通过更改源代码来破解 Python 函数
欢迎来到下一个 pikoTutorial!
通过手动修改函数的实现来更改函数的行为是显而易见的,但是我们可以在应用程序运行时以某种方式搞乱函数的实现吗?让我们将这个过程分为 3 个步骤:
- 在运行时获取函数的源代码
- 将带有源代码的字符串转换为可调用对象
- 调用函数之前修改其源代码
在运行时获取函数的源代码
我们先来学习一下如何获取函数的源码:
# Import inspect module import inspect # Define some callback function def function(): print('Do something') source_code = inspect.getsource(function) print(source_code)
输出:
def callback(): print('Do something')
将带有源代码的字符串转换为可调用对象
现在让我们看看如何将字符串中提供的任意 Python 代码转换为可调用的 Python 对象:
# Source code that we want to execute source_code = 'print("Hello from the inside of the string!")' # Wrap the source code into a function definition, so that it can be accessed by name function_name = 'print_hello' function_definition = f'def {function_name}():\n {source_code}' namespace = {} # Execute code with a function definition within the given namespace, so that the function definition is created exec(function_definition, namespace) # Retrieve function from the namespace and save to a callable variable print_hello = namespace[function_name] # Call the function print_hello()
输出:
Hello from the inside of the string!
调用函数之前修改其源代码
现在让我们实现一个函数,它将函数指针作为输入并返回一个带有修改后的源代码的可调用对象:
import inspect def get_hacked_function(function): # Get the source code of the given function original_function_source_code = inspect.getsource(function) # Append a new line to the function source code modified_function_source_code = f'{original_function_source_code} print("You didn\'t expect me here!")' # Call the function within the namespace namespace = {} exec(modified_function_source_code, namespace) # Parse function name by taking everything what's between "def " and "(" at the first line function_name = original_function_source_code.split('(')[0].split()[1] # Retrieve modified function modified_function = namespace[function_name] # Return modified function return modified_function
是时候测试一下了!
# This is the function passed as an input def original_function(): print("Hello") # Call our hacking function hacked_function = get_hacked_function(original_function) # Call the modified function hacked_function()
输出:
Hello You didn't expect me here!
初学者注意事项:请记住,此类实验主要用于教育目的。使用 exec() 函数会带来严重的安全问题,因此不建议在生产环境中使用它。如果您需要修改您无权访问其源代码的函数的行为,请考虑使用函数装饰器。在使用 exec() 之前始终保持谨慎并确保您完全理解安全隐患。
以上是通过更改源代码来破解 Python 函数的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
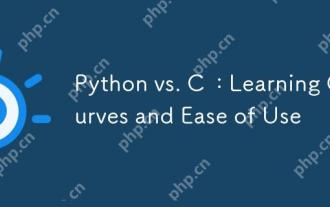
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
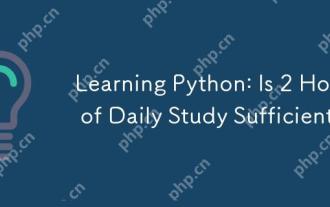
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
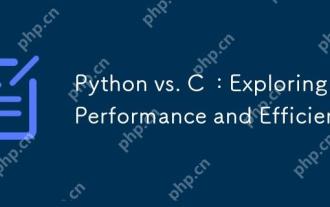
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
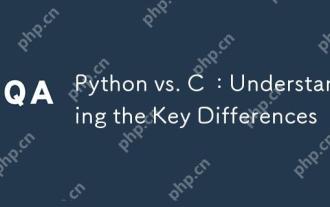
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
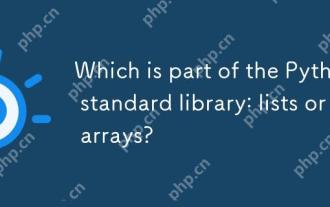
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
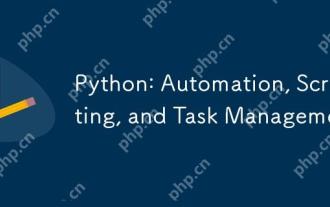
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
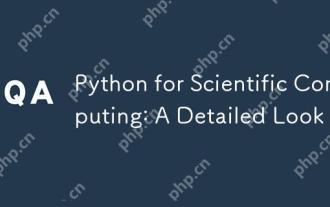
Python在科学计算中的应用包括数据分析、机器学习、数值模拟和可视化。1.Numpy提供高效的多维数组和数学函数。2.SciPy扩展Numpy功能,提供优化和线性代数工具。3.Pandas用于数据处理和分析。4.Matplotlib用于生成各种图表和可视化结果。
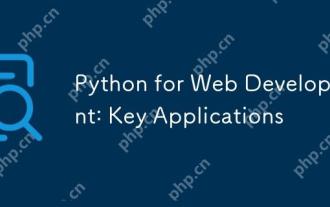
Python在Web开发中的关键应用包括使用Django和Flask框架、API开发、数据分析与可视化、机器学习与AI、以及性能优化。1.Django和Flask框架:Django适合快速开发复杂应用,Flask适用于小型或高度自定义项目。2.API开发:使用Flask或DjangoRESTFramework构建RESTfulAPI。3.数据分析与可视化:利用Python处理数据并通过Web界面展示。4.机器学习与AI:Python用于构建智能Web应用。5.性能优化:通过异步编程、缓存和代码优
