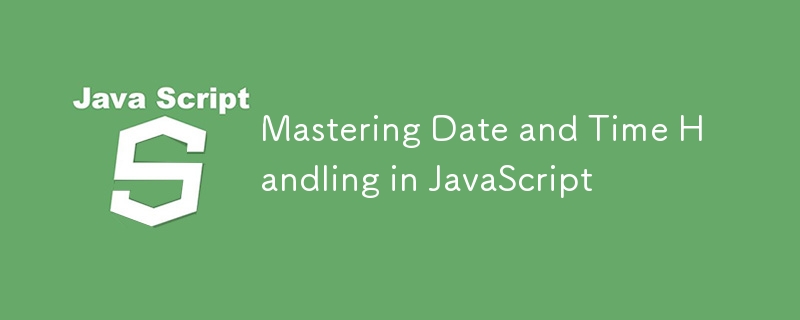
JavaScript 中的日期和时间处理
JavaScript 提供了 Date 对象来处理日期和时间。它用途广泛,提供各种方法来操作、格式化和计算日期和时间值。
1.创建日期对象
Date 对象可以通过不同的方式创建。
A.当前日期和时间
1 2 | const now = new Date ();
console.log(now);
|
登录后复制
登录后复制
B.具体日期和时间
1 2 | const specificDate = new Date ( "2023-12-31T23:59:59" );
console.log(specificDate);
|
登录后复制
登录后复制
C.使用年、月、日等
1 2 | const date = new Date (2023, 11, 31, 23, 59, 59);
console.log( date );
|
登录后复制
登录后复制
D.时间戳
Date 对象可以使用时间戳(自 1970 年 1 月 1 日起的毫秒数,UTC)来初始化。
1 2 | const timestamp = new Date (0);
console.log(timestamp);
|
登录后复制
登录后复制
2.获取日期和时间组件
Date 对象提供了提取日期特定部分的方法。
-
年份:getFullYear()
-
月份:getMonth()(0-11,其中0是一月)
-
一月中的某一天:getDate()
-
星期几:getDay()(0-6,其中 0 是星期日)
-
时间:getHours()
-
分钟:getMinutes()
-
秒:getSeconds()
-
毫秒: getMilliseconds()
示例:
1 2 3 4 5 6 | const now = new Date ();
console.log(now.getFullYear());
console.log(now.getMonth());
console.log(now. getDate ());
console.log(now.getDay());
console.log(now.getHours());
|
登录后复制
3.修改日期和时间
您可以使用 setter 方法更改日期的特定组成部分。
-
设置年份: setFullYear(year)
-
设置月份: setMonth(月)
-
设置日期: setDate(day)
-
设置时间: setHours(小时)
-
设置分钟: setMinutes(分钟)
示例:
1 2 3 4 5 | const date = new Date ();
date .setFullYear(2025);
date .setMonth(11);
date .setDate(25);
console.log( date );
|
登录后复制
4.设置日期和时间的格式
A. toISOString()
返回 ISO 8601 格式的日期。
1 2 | const now = new Date ();
console.log(now.toISOString());
|
登录后复制
B. toLocaleString()
以特定于区域设置的格式返回日期和时间。
1 2 3 | const now = new Date ();
console.log(now.toLocaleString( "en-US" ));
console.log(now.toLocaleString( "de-DE" ));
|
登录后复制
C. toDateString() 和 toTimeString()
-
toDateString():仅返回日期部分。
-
toTimeString():仅返回时间部分。
1 2 3 | const now = new Date ();
console.log(now.toDateString());
console.log(now.toTimeString());
|
登录后复制
5.计算日期差异
您可以通过将日期转换为时间戳来计算差异。
示例:
1 2 3 4 | const date1 = new Date ( "2023-12-31" );
const date2 = new Date ( "2024-01-01" );
const difference = date2 - date1;
console.log(difference / (1000 * 60 * 60 * 24));
|
登录后复制
6.比较日期
使用比较运算符来比较日期。
示例:
1 2 | const now = new Date ();
console.log(now);
|
登录后复制
登录后复制
7.使用时区
A. UTC 方法
-
getUTCFullYear()、getUTCMonth()、getUTCDate() 等返回 UTC 格式的组件。
1 2 | const specificDate = new Date ( "2023-12-31T23:59:59" );
console.log(specificDate);
|
登录后复制
登录后复制
B.转换为时区
使用 moment.js 或 date-fns 等库进行高级时区处理。
8.生成时间戳
A.当前时间戳
Date.now() 方法返回当前时间戳(以毫秒为单位)。
1 2 | const date = new Date (2023, 11, 31, 23, 59, 59);
console.log( date );
|
登录后复制
登录后复制
B.将日期转换为时间戳
使用 .getTime() 方法。
1 2 | const timestamp = new Date (0);
console.log(timestamp);
|
登录后复制
登录后复制
9.总结
- Date 对象对于处理日期和时间非常强大。
- 使用 getter 和 setter 方法提取或修改日期组件。
- toISOString() 和 toLocaleString() 等格式选项简化了日期表示。
- 对于高级操作,请考虑使用第三方库,例如 moment.js 或 date-fns.
掌握 JavaScript 中的日期和时间处理对于涉及调度、时间戳和本地化的应用程序至关重要。
嗨,我是 Abhay Singh Kathayat!
我是一名全栈开发人员,拥有前端和后端技术方面的专业知识。我使用各种编程语言和框架来构建高效、可扩展且用户友好的应用程序。
请随时通过我的商务电子邮件与我联系:kaashshorts28@gmail.com。
以上是掌握 JavaScript 中的日期和时间处理的详细内容。更多信息请关注PHP中文网其他相关文章!