保护您的 Node.js 应用程序:综合指南
在当今的数字环境中,保护 Node.js 应用程序的安全至关重要。从 Netflix 和 Uber 等全球领导者,到构建下一个伟大事物的初创公司,Node.js 为一些要求最苛刻的高性能应用程序提供支持。然而,应用程序中的漏洞可能会导致未经授权的访问、数据泄露和用户信任的丧失。
本指南将实用的安全实践与 OWASP Web 安全测试指南 (WSTG) 中的关键概念相结合,帮助您强化 Node.js 应用程序。无论您是管理实时操作还是扩展到数百万用户,这一全面的资源都将确保您的应用程序保持安全、可靠和弹性。
信息收集(WSTG-INFO)
信息收集通常是攻击者了解有关您的应用程序的更多信息的第一步。他们收集的信息越多,他们就越容易识别和利用漏洞。
典型 Express.js 服务器配置和指纹识别
默认情况下,Express.js 包含可能会无意中泄露有关您的服务器的信息的设置。一个常见的示例是 X-Powered-By HTTP 标头,它表明您的应用程序正在使用 Express。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
在此设置中,每个 HTTP 响应都包含 X-Powered-By: Express 标头。
问题:
- 指纹识别:攻击者可以使用此标头来确定您正在使用的技术。了解您正在运行 Express 后,他们可以针对特定版本的 Express 或 Node.js 中的已知漏洞定制攻击。
缓解措施:
禁用此标头以使攻击者更难识别您的服务器。
改进的代码:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
佩戴头盔增强缓解效果:
更好的方法是使用头盔中间件,它设置各种 HTTP 标头来提高应用程序的安全性。
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
为什么要戴头盔?
- 全面的安全标头: Helmet 设置多个 HTTP 标头,有助于保护您的应用程序免受众所周知的 Web 漏洞的影响。
- 易于使用:只需一行代码,即可显着增强应用程序的安全状况。
配置和部署管理测试 (WSTG-CONF)
配置和部署管理是应用程序安全的关键方面。错误配置可能为攻击者敞开大门。
在生产中以开发模式运行
在生产服务器上以开发模式运行应用程序可能会暴露详细的错误消息和堆栈跟踪。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
在此设置中,详细的错误消息将发送至客户端。
问题:
- 信息泄漏:详细的错误消息和堆栈跟踪可以泄露有关应用程序结构、依赖项和文件路径的敏感信息。
- 促进利用:攻击者可以使用此信息来识别潜在的漏洞并发起有针对性的攻击。
缓解措施:
将 NODE_ENV 设置为“生产”并在生产中使用通用错误消息。
改进的代码:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
最佳实践:
- 正确设置环境变量:确保生产环境中的 NODE_ENV 设置为“生产”。
- 内部日志记录:内部记录错误以用于调试目的,而不向最终用户公开详细信息。
使用默认或弱凭据
使用默认或弱凭据,例如用于签名 JSON Web 令牌 (JWT) 的简单密钥,是一种常见的安全错误。
易受攻击的代码示例:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 弱密钥:使用简单或常见的字符串(如“secret”)使攻击者很容易猜测或暴力破解密钥。
- 硬编码秘密:如果您的代码库遭到破坏,直接在代码中存储秘密会增加暴露的风险。
- 令牌伪造:知道您密钥的攻击者可以伪造有效的 JWT,从而获得未经授权的访问。
缓解措施:
使用强大、安全的密钥并安全存储。
改进的代码:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
最佳实践:
- 环境变量: 不要将机密提交给版本控制。使用未签入源代码管理的环境变量或配置文件。
- 轮换秘密:实施定期轮换秘密的流程。
- 验证配置:确保在应用程序启动期间设置所有必需的环境变量。
身份管理测试 (WSTG-IDNT)
身份管理对于保护用户帐户和防止未经授权的访问至关重要。
薄弱的用户名策略和帐户枚举
允许弱用户名并提供特定的错误消息可能会导致帐户枚举攻击。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 弱用户名:允许简短的用户名会增加帐户泄露的风险。
- 帐户枚举:特定的错误消息可以帮助攻击者确定有效的用户名。
缓解措施:
实施用户名验证并使用通用错误消息。
改进的代码:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 用户名验证:确保用户名符合特定标准,减少弱条目。
- 通用错误消息:防止攻击者通过错误响应识别有效用户名。
身份验证测试 (WSTG-ATHN)
