Java 的'instanceof”运算符如何在运行时确定对象类型?
探索 Java 中的 instanceof 运算符
instanceof 运算符是 Java 类型系统的一个组成部分,允许您确定运行时类型一个对象并做出相应的响应。
使用instanceof时,您可以将对象引用与类型进行比较。语法遵循以下模式:
expression instanceof Type
比较的结果是一个布尔值,指示该对象是否是指定类型的实例。例如,给定以下代码:
if (source instanceof Button) { //... } else { //... }
如果 source 是 Button 类的实例,则 if 语句的计算结果将为 true,并且块中的代码将执行。如果 source 不是 Button,则将执行 else 语句。
为了理解这个概念,让我们考虑一个简化的类层次结构:
interface Domestic {} class Animal {} class Dog extends Animal implements Domestic {}
如果创建一个 Dog 对象并进行比较它使用instanceof:
Dog dog = new Dog(); System.out.println(dog instanceof Domestic); // true System.out.println(dog instanceof Animal); // true System.out.println(dog instanceof Dog); // true
结果是有意义的,因为Dog是Domestic和Animal的子类型。它还扩展了 Dog 类本身。但是,如果您尝试将其与不同的子类型进行比较:
System.out.println(dog instanceof Cat); // compilation error
这将导致编译错误,因为 Dog 不是 Cat 的子类型。
instanceof 运算符特别有用用于在处理多态行为时确定对象的运行时类型。考虑这样一个场景,您有多个从公共基类继承的类型:
class Shape {} class Circle extends Shape {} class Square extends Shape {}
在接受 Shape 对象作为参数的方法中,您可以使用 instanceof 来区分特定类型:
public void drawShape(Shape shape) { if (shape instanceof Circle) { // Draw circle } else if (shape instanceof Square) { // Draw square } else { // Handle other shapes } }
通过使用instanceof,您可以在运行时响应不同类型的对象,使您的代码更加灵活和适应性强。
以上是Java 的'instanceof”运算符如何在运行时确定对象类型?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
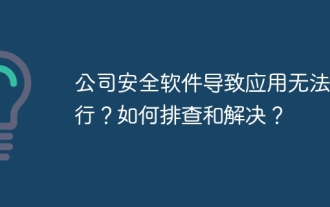
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
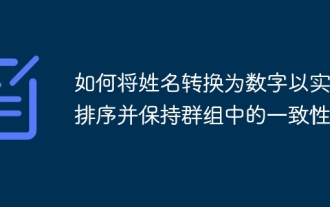
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
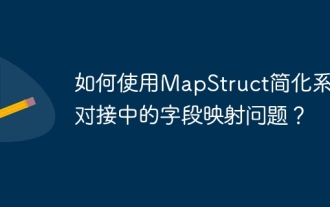
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
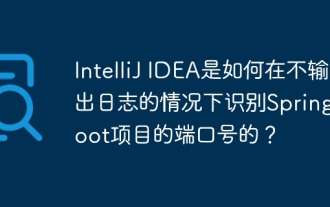
在使用IntelliJIDEAUltimate版本启动Spring...
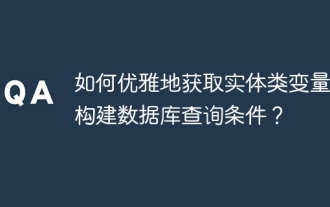
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
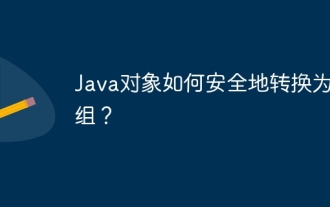
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
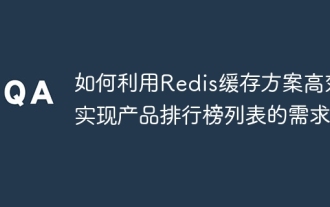
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
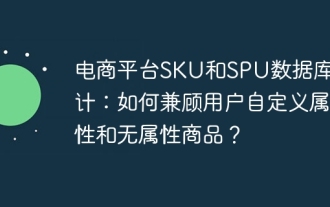
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
