掌握 Kotlin 协程的综合指南
介绍
协程通过提高可读性和效率来简化异步编程。将线程视为高速公路上的单辆汽车,每辆汽车都占用空间和资源。相比之下,协程就像拼车 - 多个任务有效地共享资源。
协程的三大优势使其脱颖而出:
- 处理异步操作的简单性和可读性
- 与传统线程相比,高效的资源管理
- 通过结构化并发增强代码可维护性
设置协程
要开始在 Android 项目中使用协程,请将这些依赖项添加到您的 build.gradle 文件中:
dependencies { implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:1.7.1" implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:1.7.1" }
了解协程构建器
协程构建器是创建和启动协程的基础。让我们用实际例子来探讨每种类型:
启动
class WeatherService { fun updateWeather() { lifecycleScope.launch { // Simulating weather API call val weather = fetchWeatherData() updateUI(weather) } } private suspend fun fetchWeatherData(): Weather { delay(1000) // Simulate network delay return Weather(temperature = 25, condition = "Sunny") } }
异步
class StockPortfolio { suspend fun fetchPortfolioValue() { val stocksDeferred = async { fetchStockPrices() } val cryptoDeferred = async { fetchCryptoPrices() } // Wait for both results val totalValue = stocksDeferred.await() + cryptoDeferred.await() println("Portfolio value: $totalValue") } }
协程作用域和上下文
理解范围和上下文对于正确的协程管理至关重要。让我们看看不同的范围类型:
生命周期范围
class NewsActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) lifecycleScope.launch { val news = newsRepository.fetchLatestNews() newsAdapter.submitList(news) } } }
ViewModelScope
class UserViewModel : ViewModel() { private val _userData = MutableLiveData<User>() fun loadUserData() { viewModelScope.launch { try { val user = userRepository.fetchUserDetails() _userData.value = user } catch (e: Exception) { // Handle error } } } }
与调度员合作
调度程序确定运行哪个线程协程。以下是如何有效地使用不同的调度程序:
class ImageProcessor { fun processImage(bitmap: Bitmap) { lifecycleScope.launch(Dispatchers.Default) { // CPU-intensive image processing val processed = applyFilters(bitmap) withContext(Dispatchers.Main) { // Update UI with processed image imageView.setImageBitmap(processed) } } } suspend fun downloadImage(url: String) { withContext(Dispatchers.IO) { // Network operation to download image val response = imageApi.fetchImage(url) saveToDatabase(response) } }
错误处理和异常管理
正确的错误处理在协程中至关重要。以下是有效实施的方法:
class DataManager { private val exceptionHandler = CoroutineExceptionHandler { _, exception -> println("Caught $exception") } fun fetchData() { lifecycleScope.launch(exceptionHandler) { try { val result = riskyOperation() processResult(result) } catch (e: NetworkException) { showError("Network error occurred") } catch (e: DatabaseException) { showError("Database error occurred") } } } }
流和状态流
Flow 非常适合处理数据流,而 StateFlow 非常适合管理 UI 状态:
class SearchViewModel : ViewModel() { private val _searchResults = MutableStateFlow<List<SearchResult>>(emptyList()) val searchResults: StateFlow<List<SearchResult>> = _searchResults.asStateFlow() fun search(query: String) { viewModelScope.launch { searchRepository.getSearchResults(query) .flowOn(Dispatchers.IO) .catch { e -> // Handle errors } .collect { results -> _searchResults.value = results } } } }
结构化并发
结构化并发有助于有效管理相关协程:
class OrderProcessor { suspend fun processOrder(orderId: String) = coroutineScope { val orderDeferred = async { fetchOrderDetails(orderId) } val inventoryDeferred = async { checkInventory(orderId) } val paymentDeferred = async { processPayment(orderId) } try { val order = orderDeferred.await() val inventory = inventoryDeferred.await() val payment = paymentDeferred.await() finalizeOrder(order, inventory, payment) } catch (e: Exception) { // If any operation fails, all others are automatically cancelled throw OrderProcessingException("Failed to process order", e) } } }
结论
Kotlin 协程提供了一种强大而直观的方法来处理 Android 开发中的异步操作。通过理解这些核心概念和模式,您可以编写更高效、可维护且健壮的应用程序。请记住始终考虑适合您的特定用例的范围、调度程序和错误处理策略。
掌握协程的关键是练习 - 开始在您的项目中实现它们,尝试不同的模式,并随着您理解的增长逐渐构建更复杂的实现。
最初写在这里
以上是掌握 Kotlin 协程的综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
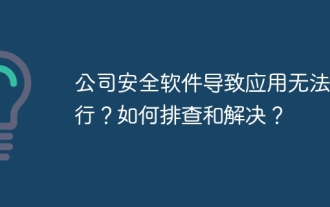
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
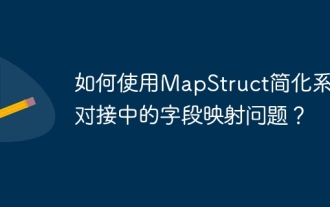
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
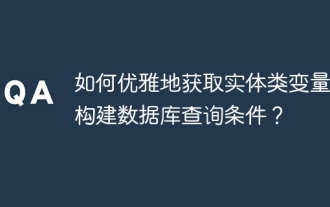
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
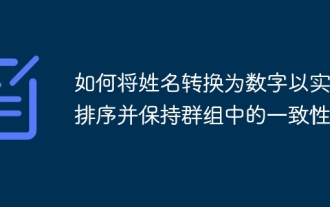
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
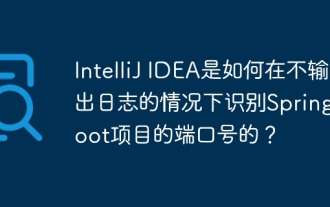
在使用IntelliJIDEAUltimate版本启动Spring...
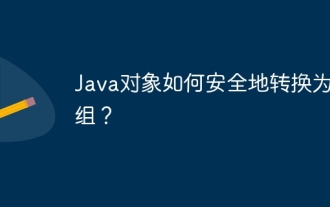
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
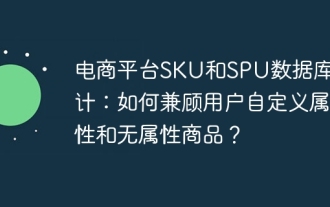
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
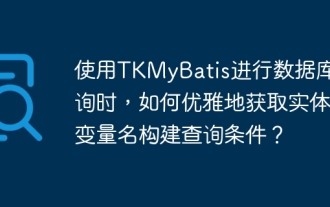
在使用TKMyBatis进行数据库查询时,如何优雅地获取实体类变量名以构建查询条件,是一个常见的难题。本文将针...
