Webpack新特性详解及性能优化实践
1. 长期缓存
Webpack 5 通过确定性的 Chunk ID、模块 ID 和导出 ID 实现长期缓存,这意味着相同的输入将始终产生相同的输出。这样,当您的用户再次访问更新后的网站时,浏览器可以重用旧的缓存,而不用重新下载所有资源。
// webpack.config.js module.exports = { // ... output: { // Use contenthash to ensure that the file name is associated with the content filename: '[name].[contenthash].js', chunkFilename: '[name].[contenthash].chunk.js', // Configure the asset hash to ensure long-term caching assetModuleFilename: '[name].[contenthash][ext][query]', // Use file system cache cache: { type: 'filesystem', }, }, // ... };
2. Tree Shaking 优化
Webpack 5 增强了 Tree Shaking 的效率,特别是对 ESM 的支持。
// package.json { "sideEffects": false, // Tell Webpack that this package has no side effects and can safely remove unreferenced code } // library.js export function myLibraryFunction() { // ... } // main.js import { myLibraryFunction } from './library.js';
3. 连接模块
Webpack 5 的 concatenateModules 选项可以组合小模块来减少 HTTP 请求的数量。不过这个功能可能会增加内存消耗,所以使用时需要权衡一下:
// webpack.config.js module.exports = { // ... optimization: { concatenateModules: true, // Defaults to true, but may need to be turned off in some cases }, // ... };
4. Node.js 模块 Polyfills 移除
Webpack 5 不再自动为 Node.js 核心模块注入 polyfill。开发者需要根据目标环境手动导入:
// If you need to be compatible with older browsers, you need to manually import polyfills import 'core-js/stable'; import 'regenerator-runtime/runtime'; // Or use babel-polyfill import '@babel/polyfill';
5. 性能优化实践
使用缓存:配置cache.type:'filesystem'使用文件系统缓存来减少重复构建。
SplitChunks 优化:根据项目需求调整 optimization.splitChunks,例如:
// webpack.config.js module.exports = { // ... optimization: { splitChunks: { chunks: 'all', minSize: 10000, // Adjust the appropriate size threshold maxSize: 0, // Allow code chunks of all sizes to be split }, }, // ... };
模块解析优化:通过resolve.mainFields和resolve.modules配置减少模块解析的开销。
并行编译:使用threads-loader或worker-loader并行处理任务,加快编译速度。
代码分割:使用动态导入(import())按需加载代码,减少初始加载时间。
// main.js import('./dynamic-feature.js').then((dynamicFeature) => { dynamicFeature.init(); });
- Module Federation:使用 webpack.container.module 配置实现跨应用的代码共享,减少重复打包。
// webpack.config.js module.exports = { // ... experiments: { outputModule: true, // Enable output module support }, // ... };
6、Tree Shaking的深入应用
虽然Webpack 5本身对Tree shake进行了优化,但开发者可以通过一些策略进一步提高其效果。确保您的代码遵循以下原则:
- 避免全局变量污染:全局变量可以防止 Tree shake 识别未使用的代码。
- 使用纯函数:确保函数没有副作用,以便Webpack可以安全地删除未调用的函数。
- 显式导出:使用显式导出(export const func = ... 而不是导出默认 func)有助于 Tree shake 更准确地工作。
- 死代码消除:结合ESLint的no-unused-vars规则,确保没有未使用的导入。
7.Loader和Plugin优化
- 减少 Loader 使用:每个 Loader 都会增加构建时间。仅在必要时使用Loaders,并考虑是否可以合并某些Loaders的功能。
- 加载器缓存:确保加载器支持并启用缓存,例如使用cacheDirectory选项。
- 选择高效的插件:有些插件可能对性能影响较大。评估并选择性能更好的替代方案,或调整其配置以减少开销。
8. Source Map策略
源映射对于调试至关重要,但它也会增加构建时间和输出大小。您可以根据环境调整Source Map类型:
// webpack.config.js module.exports = { // ... output: { // Use contenthash to ensure that the file name is associated with the content filename: '[name].[contenthash].js', chunkFilename: '[name].[contenthash].chunk.js', // Configure the asset hash to ensure long-term caching assetModuleFilename: '[name].[contenthash][ext][query]', // Use file system cache cache: { type: 'filesystem', }, }, // ... };
9. 图片及静态资源处理
- Asset Modules:Webpack 5引入了Asset Modules,可以直接处理图片等静态资源,无需额外配置Loader。此功能可以简化配置并提高性能。
// package.json { "sideEffects": false, // Tell Webpack that this package has no side effects and can safely remove unreferenced code } // library.js export function myLibraryFunction() { // ... } // main.js import { myLibraryFunction } from './library.js';
- 图像压缩和优化:使用 image-webpack-loader 等工具在构建过程中自动压缩图像,以减小文件大小。
10.持续监测和分析
- 使用Webpack Bundle Analyzer:这是一个强大的可视化工具,可以帮助您了解输出包的组成,识别大模块,然后对其进行优化。
- 定期检查依赖项:使用npmaudit或yarnaudit等工具检查依赖项的安全性和更新状态,及时删除不再使用的包或升级到更轻的替代方案。
以上是Webpack新特性详解及性能优化实践的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
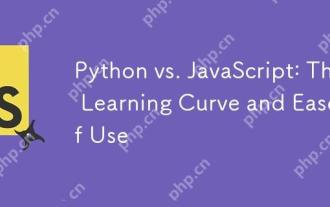
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
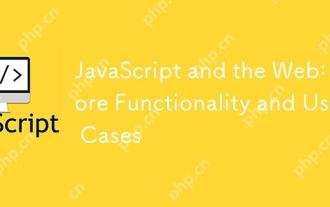
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
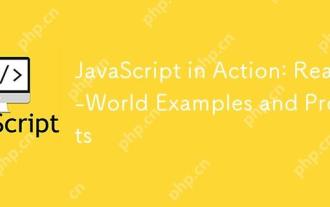
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
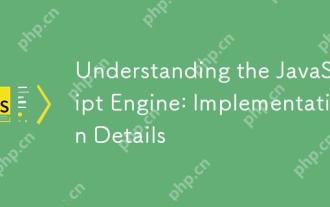
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
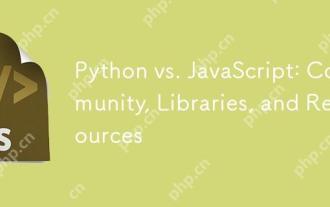
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
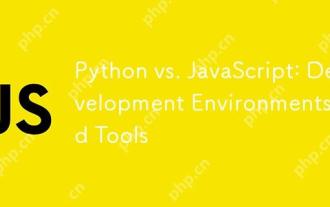
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
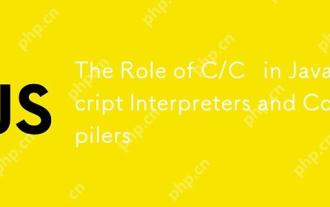
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。

Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
