如何以编程方式确定在 Windows 中打开文件类型的默认应用程序?
确定在 Windows 上打开文件类型的默认应用程序
使用适当的默认应用程序打开文件是许多应用程序的常见任务。要使用 C# 在 .NET Framework 2.0 中实现此目的,可以利用 System.Diagnostics.Process.Start 方法。但是,此方法需要确切的文件扩展名来确定默认应用程序。
要在所需的默认应用程序中打开没有特定扩展名的文件,您需要检索与该文件类型关联的应用程序。虽然使用注册表来确定这种关联是一种不可靠的方法,但 Win32 API 提供了一种更可靠的方法。
Shlwapi.dll 库中的 AssocQueryString 函数允许您查询特定文件类型的关联。使用此函数,您可以确定文件类型的默认命令、可执行文件或其他相关信息。
示例用法演示了如何利用 AssocQueryString 获取与文件扩展名关联的命令:
using System; using System.Runtime.InteropServices; namespace FileAssociation { class Program { [DllImport("Shlwapi.dll", CharSet = CharSet.Unicode)] public static extern uint AssocQueryString( AssocF flags, AssocStr str, string pszAssoc, string pszExtra, [Out] StringBuilder pszOut, ref uint pcchOut); public enum AssocF { None = 0x00000000, Init_NoRemapCLSID = 0x00000001, Init_ByExeName = 0x00000002, Open_ByExeName = 0x00000002, Init_DefaultToStar = 0x00000004, Init_DefaultToFolder = 0x00000008, NoUserSettings = 0x00000010, NoTruncate = 0x00000020, Verify = 0x00000040, RemapRunDll = 0x00000080, NoFixUps = 0x00000100, IgnoreBaseClass = 0x00000200, Init_IgnoreUnknown = 0x00000400, Init_Fixed_ProgId = 0x00000800, Is_Protocol = 0x00001000, Init_For_File = 0x00002000 } public enum AssocStr { Command = 1, Executable, FriendlyDocName, FriendlyAppName, NoOpen, ShellNewValue, DDECommand, DDEIfExec, DDEApplication, DDETopic, InfoTip, QuickTip, TileInfo, ContentType, DefaultIcon, ShellExtension, DropTarget, DelegateExecute, Supported_Uri_Protocols, ProgID, AppID, AppPublisher, AppIconReference, Max } public static string AssocQueryString(AssocStr association, string extension) { const int S_OK = 0x00000000; const int S_FALSE = 0x00000001; uint length = 0; uint ret = AssocQueryString(AssocF.None, association, extension, null, null, ref length); if (ret != S_FALSE) { throw new InvalidOperationException("Could not determine associated string"); } var sb = new StringBuilder((int)length); // (length - 1) will probably work too as the marshaller adds null termination ret = AssocQueryString(AssocF.None, association, extension, null, sb, ref length); if (ret != S_OK) { throw new InvalidOperationException("Could not determine associated string"); } return sb.ToString(); } public static void Main(string[] args) { string extension = ".txt"; string command = AssocQueryString(AssocStr.Command, extension); System.Diagnostics.Process.Start(command, "test.txt"); } } }
以上是如何以编程方式确定在 Windows 中打开文件类型的默认应用程序?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
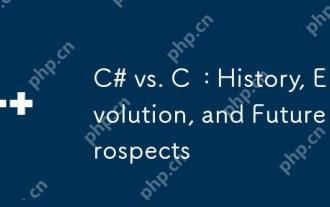
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
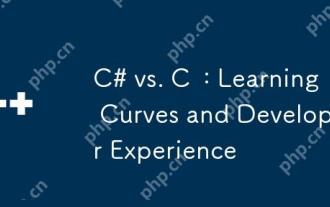
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
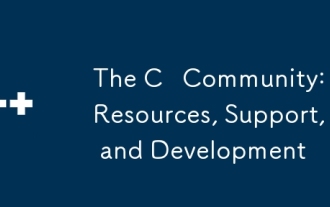
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
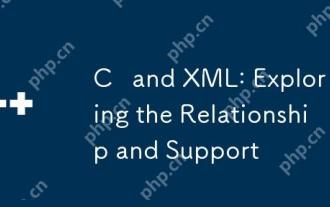
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
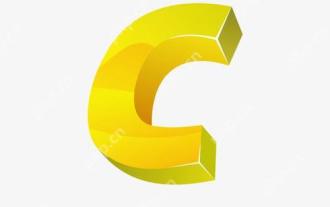
静态分析在C 中的应用主要包括发现内存管理问题、检查代码逻辑错误和提高代码安全性。1)静态分析可以识别内存泄漏、双重释放和未初始化指针等问题。2)它能检测未使用变量、死代码和逻辑矛盾。3)静态分析工具如Coverity能发现缓冲区溢出、整数溢出和不安全API调用,提升代码安全性。
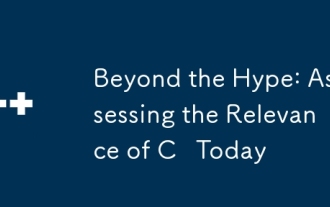
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
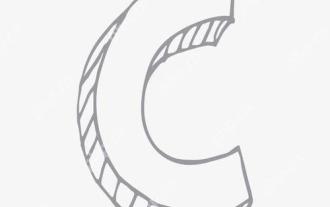
使用C 中的chrono库可以让你更加精确地控制时间和时间间隔,让我们来探讨一下这个库的魅力所在吧。C 的chrono库是标准库的一部分,它提供了一种现代化的方式来处理时间和时间间隔。对于那些曾经饱受time.h和ctime折磨的程序员来说,chrono无疑是一个福音。它不仅提高了代码的可读性和可维护性,还提供了更高的精度和灵活性。让我们从基础开始,chrono库主要包括以下几个关键组件:std::chrono::system_clock:表示系统时钟,用于获取当前时间。std::chron
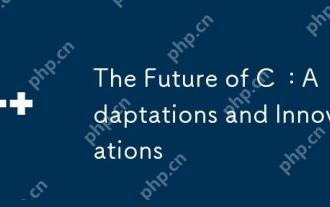
C 的未来将专注于并行计算、安全性、模块化和AI/机器学习领域:1)并行计算将通过协程等特性得到增强;2)安全性将通过更严格的类型检查和内存管理机制提升;3)模块化将简化代码组织和编译;4)AI和机器学习将促使C 适应新需求,如数值计算和GPU编程支持。
