用 Python 构建一个简单的 SQLite 库管理器
用 Python 构建一个简单的 SQLite 库管理器
高效管理数据是任何项目的关键部分,而 SQLite 使这项任务变得简单而轻量。在本教程中,我们将构建一个小型 Python 应用程序来管理图书馆数据库,使您可以轻松添加和检索书籍。
读完本文,您将了解如何:
- 创建 SQLite 数据库和表。
- 插入记录,同时防止重复。
- 根据特定条件检索数据。
1. 创建数据库和表
让我们首先创建 SQLite 数据库文件并定义 books 表。每本书都有标题、作者、ISBN、出版日期和流派字段。
import sqlite3 import os def create_library_database(): """Creates the library database if it doesn't already exist.""" db_name = "library.db" if not os.path.exists(db_name): print(f"Creating database: {db_name}") conn = sqlite3.connect(db_name) cursor = conn.cursor() cursor.execute(''' CREATE TABLE IF NOT EXISTS books ( id INTEGER PRIMARY KEY AUTOINCREMENT, title TEXT, author TEXT, isbn TEXT UNIQUE, published_date DATE, genre TEXT ) ''') conn.commit() conn.close() else: print(f"Database already exists: {db_name}")
运行此函数来初始化数据库:
create_library_database()
这将在您的项目目录中创建一个library.db 文件,其中包含具有指定字段的书籍表。
- 将书籍插入数据库
要插入书籍,我们希望确保避免重复条目(基于 isbn 字段)。我们将使用 SQLite 的 INSERT OR IGNORE 语句,而不是手动检查重复项。
这是添加书籍的功能:
def insert_book(book): """ Inserts a book into the database. If a book with the same ISBN already exists, the insertion is ignored. """ conn = sqlite3.connect("library.db") cursor = conn.cursor() try: # Insert the book. Ignore the insertion if the ISBN already exists. cursor.execute(''' INSERT OR IGNORE INTO books (title, author, isbn, published_date, genre) VALUES (?, ?, ?, ?, ?) ''', (book["title"], book["author"], book["isbn"], book["published_date"], book["genre"])) conn.commit() if cursor.rowcount == 0: print(f"The book with ISBN '{book['isbn']}' already exists in the database.") else: print(f"Book inserted: {book['title']} by {book['author']}") except sqlite3.Error as e: print(f"Database error: {e}") finally: conn.close()
此函数使用 INSERT OR IGNORE SQL 语句来确保有效地跳过重复条目。
3. 添加一些书籍
让我们通过向我们的图书馆添加一些书籍来测试 insert_book 函数。
books = [ { "title": "To Kill a Mockingbird", "author": "Harper Lee", "isbn": "9780061120084", "published_date": "1960-07-11", "genre": "Fiction" }, { "title": "1984", "author": "George Orwell", "isbn": "9780451524935", "published_date": "1949-06-08", "genre": "Dystopian" }, { "title": "Pride and Prejudice", "author": "Jane Austen", "isbn": "9781503290563", "published_date": "1813-01-28", "genre": "Romance" } ] for book in books: insert_book(book)
当您运行上述代码时,书籍将被添加到数据库中。如果您再次运行它,您将看到类似以下的消息:
The book with ISBN '9780061120084' already exists in the database. The book with ISBN '9780451524935' already exists in the database. The book with ISBN '9781503290563' already exists in the database.
4. 检索书籍
您可以通过查询数据库轻松检索数据。例如,要获取图书馆中的所有书籍:
def fetch_all_books(): conn = sqlite3.connect("library.db") cursor = conn.cursor() cursor.execute("SELECT * FROM books") rows = cursor.fetchall() conn.close() return rows books = fetch_all_books() for book in books: print(book)
结论
只需几行Python,您现在就拥有了一个功能齐全的图书馆管理器,可以插入书籍,同时防止重复并轻松检索记录。 SQLite 的 INSERT OR IGNORE 是一个强大的功能,可以简化处理约束,使您的代码更加简洁和高效。
随意扩展此项目,具有以下功能:
- 按书名或作者搜索书籍。
- 更新图书信息。
- 删除书籍。
接下来你会建造什么? ?
以上是用 Python 构建一个简单的 SQLite 库管理器的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
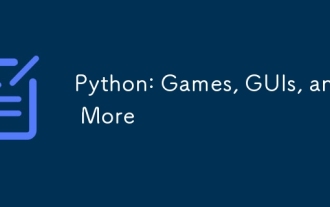
Python在游戏和GUI开发中表现出色。1)游戏开发使用Pygame,提供绘图、音频等功能,适合创建2D游戏。2)GUI开发可选择Tkinter或PyQt,Tkinter简单易用,PyQt功能丰富,适合专业开发。
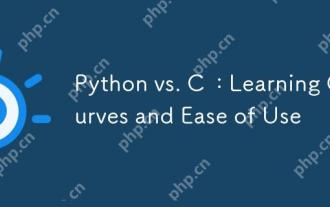
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
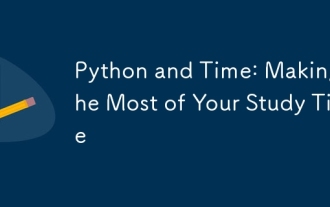
要在有限的时间内最大化学习Python的效率,可以使用Python的datetime、time和schedule模块。1.datetime模块用于记录和规划学习时间。2.time模块帮助设置学习和休息时间。3.schedule模块自动化安排每周学习任务。
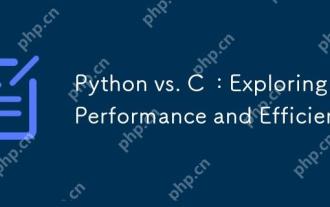
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
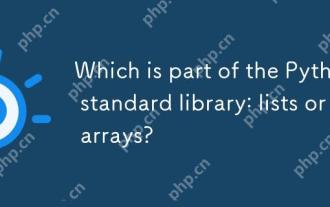
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
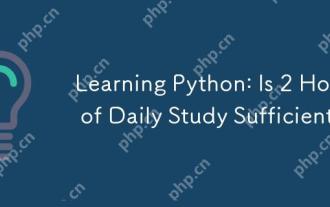
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
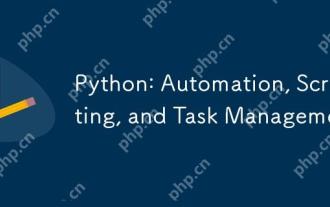
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
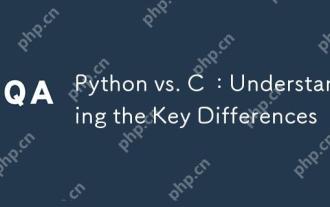
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