身份验证机制对于验证用户身份和防止未经授权的访问至关重要。
对密码和 2FA 的暴力攻击
缺乏保护,攻击者可以通过反复尝试猜测密码或 2FA 代码。
易受攻击的代码示例:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 无限制的登录尝试:攻击者可以重复尝试不同的密码或 2FA 代码。
- 薄弱的 2FA 实施:静态或可预测的 2FA 代码容易受到攻击。
缓解措施:
实施速率限制并增强 2FA 安全性。
改进的代码:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
额外措施:
- 尝试失败后使用验证码:在多次登录尝试失败后引入验证码来验证人类用户。
- 采用 TOTP 进行 2FA: 使用基于时间的一次性密码来实现动态且安全的 2FA 代码。
说明:
- 速率限制:通过限制登录尝试来降低自动攻击风险。
- 增强的 2FA: 基于时间的代码比静态代码提高了安全性。
授权测试(WSTG-ATHZ)
授权确保用户仅访问他们有权使用的资源,防止未经授权的操作。
不安全的直接对象引用 (IDOR)
用户可以通过操纵请求中的标识符来访问未经授权的资源。
易受攻击的代码示例:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
问题:
- 未授权访问:用户可以通过修改orderId参数来访问不该访问的数据。
缓解措施:
在提供访问权限之前验证资源所有权。
改进的代码:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 所有权验证: 确保请求的资源属于经过身份验证的用户。
- 访问控制:防止用户通过操纵请求参数来访问他人的数据。
会话管理测试 (WSTG-SESS)
会话管理对于维护用户状态和确保安全交互至关重要。
没有过期时间的代币
永不过期的令牌如果被泄露就会带来安全风险。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 持久令牌:没有过期的令牌无限期地保持有效,增加了滥用的机会。
缓解措施:
设置令牌的过期时间。
改进的代码:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 令牌过期:限制有效期,降低令牌被泄露的风险。
- 安全最佳实践:定期令牌更新可增强整体安全性。
不安全的令牌存储
将令牌存储在 localStorage 中会使它们遭受跨站脚本 (XSS) 攻击。
易受攻击的代码示例:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
问题:
- 客户端暴露:恶意脚本可以访问 localStorage、窃取令牌和劫持会话。
缓解措施:
使用仅 HTTP 的 cookie 来安全地存储令牌。
改进的代码:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
说明:
- 仅限 HTTP 的 Cookie: JavaScript 无法访问,从而降低 XSS 风险。
- 安全和 SameSite 标志: 增强对中间人和跨站点请求伪造攻击的防护。
输入验证测试 (WSTG-INPV)
输入验证确保用户提供的数据安全且符合预期,防止注入攻击。
缺乏输入验证
未经验证而接受和处理用户输入可能会导致漏洞。
易受攻击的代码示例:
const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); // Weak secret key const SECRET_KEY = 'secret'; app.post('/login', (req, res) => { // Authenticate user (authentication logic not shown) const userId = req.body.userId; // Sign the JWT with a weak secret const token = jwt.sign({ userId }, SECRET_KEY); res.json({ token }); }); app.get('/protected', (req, res) => { const token = req.headers['authorization']; try { // Verify the token using the weak secret const decoded = jwt.verify(token, SECRET_KEY); res.send('Access granted to protected data'); } catch (err) { res.status(401).send('Unauthorized'); } }); app.listen(3000, () => { console.log('Server started on port 3000'); });
问题:
