如何将多个值从 Go 模板传递到嵌套模板?
将多个值从模板传递到模板
在 Go 模板中,可以使用 {{模板}} 操作。但是,此操作仅接受单个数据值作为输入。当需要将多个数据实体传递给嵌套模板时,需要一个解决方案。
用于数据打包的自定义函数
Go 的模板系统允许使用以下方式注册自定义函数Template.Funcs() 方法。这些函数可以在数据传递到模板之前对数据进行操作,从而实现数据操作和打包。
在需要将多个值传递给模板的情况下,可以创建一个自定义函数来包装将这些值放入单个实体中,例如映射或结构。然后可以将此包装的实体传递给 {{template}} 操作。
模板修改
定义自定义函数后,可以修改模板以调用此函数并将包装的数据实体传递给嵌套模板。其语法为:
{{template "templateName" (customFunctionName data1 data2)}}
示例
考虑以下城市和地区结构:
type City struct { ID int Name string Regions []Region } type Region struct { ID int Name string Shops []Destination Masters []Master EducationCenters []Destination }
传递多个数据实体对于嵌套模板,自定义函数 Wrap() 可以定义为如下:
func Wrap(shops []Destination, cityName, regionName string) map[string]interface{} { return map[string]interface{}{ "Shops": shops, "CityName": cityName, "RegionName": regionName, } }
此函数将商店数组以及城市和地区名称包装到地图中。修改后的模板现在如下所示:
const src = ` {{define "data"}} City: {{.CityName}}, Region: {{.RegionName}}, Shops: {{.Shops}} {{end}} {{- range . -}} {{$city:=.Name}} {{- range .Regions -}} {{$region:=.Name}} {{- template "data" (Wrap .Shops $city $region) -}} {{end}} {{- end}}`
可运行示例
以下代码演示了如何在实践中使用此解决方案:
package main import ( "os" "text/template" ) type City struct { ID int Name string Regions []Region } type Region struct { ID int Name string Shops []Destination Masters []Master EducationCenters []Destination } type Destination struct { Name string } func main() { t := template.Must(template.New("cities.gohtml").Funcs(template.FuncMap{ "Wrap": Wrap, }).Parse(src)) CityWithSomeData := []City{ { Name: "CityA", Regions: []Region{ {Name: "CA-RA", Shops: []Destination{{"CA-RA-SA"}, {"CA-RA-SB"}}}, {Name: "CA-RB", Shops: []Destination{{"CA-RB-SA"}, {"CA-RB-SB"}}}, }, }, { Name: "CityB", Regions: []Region{ {Name: "CB-RA", Shops: []Destination{{"CB-RA-SA"}, {"CB-RA-SB"}}}, {Name: "CB-RB", Shops: []Destination{{"CB-RB-SA"}, {"CB-RB-SB"}}}, }, }, } if err := t.ExecuteTemplate(os.Stdout, "cities.gohtml", CityWithSomeData); err != nil { panic(err) } } const src = ` {{define "data"}} City: {{.CityName}}, Region: {{.RegionName}}, Shops: {{.Shops}} {{end}} {{- range . -}} {{$city:=.Name}} {{- range .Regions -}} {{$region:=.Name}} {{- template "data" (Wrap .Shops $city $region) -}} {{end}} {{- end}}`
此代码演示了如何使用自定义函数将多个数据实体成功传递到嵌套模板。
以上是如何将多个值从 Go 模板传递到嵌套模板?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
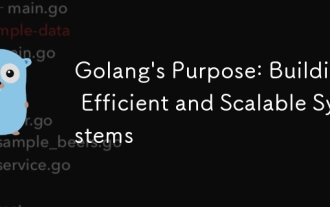
Go语言在构建高效且可扩展的系统中表现出色,其优势包括:1.高性能:编译成机器码,运行速度快;2.并发编程:通过goroutines和channels简化多任务处理;3.简洁性:语法简洁,降低学习和维护成本;4.跨平台:支持跨平台编译,方便部署。
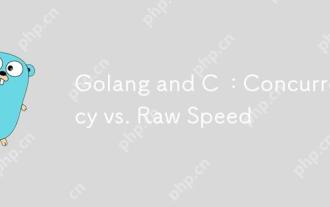
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
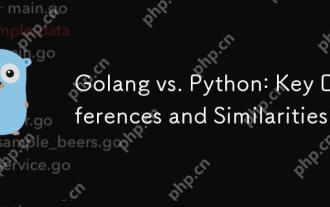
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
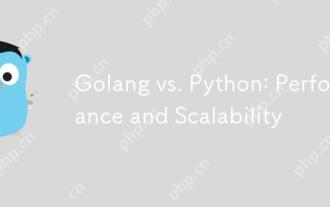
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
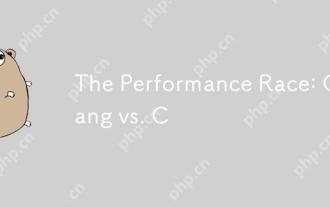
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
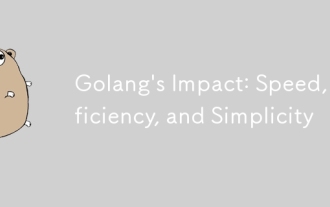
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
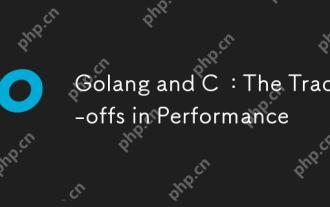
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
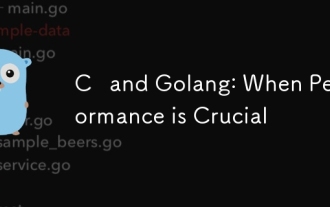
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
