使用 Response 将 FormData 转换为 multipart/form-data 字符串并返回
在撰写另一篇有关将各种值转换为 ReadableStream 的文章时,我偶然发现了一个巧妙的技巧,可以将 FormData 转换为多部分/表单数据字符串,然后使用 Response 将其转换回来。令人惊讶的是,FormData 并未立即提供此功能。然而,通过利用 Response 的方法,我们可以很容易地实现这一点。
我什至尝试从 ChatGPT(4o 和 o1-preview)获得帮助,但即使有提示,它也无法建议或综合此解决方案。谷歌搜索也没有产生任何结果。尽管我不声称具有独创性,但这种方法似乎是未知的或鲜为人知的。我不认为这种转换的能力是 Response API 的作者有意设计的;它更多的是一个副作用,这使得解决方案更加有趣。因此,我决定写这篇文章来分享我的发现。
将 FormData 转换为多部分/表单数据字符串
假设我们有一个 FormData 实例:
const formData = new FormData(); formData.set('value', 123); formData.set( 'myfile', new File(['{"hello":"world"}'], 'demo.json', { type: 'application/json' }) );
我们的目标是从此 FormData 实例获取 multipart/form-data 字符串。常见的建议包括手动将 FormData 序列化为字符串或通过 Request 执行复杂的操作,或使用库。但是,我们可以简单地使用 Response 构造函数,它接受 FormData 作为正文,然后调用其 text() 方法来获取所需的字符串表示形式:
// FormData -> multipart/form-data string function convertFormDataToMultipartString(formData) { return new Response(formData).text(); } const multipartString = await convertFormDataToMultipartString(formData); console.log(multipartString); // Example output: // ------WebKitFormBoundaryQi7NBNu0nAmyAhpU // Content-Disposition: form-data; name="value" // // 123 // ------WebKitFormBoundaryQi7NBNu0nAmyAhpU // Content-Disposition: form-data; name="myfile"; filename="demo.json" // Content-Type: application/json // // {"hello":"world"} // ------WebKitFormBoundaryQi7NBNu0nAmyAhpU--
如果需要,我们还可以提取其他值,例如 Content-Type 标头和边界:
// FormData -> multipart/form-data async function convertFormDataToMultipart(formData) { const response = new Response(formData); const contentType = response.headers.get('Content-Type'); const boundary = contentType.match(/boundary=(\S+)/)[1]; return { contentType, boundary, body: await response.text(), }; } const multipart = await convertFormDataToMultipart(formData); console.log(multipart); // { // contentType: 'multipart/form-data; boundary=----WebKitFormBoundarybfJIH5LgEGPqNcqt', // boundary: '----WebKitFormBoundarybfJIH5LgEGPqNcqt', // body: '------WebKitFormBoundarybfJIH5LgEGPqNcqt\r\n...' // }
除了使用 text() 之外,我们还可以使用 arrayBuffer() 等其他方法来获取 FormData 的编码内容作为 ArrayBuffer。这种类型的值的优点是它是一个可传输的对象,并且可以使用 postMessage() 在工作人员之间有效地传输(意味着无需复制,与字符串不同)。 FormData 对象本身是不可转让的。
将多部分/表单数据字符串转换回 FormData
为了反转该过程,我们再次使用 Response,但这次我们使用它的 formData() 方法。此方法的一个关键方面是它需要正确的 Content-Type 标头,其中必须包含 multipart/form-data 类型以及用于分隔各部分的边界。如果我们有边界值,我们可以将其插入到标头中。但是,我们可能只有多部分字符串而没有其他内容。在这种情况下,我们可以使用正则表达式从字符串中提取边界。
// multipart/form-data string -> FormData async function convertMultipartStringToFormData(multipartString) { const boundary = multipartString.match(/^\s*--(\S+)/)[1]; return new Response(multipartString, { headers: { // Without the correct header, the string won't be parsed, // and an exception will be thrown on formData() call 'Content-Type': 'multipart/form-data; boundary=' + boundary, }, }).formData(); } const restoredFormData = await convertMultipartStringToFormData(multipartString); console.log([...restoredFormData]); // [ // ['value', '123'], // ['myfile', File] // ]
就像序列化 FormData 一样,我们不仅可以将字符串转换为 FormData,还可以将 ArrayBuffer、TypedArray 和 ReadableStream 转换为 FormData,因为 Response 接受此类值作为正文值。
结论
一个潜在的缺点是这两种类型的转换都是异步操作。除此之外,该方法看起来相当可靠,可以用于调试或需要在 fetch() 之外进行转换时。
此方法具有广泛的浏览器支持(它应该适用于 2017 年之后发布的任何浏览器)。如果 Node.js、Deno 和 Bun 支持 Response 对象(即 fetch() 可用的版本),该方法也适用于它们。
我希望您和我一样觉得这种方法很有趣。这是一种简单而有效的方法,可利用 Response API 的功能将 FormData 转换为多部分/表单数据字符串(而不仅仅是字符串)并返回。如果您有任何想法或知道其他方法可以达到相同的结果,请随时在评论中分享!
以上是使用 Response 将 FormData 转换为 multipart/form-data 字符串并返回的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
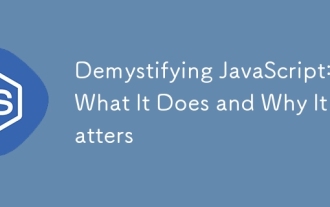
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
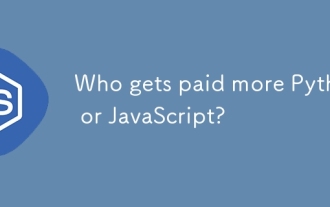
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
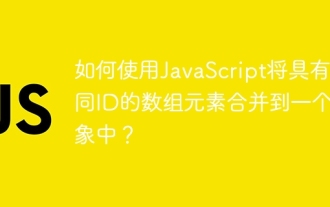
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
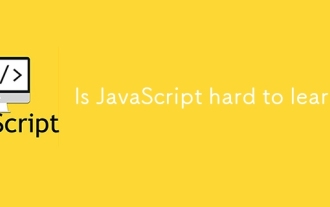
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
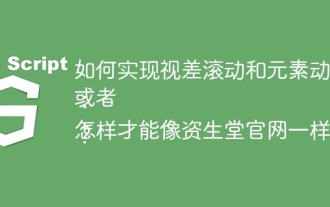
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
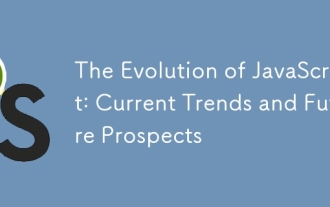
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
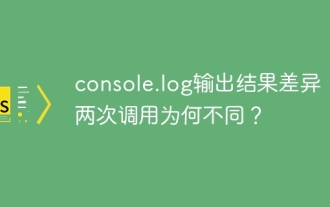
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
