原生 JavaScript API 简介:MutationObserver、IntersectionObserver 和 History API
现代 Web 应用程序需要响应能力、效率和动态交互性。原生 JavaScript API(例如 MutationObserver、IntersectionObserver 和 History API)使开发人员能够直接应对这些挑战,而无需外部库。让我们详细探索这些 API,了解它们的用例,并学习如何有效地利用它们的功能。
变异观察者
概述:
MutationObserver 接口监视 DOM 树中的更改,替换现已弃用的 Mutation Events。它可以检测节点何时添加、删除或修改,使其成为动态应用程序的必备工具。
主要特点:
- 监视 DOM 树的更改。
- 检测对属性、子节点和文本内容的修改。
- 异步操作,确保对性能的影响最小。
问。 MutationObserver 是如何工作的?
MutationObserver 实例是使用回调函数创建的,每当 DOM 中发生指定的更改时就会触发该回调函数。
MutationObserver 中的选项
子树:观察目标节点及其所有后代。
childList:监视子节点的添加或删除。
属性:跟踪目标节点属性的更改。
attributeFilter:限制对指定属性的监控。
attributeOldValue:捕获属性更改之前的先前值。
characterData:观察节点文本内容的变化。
characterDataOldValue:捕获文本内容修改前的先前值。
HTML 语法
<div> <p><strong>JS Syntax</strong><br> </p> <pre class="brush:php;toolbar:false"> const target = document.querySelector('#something') const observer = new MutationObserver(function(mutations){ mutations.forEach(function(mutation){ console.log('Mutation detected:', mutation) }) }) const config = {attributes:true, childList:true, characterData:true, subtree:true} observer.observe(target,config) //observer.disconnect() - to stop observing
用例:
- 动态更新 UI 元素。
- 实现 DOM 更改的自定义行为。
- 监控第三方库的修改。
路口观察者
概述:
IntersectionObserver 是一个异步观察目标元素相对于根容器或视口的可见性变化的接口。它通常用于延迟加载、无限滚动和分析。
主要特点:
- 有效跟踪元素可见性。
- 减少对滚动事件侦听器的依赖。
- 提供对阈值的细粒度控制。
问。路口观察器如何工作?
Intersection Observer API 会在发生以下任一情况时触发回调:
目标元素与设备的视口或指定的根元素相交。
观察者第一次开始观察目标元素。
路口观察器中的选项
root:用作检查可见性的视口的元素。如果未指定,则默认为浏览器的视口。
rootMargin:根周围的边距,指定为字符串(例如“10px 20px”)。扩大或缩小可观察区域。
阈值:0 到 1 之间的值(或值数组),指示触发回调所需的可见性百分比。
问。交集是如何计算的?
Intersection Observer API 使用矩形来计算交叉区域:
不规则形状的元素被视为适合完全包围它们的最小矩形。
对于部分可见的元素,使用包含所有可见部分的最小矩形。无论元素形状或可见性如何,这都可以确保测量的一致性。
基本语法
<div> <p><strong>JS Syntax</strong><br> </p> <pre class="brush:php;toolbar:false"> const target = document.querySelector('#something') const observer = new MutationObserver(function(mutations){ mutations.forEach(function(mutation){ console.log('Mutation detected:', mutation) }) }) const config = {attributes:true, childList:true, characterData:true, subtree:true} observer.observe(target,config) //observer.disconnect() - to stop observing
用例:
- 延迟加载图像或视频。
- 实现无限滚动。
- 跟踪用户对特定元素的参与度。
高级功能:
- 多个阈值:使用阈值数组观察部分可见性。
- 根边距:扩展视口的边界以进行早期检测。
历史API
概述:
History API 使 Web 应用程序能够操作浏览器的会话历史记录。它允许添加、替换或修改条目,而无需重新加载页面,这是单页应用程序 (SPA) 的基石。
主要特点:
- 使用pushState和replaceState管理历史堆栈。
- 使用 popstate 监听导航事件。
- 更新浏览器的地址栏,无需重新加载整页。
基本语法:
const observer = new IntersectionObserver((entries, observer) => { entries.forEach(entry => { if (entry.isIntersecting) { console.log('Element is visible in the viewport.') // Optionally stop observing observer.unobserve(entry.target) } }) }) // Target elements to observe const targetElement = document.querySelector('.lazy-load') // Start observing observer.observe(targetElement)
用例:
- 使用动态路由构建 SPA。
- 通过浏览器导航管理应用程序状态。
- 创建自定义导航体验。
- 重要提示:
- 确保旧版浏览器有适当的回退。
- 与 URL 参数结合以获得更好的 SEO。
组合这些 API
这些 API 可以协同工作来创建复杂的 Web 应用程序。例如:
- 使用MutationObserver来监控动态DOM变化。
- 实现 IntersectionObserver 来通过 DOM 更改添加 延迟加载内容。
- 利用历史API在应用程序内提供无缝导航。
示例用例:
当用户向下滚动(无限滚动)时,博客应用程序会动态加载帖子。它还会更新 URL 以反映当前帖子,而无需重新加载页面,从而确保更好的用户体验并改进 SEO。
<div> <p><strong>JS Syntax</strong><br> </p> <pre class="brush:php;toolbar:false"> const target = document.querySelector('#something') const observer = new MutationObserver(function(mutations){ mutations.forEach(function(mutation){ console.log('Mutation detected:', mutation) }) }) const config = {attributes:true, childList:true, characterData:true, subtree:true} observer.observe(target,config) //observer.disconnect() - to stop observing
结论
MutationObserver、IntersectionObserver 和 History API 为动态和交互式 Web 应用程序提供了强大的本机解决方案。通过了解它们的功能并有效地集成它们,开发人员可以构建高性能且功能丰富的应用程序,而无需严重依赖外部库。
以上是原生 JavaScript API 简介:MutationObserver、IntersectionObserver 和 History API的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
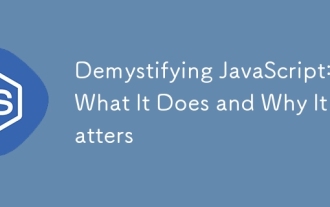
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
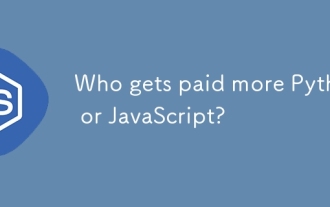
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
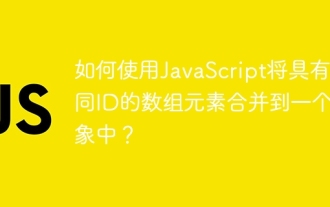
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
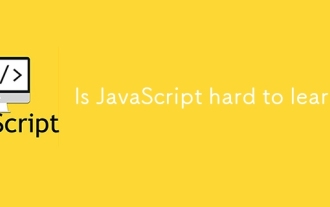
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
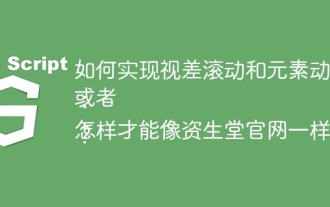
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
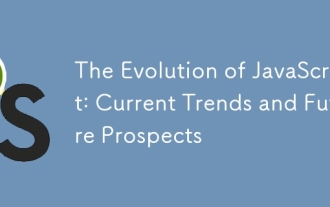
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
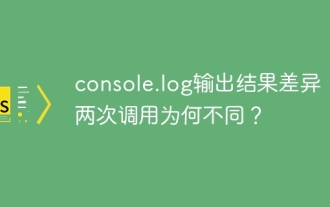
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