- 注入攻击:未经验证的输入可能导致 SQL 注入、NoSQL 注入或其他代码注入攻击。
缓解措施:
验证并清理所有用户输入。
改进的代码:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 输入验证: 检查输入是否符合预期标准。
- 输入清理: 删除或转义潜在有害字符。
- 安全数据库查询:使用参数化查询可以防止注入攻击。
错误处理测试 (WSTG-ERRH)
正确的错误处理可以避免泄露敏感信息并改善用户体验。
暴露敏感错误信息
详细的错误消息可以向攻击者揭示系统内部结构。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 信息泄露:攻击者可以深入了解您的应用程序的结构和潜在漏洞。
缓解措施:
使用通用错误消息并在内部记录详细错误。
改进的代码:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 内部日志记录: 确保详细错误信息的安全。
- 用户友好的消息:提供通用消息,而不泄露敏感细节。
弱加密测试 (WSTG-CRYP)
密码学保护敏感数据;使用弱加密实践会破坏安全性。
使用不安全的哈希算法
使用过时的算法对密码进行哈希处理是不安全的。
易受攻击的代码示例:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
问题:
- 弱哈希: MD5 和 SHA-1 等算法容易受到碰撞攻击,不应该用于密码哈希。
缓解措施:
使用专为密码设计的强大哈希算法。
改进的代码:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
说明:
- Bcrypt: 一个强大的哈希函数,结合了加盐和多轮哈希。
- 密码安全: 使攻击者在计算上无法对密码进行逆向工程。
硬编码密钥
直接在代码中存储秘密会增加暴露的风险。
易受攻击的代码示例:
const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); // Weak secret key const SECRET_KEY = 'secret'; app.post('/login', (req, res) => { // Authenticate user (authentication logic not shown) const userId = req.body.userId; // Sign the JWT with a weak secret const token = jwt.sign({ userId }, SECRET_KEY); res.json({ token }); }); app.get('/protected', (req, res) => { const token = req.headers['authorization']; try { // Verify the token using the weak secret const decoded = jwt.verify(token, SECRET_KEY); res.send('Access granted to protected data'); } catch (err) { res.status(401).send('Unauthorized'); } }); app.listen(3000, () => { console.log('Server started on port 3000'); });
问题:
- 秘密曝光:如果代码库遭到破坏,可以轻松提取硬编码的秘密。
缓解措施:
将机密存储在环境变量或安全配置文件中。
改进的代码:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 环境变量:对代码库和版本控制系统保密。
- 安全实践:降低意外暴露的风险。
业务逻辑测试 (WSTG-BUSL)
业务逻辑当应用程序流被以意想不到的方式操纵时,就会出现漏洞。
滥用批量操作
不受限制的数据操作可能会导致性能问题或数据泄漏。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 拒绝服务 (DoS): 大量数据导出会耗尽服务器资源。
- 数据泄露:不受限制的访问可能会泄露敏感信息。
缓解措施:
实施分页和访问控制。
改进的代码:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 分页:控制返回的数据量,防止资源耗尽。
- 访问控制:确保用户只能访问自己的数据。
客户端测试 (WSTG-CLNT)
防范客户端漏洞对于保护用户免受跨站脚本 (XSS) 等攻击至关重要。
使用 xss 库转义用户输入
客户端脚本中对用户输入的不当处理可能会导致 XSS 攻击。
易受攻击的代码示例:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
问题:
- 不安全的 DOM 操作: 将未经净化的用户输入插入到 innerHTML 中会允许执行恶意脚本。
