掌握JavaScript中的数组方法:map、filter和reduce
JavaScript 中的数组方法:map、filter 和 reduce
JavaScript 提供了强大的数组方法来简化数组操作。其中,map、filter、reduce是每个开发者都应该了解的三个必备的高阶函数。
1.地图方法
map 方法通过使用回调函数转换现有数组的每个元素来创建一个新数组。
语法
array.map(callback(currentValue[, index[, array]])[, thisArg]);
示例:变换元素
const numbers = [1, 2, 3, 4]; const squared = numbers.map(function(number) { return number * number; }); console.log(squared); // Output: [1, 4, 9, 16]
用例
- 对数组应用转换(例如,转换单位、格式化数据)。
2.过滤方法
filter 方法创建一个新数组,仅包含通过所提供的回调函数实现的测试的元素。
语法
array.filter(callback(element[, index[, array]])[, thisArg]);
示例:过滤元素
const numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter(function(number) { return number % 2 === 0; }); console.log(evenNumbers); // Output: [2, 4]
用例
- 根据特定条件过滤数组(例如,过滤空值、查找活跃用户)。
3.减少方法
reduce 方法将函数应用于累加器和数组的每个元素(从左到右),将其减少为单个值。
语法
array.reduce(callback(accumulator, currentValue[, index[, array]])[, initialValue]);
示例:对数组元素求和
const numbers = [1, 2, 3, 4]; const sum = numbers.reduce(function(accumulator, currentValue) { return accumulator + currentValue; }, 0); console.log(sum); // Output: 10
用例
- 计算总计(例如总和、平均值)。
- 展平嵌套数组。
4.结合map、filter和reduce
这些方法可以组合起来执行复杂的操作。
示例:偶数平方和
const numbers = [1, 2, 3, 4, 5]; const total = numbers .filter(function(number) { return number % 2 === 0; // Keep even numbers }) .map(function(number) { return number * number; // Square the numbers }) .reduce(function(accumulator, currentValue) { return accumulator + currentValue; // Sum the squares }, 0); console.log(total); // Output: 20
5.主要差异
Method | Purpose | Return Value |
---|---|---|
map | Transforms each element | A new array of the same length |
filter | Filters elements | A new array with fewer or equal items |
reduce | Reduces array to a single value | A single accumulated result |
6.使用这些方法的好处
- 可读性:更干净、更具声明性的代码。
- 可重用性:模块化数组上的操作。
- 性能:通过优化的方法简化常用操作。
7.总结
- map 通过对每个元素应用函数来转换数组。
- 过滤器根据条件选择元素。
- reduce 将数组元素聚合为单个值。
- 这些方法促进函数式编程并简化数组处理。
掌握map、filter和reduce将提升你的JavaScript技能,让你的代码更干净、更高效。
嗨,我是 Abhay Singh Kathayat!
我是一名全栈开发人员,拥有前端和后端技术方面的专业知识。我使用各种编程语言和框架来构建高效、可扩展且用户友好的应用程序。
请随时通过我的商务电子邮件与我联系:kaashshorts28@gmail.com。
以上是掌握JavaScript中的数组方法:map、filter和reduce的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
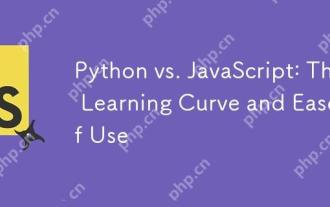
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
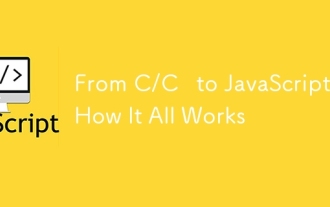
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
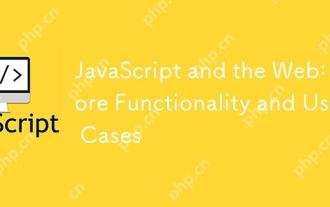
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
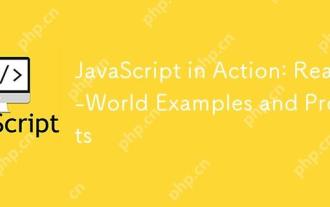
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
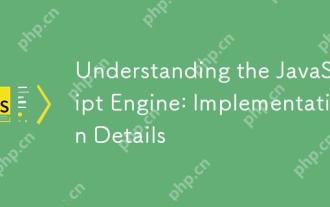
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
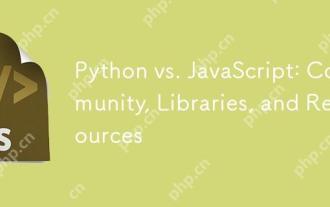
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
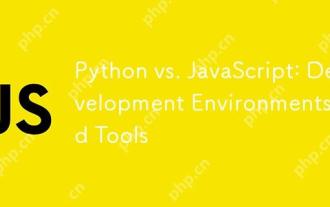
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
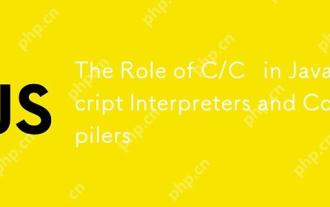
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
