


为什么在 Swing 中添加并关注 JTextField 而不是双缓冲动画 JPanel 会导致在使用 Java 1.6 的 Mac OS X 10.5 上出现闪烁问题?
JPanel 上活动绘图之上的 JTextFields,线程问题
Swing 可用于在顶部创建适当的多缓冲渲染环境其中可以添加 Swing 用户界面元素。在本例中,我们在背景上绘制了一个动画红色矩形。背景不需要每帧都更新,因此我们将其渲染到 BufferedImage 上,并仅重绘清除矩形先前位置所需的部分。
到目前为止一切顺利;动画流畅,CPU占用率低,无闪烁。然后我们向 Jpanel 添加一个 JTextField(通过单击屏幕上的任意位置),并通过单击文本框内部来聚焦它。现在,每次光标闪烁时,清除矩形的先前位置都会失败,请参见下图。
我们很好奇是否有人知道为什么会发生这种情况(Swing 不是线程安全的?正在绘制的图像异步?)以及在什么方向寻找可能的解决方案。
这是在 Mac OS 10.5、Java 1.6 上。
NewTest 扩展J面板;但是因为每次调用paintComponent()时你并没有绘制每个像素,所以你需要调用超类的方法并删除旧的绘图:
@Override protected void paintComponent(Graphics g) { super.paintComponent(g); int width = this.getWidth(); int height = this.getHeight(); g.setColor(Color.black); g.fillRect(0, 0, width, height); ... }
附录:正如你所注意到的,设置背景构造函数中的 color 不需要在 PaintComponent() 中填充面板,而 super.paintComponent() 允许文本字段正确运行。正如您所观察到的,提议的解决方法很脆弱。相反,应简化代码并根据需要进行优化。例如,您可能不需要复杂的插图、额外的缓冲区和组件侦听器。
附录 2:请注意 super.paintComponent() 调用 UI 委托的 update() 方法,“该方法填充指定的组件”及其背景颜色(如果其不透明属性为真)。”您可以使用 setOpaque(false) 来排除这种情况。
代码的进一步改进版本,具有一些附加功能和优化:
import java.awt.Color; import java.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.GraphicsConfiguration; import java.awt.GraphicsEnvironment; import java.awt.Rectangle; import java.awt.Transparency; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.ComponentAdapter; import java.awt.event.ComponentEvent; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.awt.image.BufferedImage; import java.util.Random; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JTextField; import javax.swing.Timer; /** @see http://stackoverflow.com/questions/3256941 */ public class AnimationTest extends JPanel implements ActionListener { private static final int WIDE = 640; private static final int HIGH = 480; private static final int RADIUS = 25; private static final int FRAMES = 24; private final Timer timer = new Timer(20, this); private final Rectangle rect = new Rectangle(); private BufferedImage background; private int index; private long totalTime; private long averageTime; private int frameCount; public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { new AnimationTest().create(); } }); } private void create() { JFrame f = new JFrame("AnimationTest"); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(this); f.pack(); f.setLocationRelativeTo(null); f.setVisible(true); timer.start(); } public AnimationTest() { super(true); this.setOpaque(false); this.setPreferredSize(new Dimension(WIDE, HIGH)); this.addMouseListener(new MouseHandler()); this.addComponentListener(new ComponentHandler()); } @Override protected void paintComponent(Graphics g) { long start = System.nanoTime(); super.paintComponent(g); int w = this.getWidth(); int h = this.getHeight(); g.drawImage(background, 0, 0, this); double theta = 2 * Math.PI * index++ / 64; g.setColor(Color.blue); rect.setRect( (int) (Math.sin(theta) * w / 3 + w / 2 - RADIUS), (int) (Math.cos(theta) * h / 3 + h / 2 - RADIUS), 2 * RADIUS, 2 * RADIUS); g.fillOval(rect.x, rect.y, rect.width, rect.height); g.setColor(Color.white); if (frameCount == FRAMES) { averageTime = totalTime / FRAMES; totalTime = 0; frameCount = 0; } else { totalTime += System.nanoTime() - start; frameCount++; } String s = String.format("%1.3f", averageTime / 1000000d); g.drawString(s, 5, 16); } @Override public void actionPerformed(ActionEvent e) { this.repaint(); } private class MouseHandler extends MouseAdapter { @Override public void mousePressed(MouseEvent e) { super.mousePressed(e); JTextField field = new JTextField("test"); Dimension d = field.getPreferredSize(); field.setBounds(e.getX(), e.getY(), d.width, d.height); add(field); } } private class ComponentHandler extends ComponentAdapter { private final GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment(); private final GraphicsConfiguration gc = ge.getDefaultScreenDevice().getDefaultConfiguration(); private final Random r = new Random(); @Override public void componentResized(ComponentEvent e) { super.componentResized(e); int w = getWidth(); int h = getHeight(); background = gc.createCompatibleImage(w, h, Transparency.OPAQUE); Graphics2D g = background.createGraphics(); g.clearRect(0, 0, w, h); g.setColor(Color.green.darker()); for (int i = 0; i < 128; i++) { g.drawLine(w / 2, h / 2, r.nextInt(w), r.nextInt(h)); } g.dispose(); System.out.println("Resized to " + w + " x " + h); } } }
以上是为什么在 Swing 中添加并关注 JTextField 而不是双缓冲动画 JPanel 会导致在使用 Java 1.6 的 Mac OS X 10.5 上出现闪烁问题?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
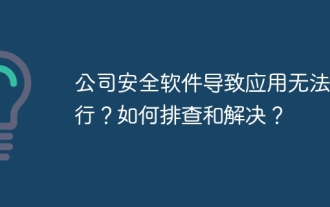
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
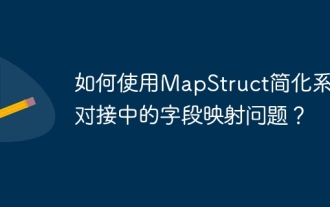
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
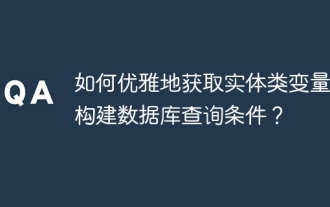
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
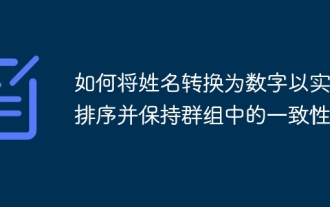
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
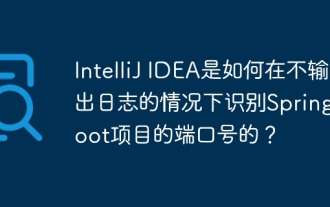
在使用IntelliJIDEAUltimate版本启动Spring...
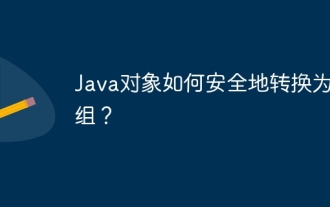
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
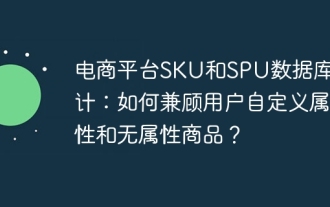
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
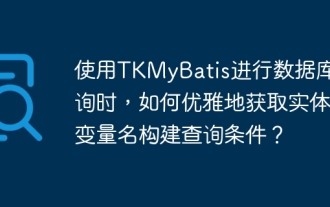
在使用TKMyBatis进行数据库查询时,如何优雅地获取实体类变量名以构建查询条件,是一个常见的难题。本文将针...