缓解措施:
使用 xss 库在渲染之前清理用户输入。
改进的代码:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
说明:
- 输入清理: xss 库通过转义或删除潜在危险内容来清理输入。
- 防止脚本执行:中和恶意脚本,防止它们在浏览器中执行。
最佳实践:
- 尽可能使用textContent:将用户输入分配给textContent会将其视为纯文本。
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
- 结合客户端和服务器端验证:深度防御方法可增强安全性。
API 测试 (WSTG-APIT)
保护 API 端点的安全对于防止数据泄露和未经授权的访问至关重要。
GraphQL 自省曝光
在生产环境中启用 GraphQL 自省会暴露您的 API 模式。
易受攻击的代码示例:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
问题:
- 架构泄露:攻击者可以探索您的 API 架构,帮助发起有针对性的攻击。
缓解措施:
在生产环境中禁用自省。
改进的代码:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
说明:
- 条件自省: 允许在开发过程中自省,但在生产中禁用它。
- 安全增强:通过隐藏架构详细信息来减少攻击面。
不受限制的查询复杂性
深度嵌套或复杂的查询会耗尽服务器资源。
易受攻击的代码示例:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
问题:
- 拒绝服务 (DoS): 复杂的查询可能会导致 CPU 和内存使用率较高。
缓解措施:
限制查询深度和复杂性。
改进的代码:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
说明:
- 深度限制:限制查询的深度,防止资源耗尽。
- 性能保护: 确保 API 保持响应和可用。
结论
保护 Node.js 应用程序涉及多层方法:
- 防止信息泄露:清理代码和服务器配置以避免暴露敏感数据。
- 安全地管理配置:删除默认凭据和安全配置文件。
- 验证和清理输入:永远不要相信用户输入。
- 实施适当的身份验证和授权:确保用户拥有适当的访问权限。
- 使用强加密技术:使用安全算法和密钥管理来保护数据。
- 优雅地处理错误:避免泄露敏感信息。
- 保护客户端交互:减轻 XSS 和其他基于浏览器的攻击。
- 安全 API: 控制数据暴露并实施速率限制。
进一步阅读
- OWASP 网络安全测试指南 (WSTG): OWASP WSTG
- Node.js 安全指南: Node.js 安全
- Express.js 安全提示: Express 安全最佳实践
- GraphQL 安全最佳实践: Apollo GraphQL 安全
- OWASP 前十名:OWASP 前十名
- MDN Web 文档 - 网络安全: MDN Web 安全
注意:本指南提供一般建议。对于特定的安全问题,请咨询专业人士。
以上是保护您的 Node.js 应用程序:综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
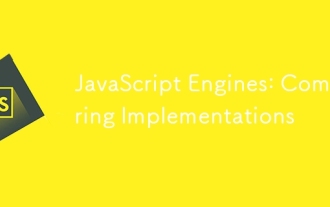
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
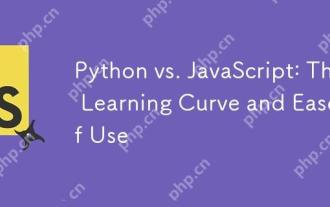
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
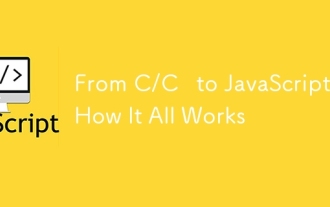
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
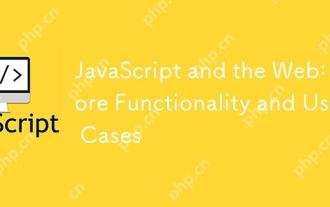
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
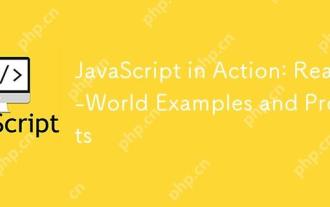
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
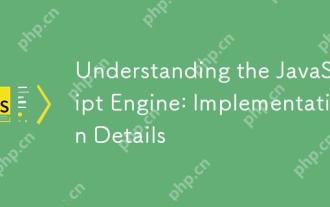
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
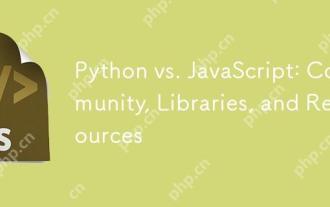
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
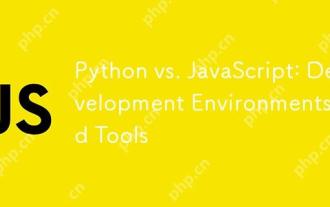
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
