掌握 JavaScript 事件委托
在现代 JavaScript 开发中,事件处理在使 Web 应用程序具有交互性和动态性方面发挥着至关重要的作用。随着应用程序的增长,管理事件侦听器的复杂性也随之增加。输入事件委托,这是一种强大的模式,可以利用JavaScript的事件传播系统来优化事件处理。
什么是事件委托?
事件委托是一种将单个事件侦听器附加到父元素以管理其子元素上的事件的技术。父级不是为每个子级添加单独的侦听器,而是捕获冒泡事件并识别交互源。
它是如何工作的?
事件委托依赖于两个关键的 JavaScript 机制:
事件冒泡:事件从目标元素传播到 DOM 树的根。
event.target: 标识事件的原始元素。
活动委托的优点
Feature | Explanation |
---|---|
Performance | Reduces the number of event listeners, saving memory and improving efficiency. |
Control Mechanism | Automatically manages dynamically added elements without additional listeners. |
Memory Handling | Centralized event handling logic in fewer places in the code. |
Common Use Cases | Supported universally across modern browsers. |
深入研究事件传播
JavaScript 事件在 DOM 中遵循可预测的生命周期。了解这些阶段对于掌握委派至关重要:
1.捕获阶段:事件从根开始,向下遍历到目标元素。
2.目标阶段: 事件在目标元素上激活。
3.气泡阶段:事件传播回根。
事件委托主要在冒泡阶段工作。
代码示例:实践中的事件委托
场景 1:管理列表的点击事件
而不是向每个列表项添加侦听器:
const ul = document.querySelector("ul"); ul.addEventListener("click", (event) => { if (event.target.tagName === "LI") { console.log("Clicked item:", event.target.textContent); } });
这个单一侦听器管理所有 li 元素,甚至是动态添加的元素:
const ul = document.querySelector("ul"); ul.innerHTML += "<li>New Item</li>"; // No new listener required.
场景 2:委托多个事件类型
将事件委托与事件类型检查相结合:
document.querySelector("#container").addEventListener("click", (event) => { if (event.target.matches(".button")) { console.log("Button clicked"); } else if (event.target.matches(".link")) { console.log("Link clicked"); } });
场景3:委托处理表格
document.querySelector("#form").addEventListener("input", (event) => { if (event.target.matches("input[name='email']")) { console.log("Email updated:", event.target.value); } else if (event.target.matches("input[name='password']")) { console.log("Password updated."); } });
此方法可确保自动处理动态添加的任何新输入字段。
活动委托的最佳实践
1。使用特定选择器: 避免广泛匹配以防止意外行为。使用 event.target.matches() 或 event.target.closest()。
2.避免过度委派:如果父级包含大量子级,则将太多事件委派给父级可能会变得低效。
3.优化条件逻辑:构建条件以尽量减少不必要的检查。
4.限制或反跳事件: 对于滚动或调整大小等事件,使用限制来增强性能:
function throttle(callback, delay) { let lastTime = 0; return function (...args) { const now = Date.now(); if (now - lastTime >= delay) { callback(...args); lastTime = now; } }; } document.addEventListener("scroll", throttle(() => console.log("Scrolled!"), 200));
事件委托与直接事件处理
Aspect | Direct Event Handling | Event Delegation |
---|---|---|
Setup Complexity | Requires multiple listeners. | Single listener handles multiple events. |
Dynamic Elements | Requires manual re-attachment. | Automatically supported. |
Performance in Large DOM | Degrades as the number of listeners grows. | Efficient with a centralized listener. |
Maintainability | Scattered logic across multiple places. | Centralized and clean. |
框架中的事件委托
反应
虽然 React 抽象了 DOM 操作,但您可以在合成事件中看到等效的委托。 React 使用单个根侦听器来管理其虚拟 DOM 中的所有事件。
jQuery
jQuery 的 .on() 方法简化了委托:
const ul = document.querySelector("ul"); ul.addEventListener("click", (event) => { if (event.target.tagName === "LI") { console.log("Clicked item:", event.target.textContent); } });
事件委托中的常见陷阱
1.意外比赛
确保您的选择器不会意外匹配不相关的元素。使用特定选择器或 event.target.closest().
