decrypted.toString());
探索 Whisper 的代码以获取详细示例。
结论
密码学增强了应用程序中的数据安全性。对于共享密钥场景使用 AES 等对称加密,对于公私密钥系统使用非对称加密。散列可确保数据完整性,尤其是密码。根据您的应用程序的需求选择正确的加密方法。
需要更多知识吗?
了解有关共享密钥的更多信息
了解有关公钥的更多信息
了解有关 SHA-256 的更多信息
了解有关 SHA-3 的更多信息
了解有关 MD5 的更多信息
了解有关 SHA-1 的更多信息
阅读有关对称加密的更多信息
了解有关 AES 的更多信息
感谢您的阅读,请告诉我您对此的看法,如果您想了解更多,如果您认为我犯了错误或遗漏了某些内容,请随时发表评论
以上是JavaScript 中的密码学:实用指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
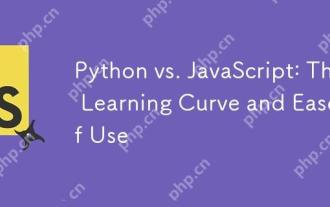
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
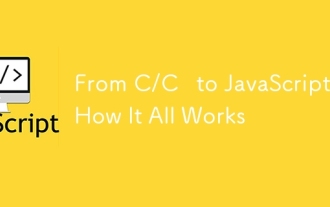
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
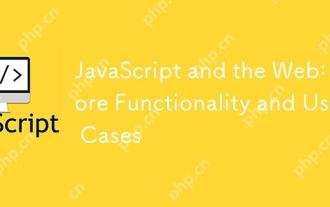
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
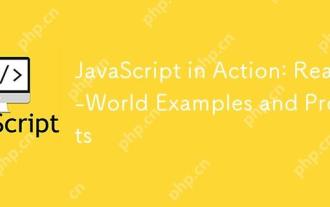
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
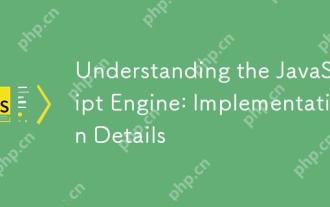
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
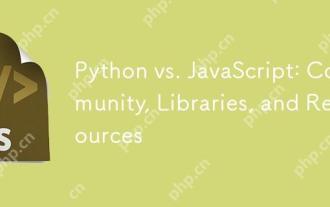
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
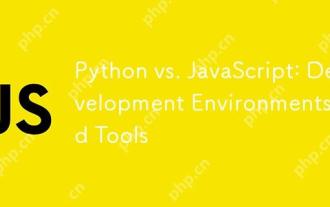
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
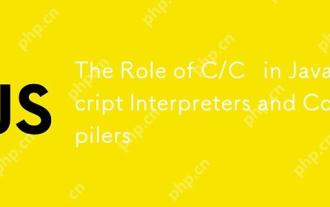
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
