了解 JavaScript 运算符:带有示例的完整指南
### JavaScript 中的运算符
JavaScript 中的运算符是用于对值和变量执行运算的特殊符号。这些操作可以涉及算术、赋值、比较、逻辑和其他操作。了解运算符对于执行基本计算、比较和控制代码流程至关重要。
JavaScript 支持多种运算符,它们分为以下类型:
### 1. **算术运算符**
算术运算符用于对数字进行数学计算。
Operator | Description | Example |
---|---|---|
Addition | 5 3 → 8 | |
- | Subtraction | 5 - 3 → 2 |
* | Multiplication | 5 * 3 → 15 |
/ | Division | 5 / 3 → 1.666... |
% | Modulus (Remainder) | 5 % 3 → 2 |
** | Exponentiation | 5 ** 2 → 25 |
例子:
let a = 10; let b = 2; console.log(a + b); // Output: 12 console.log(a - b); // Output: 8 console.log(a * b); // Output: 20 console.log(a / b); // Output: 5 console.log(a % b); // Output: 0 console.log(a ** b); // Output: 100
**
2. 赋值运算符**
赋值运算符用于为变量赋值。
Operator | Description | Example |
---|---|---|
= | Assign value | x = 5 |
= | Add and assign | x = 3 → x = x 3 |
-= | Subtract and assign | x -= 2 → x = x - 2 |
*= | Multiply and assign | x *= 4 → x = x * 4 |
/= | Divide and assign | x /= 2 → x = x / 2 |
%= | Modulus and assign | x %= 3 → x = x % 3 |
**= | Exponentiation and assign | x **= 2 → x = x ** 2 |
例子:
let a = 10; let b = 2; console.log(a + b); // Output: 12 console.log(a - b); // Output: 8 console.log(a * b); // Output: 20 console.log(a / b); // Output: 5 console.log(a % b); // Output: 0 console.log(a ** b); // Output: 100
### 3. **比较运算符**
比较运算符用于比较值并根据条件返回布尔值(true 或 false)。
Operator | Description | Example |
---|---|---|
== | Equal to (loose) | 5 == '5' → true |
=== | Equal to (strict) | 5 === '5' → false |
!= | Not equal to (loose) | 5 != '5' → false |
!== | Not equal to (strict) | 5 !== '5' → true |
> | Greater than | 5 > 3 → true |
< | Less than | 5 < 3 → false |
>= | Greater than or equal | 5 >= 5 → true |
<= | Less than or equal | 5 <= 3 → false |
例子:
let x = 10; x += 5; // x = x + 5 -> 15 x *= 2; // x = x * 2 -> 30 console.log(x); // Output: 30 <hr> <p><strong>### 4. **逻辑运算符</strong>**<br> 逻辑运算符用于执行逻辑运算,返回布尔值。</p> <div><table> <thead> <tr> <th>Operator</th> <th>Description</th> <th>Example</th> </tr> </thead> <tbody> <tr> <td>&&</td> <td>Logical AND</td> <td> true && false → false </td> </tr> <tr> <td>`</td> <td></td> <td>`</td> </tr> <tr> <td>!</td> <td>Logical NOT</td> <td> !true → false </td> </tr> </tbody> </table></div> <p><strong>#### 示例:</strong><br> </p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">console.log(5 == '5'); // Output: true (loose comparison) console.log(5 === '5'); // Output: false (strict comparison) console.log(10 > 5); // Output: true console.log(3 <= 2); // Output: false
### 5. **一元运算符**
一元运算符对单个操作数进行运算以执行特定操作。
Operator | Description | Example |
---|---|---|
Increment | x → x = x 1 | |
-- | Decrement | x-- → x = x - 1 |
typeof | Type of operand | typeof x → number |
void | Evaluates expression without returning a value | void(0) |
#### 示例:
let a = true; let b = false; console.log(a && b); // Output: false console.log(a || b); // Output: true console.log(!a); // Output: false
### 6. **三元(条件)运算符
**三元运算符是 if...else 语句的简写。它评估一个条件并根据条件是真还是假返回两个值之一。
Operator | Description | Example |
---|---|---|
condition ? expr1 : expr2 | If condition is true, return expr1; otherwise, return expr2 | x > 10 ? 'Greater' : 'Lesser' |
*#### 示例:
*
let a = 10; let b = 2; console.log(a + b); // Output: 12 console.log(a - b); // Output: 8 console.log(a * b); // Output: 20 console.log(a / b); // Output: 5 console.log(a % b); // Output: 0 console.log(a ** b); // Output: 100
### 7. **位运算符
**按位运算符对二进制数进行运算。
Operator | Description | Example |
---|---|---|
& | AND | 5 & 3 → 1 |
` | ` | OR |
^ | XOR | 5 ^ 3 → 6 |
~ | NOT | ~5 → -6 |
<< | Left shift | 5 << 1 → 10 |
>> | Right shift | 5 >> 1 → 2 |
>>> | Unsigned right shift | 5 >>> 1 → 2 |
*#### 示例:
*
let x = 10; x += 5; // x = x + 5 -> 15 x *= 2; // x = x * 2 -> 30 console.log(x); // Output: 30
