LeetCode 冥想:缺失的数字
我们先来描述这个问题:
给定一个数组 nums,其中包含 [0, n] 范围内的 n 个不同数字,返回 该范围内数组中唯一缺少的数字。
例如:
Input: nums = [3, 0, 1] Output: 2 Explanation: n = 3 since there are 3 numbers, so all numbers are in the range [0, 3]. 2 is the missing number in the range since it does not appear in nums.
或者:
Input: nums = [0, 1] Output: 2 Explanation: n = 2 since there are 2 numbers, so all numbers are in the range [0, 2]. 2 is the missing number in the range since it does not appear in nums.
或者:
Input: nums = [9, 6, 4, 2, 3, 5, 7, 0, 1] Output: 8 Explanation: n = 9 since there are 9 numbers, so all numbers are in the range [0, 9]. 8 is the missing number in the range since it does not appear in nums.
还指出所有nums的数字都是唯一。
解决这个问题的一个简单方法是获取范围的总和,然后减去给定数组的总和。剩下的就是缺失的数字。
可以使用reduce来对数字求和,如下所示:
function missingNumber(nums: number[]): number { return Array.from({ length: nums.length + 1 }, (_, idx) => idx).reduce((acc, item) => acc + item, 0) - nums.reduce((acc, item) => acc + item, 0); }
首先,我们创建一个数组,其值从 0 到 nums.length 1 并获取其总和,然后从中减去 nums 的总和。
但是,时间和空间复杂度将为 O(n) 使用此解决方案,我们为该范围创建一个数组。
我们可以使用位操作获得更(存储方面)高效的解决方案。
事实上,我们可以使用 XOR 运算来帮助我们实现这一点。
要记住,如果两个位都不同,则 XOR 结果为 1,即其中一个为 0,另一个为 1。
当我们将一个数字与其自身进行异或时,结果将是 0,因为所有位都是相同的。
例如,二进制的 3 是 11,当我们执行 11 ^ 11 时,结果是 0:
const n = 3; const result = n ^ n; // 0
换句话说,数字与自身的异或运算将得到 0。
如果我们将数组中的每个数字与索引进行异或,最终所有数字都会抵消(结果为 0),只留下缺失的数字。
你可能会认为并不是所有的数字都在它们的索引处,例如如果 nums 是 [3, 0, 1],很明显 3 甚至没有可以与之关联的“索引 3”!
为此,我们可以首先将结果初始化为 nums.length。现在,即使缺失的数字等于 nums.length,我们也会处理这种边缘情况。
let result = nums.length;
此外,XOR 是可交换和结合的,因此数字出现在哪个索引处并不重要(或者没有一个,如上面的示例) - 它们最终会抵消。
现在,通过 for 循环,我们可以使用按位异或赋值运算符进行异或:
for (let i = 0; i < nums.length; i++) { result ^= i ^ nums[i]; }
并且,最终的结果是缺失的数字。解决方案总体如下所示:
Input: nums = [3, 0, 1] Output: 2 Explanation: n = 3 since there are 3 numbers, so all numbers are in the range [0, 3]. 2 is the missing number in the range since it does not appear in nums.
时间和空间复杂度
时间复杂度又是 O(n) 当我们遍历数组中的每个数字时,但空间复杂度将为 O(1) 因为我们没有任何额外的存储需求,这些需求会随着输入大小的增加而增加。
接下来,我们来看看整个系列的最后一个问题,两个整数的和。在那之前,祝您编码愉快。
以上是LeetCode 冥想:缺失的数字的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
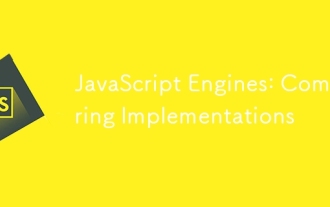
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
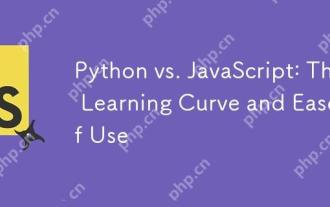
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
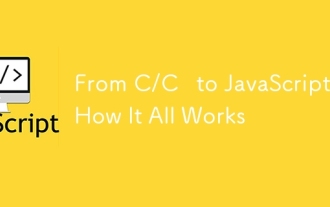
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
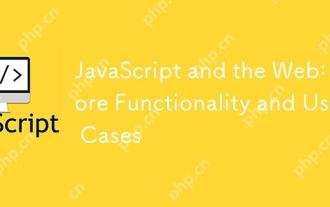
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
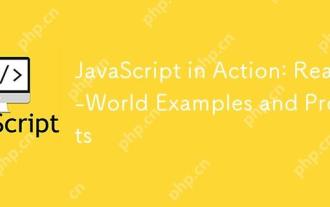
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
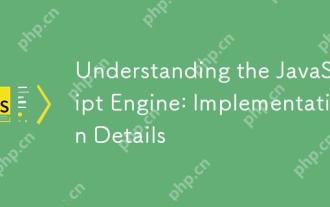
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
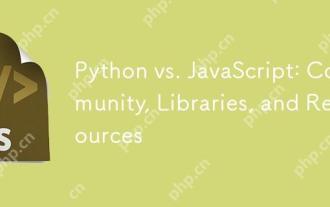
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
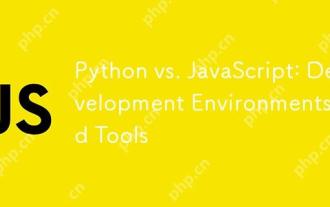
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