2.防止事件冒泡
在某些情况下,您可能需要停止特定元素的冒泡:
const ul = document.querySelector("ul"); ul.innerHTML += "<li>New Item</li>"; // No new listener required.
性能考虑因素
1.基准
事件委托减少了大型 DOM 中的内存使用量,但如果父进程处理太多事件,可能会引入延迟。
2.DevTools
使用浏览器开发者工具分析附加的侦听器(Chrome 控制台中的 getEventListeners):
document.querySelector("#container").addEventListener("click", (event) => { if (event.target.matches(".button")) { console.log("Button clicked"); } else if (event.target.matches(".link")) { console.log("Link clicked"); } });
提示和技巧
- 在非冒泡事件中模拟委托: 有些事件,例如焦点和模糊,不会冒泡。使用 focusin 和 focusout 代替:
document.querySelector("#form").addEventListener("input", (event) => { if (event.target.matches("input[name='email']")) { console.log("Email updated:", event.target.value); } else if (event.target.matches("input[name='password']")) { console.log("Password updated."); } });
- 在根级别附加委托: 为了获得 SPA 或动态内容的最大灵活性,请将侦听器附加到文档。
结论
JavaScript 事件委托 是一种关键的优化策略,可以有效地扩展交互式应用程序。通过集中事件处理、减少内存使用和提高可维护性,它使开发人员能够构建健壮且高性能的 Web 应用程序。
我的网站:https://shafayet.zya.me
给你的模因(也许相关......)??
以上是掌握 JavaScript 事件委托的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
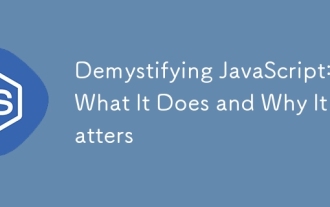
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
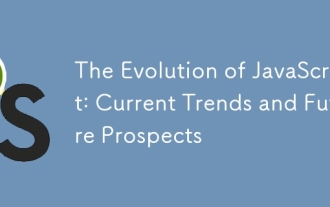
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
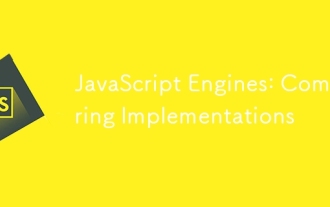
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
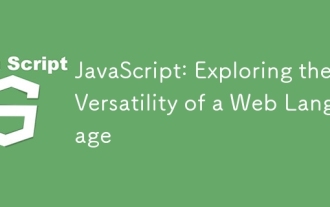
JavaScript是现代Web开发的核心语言,因其多样性和灵活性而广泛应用。1)前端开发:通过DOM操作和现代框架(如React、Vue.js、Angular)构建动态网页和单页面应用。2)服务器端开发:Node.js利用非阻塞I/O模型处理高并发和实时应用。3)移动和桌面应用开发:通过ReactNative和Electron实现跨平台开发,提高开发效率。
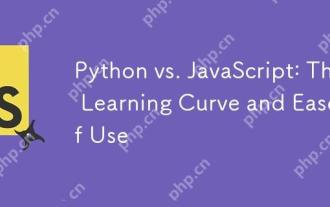
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
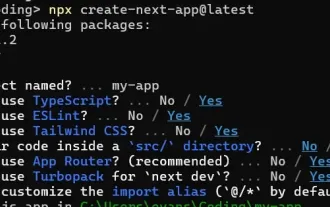
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库
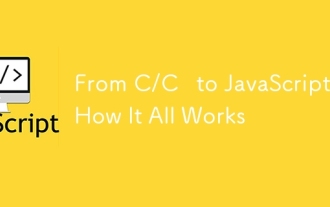
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
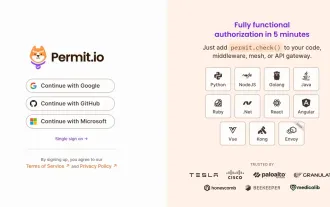
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