### 8. **扩展运算符 (...)
**扩展运算符允许您将数组或对象中的元素解压到新的数组或对象中。
*#### 示例:
*
console.log(5 == '5'); // Output: true (loose comparison) console.log(5 === '5'); // Output: false (strict comparison) console.log(10 > 5); // Output: true console.log(3 <= 2); // Output: false
### 结论
JavaScript 运算符是执行计算、比较和逻辑运算的基础。无论您是操作值、比较它们还是控制程序流程,理解这些运算符对于高效编码都至关重要。根据您的任务使用正确的运算符,以确保代码干净且可读。
嗨,我是 Abhay Singh Kathayat!
我是一名全栈开发人员,拥有前端和后端技术方面的专业知识。我使用各种编程语言和框架来构建高效、可扩展且用户友好的应用程序。
请随时通过我的商务电子邮件与我联系:kaashshorts28@gmail.com。
以上是了解 JavaScript 运算符:带有示例的完整指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
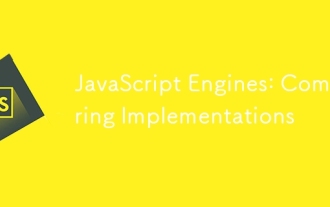
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
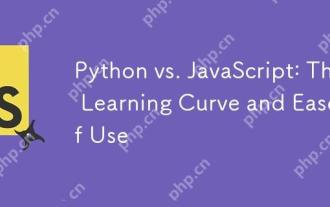
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
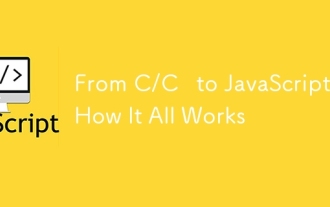
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
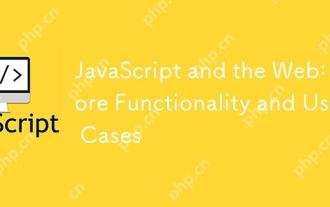
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
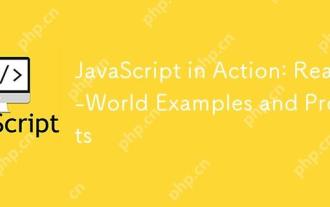
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
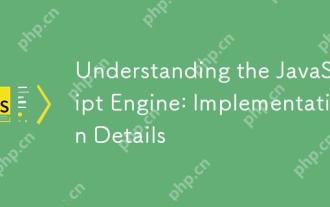
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
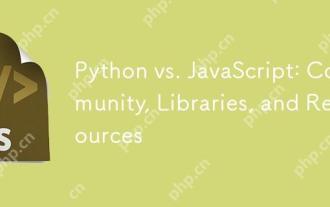
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
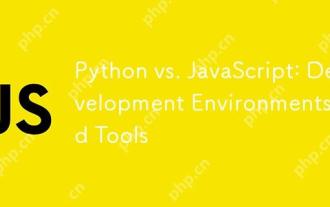
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
