在 Python 请求库中使用 XML
什么是 XML? XML 是指可扩展标记语言,它需要存储结构化数据并对任何项目进行分组。在 XML 标记语言中,您可以创建具有任何名称的标签。最流行的 XML 示例 - 站点地图和 RSS 提要。
XML 文件示例:
<breakfast_menu> <food> <name>Belgian Waffles</name> <price>.95</price> <description>Two of our famous Belgian Waffles with plenty of real maple syrup</description> <calories>650</calories> </food> <food> <name>Strawberry Belgian Waffles</name> <price>.95</price> <description>Light Belgian waffles covered with strawberries and whipped cream</description> <calories>900</calories> </food> <food> <name>Berry-Berry Belgian Waffles</name> <price>.95</price> <description>Light Belgian waffles covered with an assortment of fresh berries and whipped cream</description> <calories>900</calories> </food> <food> <name>French Toast</name> <price>.50</price> <description>Thick slices made from our homemade sourdough bread</description> <calories>600</calories> </food> <food> <name>Homestyle Breakfast</name> <price>.95</price> <description>Two eggs, bacon or sausage, toast, and our ever-popular hash browns</description> <calories>950</calories> </food> </breakfast_menu>
在这个例子中,文件包含 breakfast_menu 全局标签,其中包含食物类别,每个食物类别包括名称、价格、描述和卡路里标签。
现在我们开始学习如何使用 XML 和 Python 请求库。首先我们需要准备好工作环境。
要创建新项目和虚拟环境,请安装 python3-virtualenv 包。它需要每个项目的分离要求。在 Debian/Ubuntu 中安装:
sudo apt install python3 python3-virtualenv -y
创建项目文件夹:
mkdir my_project cd my_project
使用 env 命名的文件夹创建 Python 虚拟环境:
python3 -m venv env
激活虚拟环境:
source env/bin/activate
安装 PIP 的依赖项:
pip3 install requests
让我们开始编写代码吧。
创建 main.py 文件并在下面插入代码:
import requests import xml.etree.ElementTree as ET request = requests.get('https://www.w3schools.com/xml/simple.xml') root = ET.fromstring(request.content) for item in root.iter('*'): print(item.tag)
此代码片段帮助我们找到所有内部标签。
此代码的输出:
(env) user@localhost:~/my_project$ python3 main.py breakfast_menu food name price description calories food name price description calories food name price description calories food name price description calories food name price description calories
现在我们正在编写从内部元素获取值的代码。打开 main.py 文件并将之前的代码替换为:
import requests import xml.etree.ElementTree as ET request = requests.get('https://www.w3schools.com/xml/simple.xml') root = ET.fromstring(request.content) for item in root.iterfind('food'): print(item.findtext('name')) print(item.findtext('price')) print(item.findtext('description')) print(item.findtext('calories'))
我们收到了下一个结果:
(env) user@localhost:~/my_project$ python3 main.py Belgian Waffles .95 Two of our famous Belgian Waffles with plenty of real maple syrup 650 Strawberry Belgian Waffles .95 Light Belgian waffles covered with strawberries and whipped cream 900 Berry-Berry Belgian Waffles .95 Light Belgian waffles covered with an assortment of fresh berries and whipped cream 900 French Toast .50 Thick slices made from our homemade sourdough bread 600 Homestyle Breakfast .95 Two eggs, bacon or sausage, toast, and our ever-popular hash browns 950
最后一步,我们美化输出数据以使其更易于阅读:
import requests import xml.etree.ElementTree as ET request = requests.get('https://www.w3schools.com/xml/simple.xml') root = ET.fromstring(request.content) for item in root.iterfind('food'): print('Name: {}. Price: {}. Description: {}. Calories: {}'.format(item.findtext('name'), item.findtext('price'), item.findtext('description'), item.findtext('calories')))
此处输出:
(env) user@localhost:~/my_project$ python3 main.py Name: Belgian Waffles. Price: .95. Description: Two of our famous Belgian Waffles with plenty of real maple syrup. Calories: 650 Name: Strawberry Belgian Waffles. Price: .95. Description: Light Belgian waffles covered with strawberries and whipped cream. Calories: 900 Name: Berry-Berry Belgian Waffles. Price: .95. Description: Light Belgian waffles covered with an assortment of fresh berries and whipped cream. Calories: 900 Name: French Toast. Price: .50. Description: Thick slices made from our homemade sourdough bread. Calories: 600 Name: Homestyle Breakfast. Price: .95. Description: Two eggs, bacon or sausage, toast, and our ever-popular hash browns. Calories: 950
来源材料:
XML 文件示例取自 W3Schools。
我的帖子有帮助吗?您可以在 Patreon 上支持我。
以上是在 Python 请求库中使用 XML的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
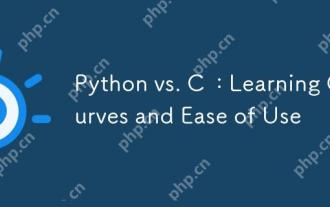
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
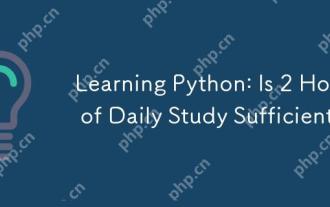
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
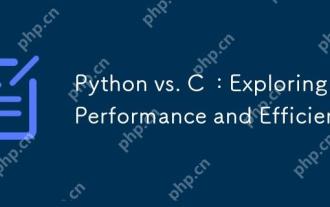
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
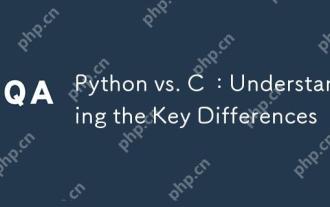
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
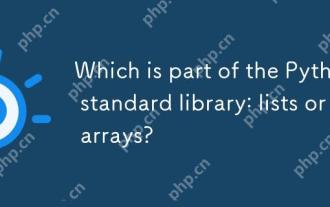
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
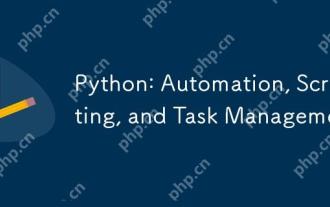
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
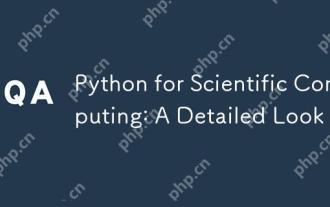
Python在科学计算中的应用包括数据分析、机器学习、数值模拟和可视化。1.Numpy提供高效的多维数组和数学函数。2.SciPy扩展Numpy功能,提供优化和线性代数工具。3.Pandas用于数据处理和分析。4.Matplotlib用于生成各种图表和可视化结果。
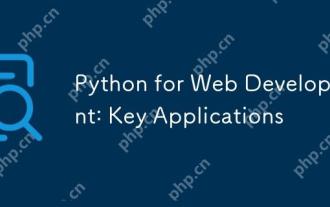
Python在Web开发中的关键应用包括使用Django和Flask框架、API开发、数据分析与可视化、机器学习与AI、以及性能优化。1.Django和Flask框架:Django适合快速开发复杂应用,Flask适用于小型或高度自定义项目。2.API开发:使用Flask或DjangoRESTFramework构建RESTfulAPI。3.数据分析与可视化:利用Python处理数据并通过Web界面展示。4.机器学习与AI:Python用于构建智能Web应用。5.性能优化:通过异步编程、缓存和代码优
